Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial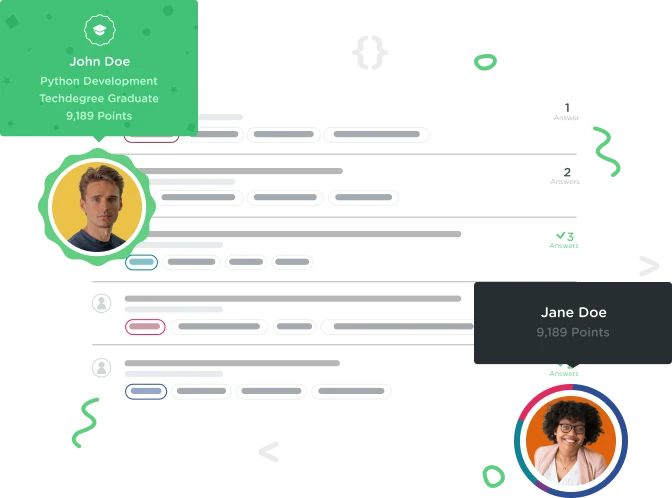
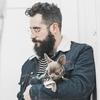
Alexander D
8,685 PointsReversing a word with pop()
Hello there,
I am stuck doing a simple exercise, I do not understand what I am going wrong. Take this very simple code:
var word = "racecar"
var rword = "";
var letters = ["r", "a", "c", "e", "c", "a", "r"];
for (var i=0; i < word.length; i ++) {
rword += letters.pop()
}
console.log(rword)
The program loops through an array and saves the letters in a variable. The program should return the inverted palindrome word "racecar".
However if I modify the code to the following I am getting only "race". Why is that?
for (var i=0; i < letters.length; i ++) {
rword += letters.pop()
}
console.log(rword)
letters.length is 7. word.length is 7 as well, so I should get the same result?
I noticed that while I loop through the second example, the array gets shorter and shorter because I am removing letters from the array. Therefore, I would make sense that the final result is incomplete. However I still have 3 items left when the loop finishes:
for (var i=0; i < letters.length; i ++) {
console.log(letters.length)
rword += letters.pop()
}
console.log(rword, letters.length)
Thanks!
3 Answers
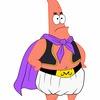
<noob />
17,062 PointsYour very close. the only thing you dont know is that You can't modify the array ur iterating on because your'e changing the index of the array evreytime you use the pop() function. run this code to see:
var copyLetters = ["r", "a", "c", "e", "c", "a", "r"];
var rword = "";
var index = 0;
for (let i=0; i < copyLetters.length; i++) {
const k = copyLetters.pop();
rword += k;
console.log(`${k} has been added and the index we are iterating on is index ${index} and the array looks like this: ${copyLetters}`);
index++;
}
console.log(rword);
in order to keep track of the indexing you will have to create a copy of the array that you're going to iterate on. 1 array for the iteration and the indexing 1 array the modifciation , such as "pop" out a letter evrey iteration.
var word = "racecar"
var rword = "";
var letters = ["r", "a", "c", "e", "c", "a", "r"];
var copyLetters = ["r", "a", "c", "e", "c", "a", "r"];
let index = 0;
for (let i=0; i < copyLetters.length; i++) {
const k = letters.pop();
rword += k;
console.log(`${k} has been added and the index is ${index}`);
index++;
}
console.log(rword);
as u see now, the word "racecar" has been reversed.
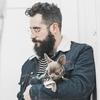
Alexander D
8,685 PointsScript Ninja Thanks for your answer, very clear!
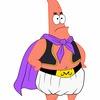
<noob />
17,062 Points:]