Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial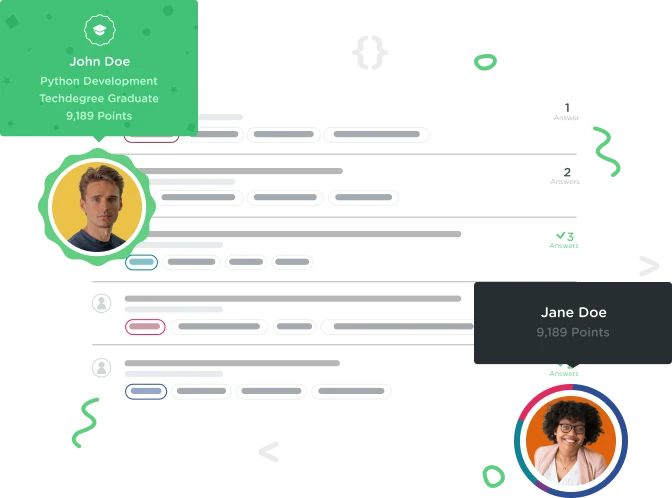

sondreg
5,515 PointsReview my code
Here's how I did the show spoiler program.
// Hide spoiler
$(".spoiler span").hide();
// Add a button
$(".spoiler").append("<button>Show Spoiler</>");
// When button is pressed
$(".spoiler button").click(function(){
// Get rid of button
$(".spoiler button").hide();
// Show Spoiler
$(".spoiler span").show();
});
2 Answers

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHi,
Your code looks good so far but could do with some improvement. When you click 'show spoiler' both spoilers are revealed. Ideally, only the spoiler that was clicked should be revealed.
Heres the code I used to do this:
// Hide spoiler
$(".spoiler span").hide();
// Add a button
$(".spoiler").append("<button>Show Spoiler</>");
// When button is pressed
$(".spoiler button").click(function(){
// Get rid of button
$(this).hide();
// Show Spoiler
$(this).prev().show();
});
Some explanation:
Instead of declaring $('.spoiler button') twice in our code, we can use $(this) which refers to the element that was clicked.
$('.spoiler button').hide() will select all buttons inside an element with the class spoiler (it will select both buttons the page). $(this).hide will only select the button that was clicked.
$(this).prev().show() will select the button that was clicked, then select it's preceding sibling (the span element), and then show the span element to the user. This can also be written as $(this).prev('span').show() for more clarity.
If you are unfamiliar with "this" I would recommend checking out the Understanding "this" workshop on Treehouse (requires pro account). Or just google "this javascript" and you'll find plenty of resources that will help explain it.
Hope this helps :)

Aaron Martone
3,290 PointsIf you find yourself making multiple references to the same element, it may be a good choice to create a variable. Each time you reference the same element, jQuery has to reparse the DOM to find it, ie. (I prefix my varnames with '$' when they are jQuery result sets). Also remember you can chain off of jQuery result sets.
var $spoilerButton = $('.spoiler button');
$spoilerButton.on('click', function spoilerButtonClickHandler() {
// ...
}).html('Hello');