Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial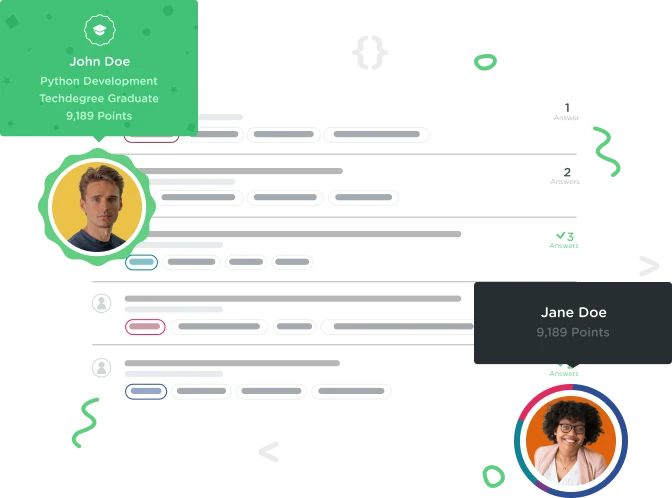

Siddharth Pande
9,046 PointsReview my code for the challenge by Guil
// I tried to remove the "Up" button from the first list item (not hide) even when there is a change in the
//placement of the buttons due to user activity.
// When a fifth list item (at index of 4) is added this code doesn't work.
//You are free to comment. Please make this code better in its functionality and help me learn more.
// There are many problems in the functionality like after removal of the button "Up" and "Down" the buttons next to them
// adopt their design pattern. Kindly help fix and let me know how you did it.
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const listUl = listDiv.querySelector('ul');
const lis = listUl.children;
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
var li;
//This function when called upon will add three button items to each of the list items
function addButtonsToList(li) {
var btnUp = document.createElement('button');
btnUp.className = "up";
btnUp.textContent = "Up";
li.appendChild(btnUp);
var btnDown = document.createElement('button');
btnDown.className = 'down';
btnDown.textContent = 'Down';
li.appendChild(btnDown);
var btnRemove = document.createElement('button');
btnRemove.className = "remove";
btnRemove.textContent = "Remove";
li.appendChild(btnRemove);
}
function removeButtonsFromList(li) {// function will be called upon after change in the first list item to remove the "Up" button
li.removeChild(li.firstElementChild);
}
function removeDownButton (li) { // this code removes "down" button from the last list item after there is a change in the buttons placement
li.removeChild(li.querySelector('button.down'));
}
function addDownButtonOnly2ndLastListItem (li) { // to add "down" button again after it gets removed form the second to last list item
var btnDown = document.createElement('button');
btnDown.className = 'down';
btnDown.textContent = 'Down';
li.insertBefore(btnDown, li.children[1]);
}
function addUpButtonOnlyToList2(li) { // code to add "Up" button again after it is removed from the second list item by the user
var btnUp = document.createElement('button');
btnUp.className = "up";
btnUp.textContent = "Up";
li.insertBefore(btnUp, li.children[0]);
}
//The function declared below forms the three buttons namely "Up", "Down", and "Remove"
function formButtons() {
for (i = 0; i < lis.length; i += 1) { // for loop, 'lis' is HTMLCollection of list items)
if (i == 0) { //we want to remove the 'Up' button in the first element with this condition
addButtonsToList(lis[i]); //function call which add the buttons to specified place on page as per the loop and condition
let firstBtn = lis[i].firstElementChild; // select the first button
lis[i].removeChild(firstBtn); //remove the 'Up' button here
} else if (i == 3) { //the 'down' button is removed from the last list item (last li has index of 3)
addButtonsToList(lis[i]); //function call to add buttons
let lastBtn = lis[i].lastElementChild.previousElementSibling; // select the 'down' button in the last list item
lis[i].removeChild(lastBtn); // remove the down button in the last list item
}
else {
addButtonsToList(lis[i]); //add all three button to the second and third list item
}
}
}
listUl.addEventListener('click', (event) => { //code in the line 33 to line 56 was already written by guil hernandez
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
} // code upto here is written by guil hernandez
// In the code below we check that if after clicking 'up' or 'down' button in the page
// the 'Up' button from the second list item (index 1) has taken a new place in the place
// of the first list item. Also note that this code will only run when any of buttons in the Ul Lists is clicked
if (lis[0].querySelector('button.up')) {
removeButtonsFromList(lis[0]); // if there is an "Up" button in the first list item we remove it here
addUpButtonOnlyToList2(lis[1]);// and whenever there is an "up" button in the first list after pressing a button that means we will need to add an up button in the second list item
}
//similar to the code above the below code checks for the presence of "Down" button
//in the last list item and remove it. This code also adds a "Down" button
//in the penultimate list item.
if (lis[3].querySelector('button.down')) {
removeDownButton (lis[3]);
addDownButtonOnly2ndLastListItem (lis[2]);
}
}); // Event Listner code ends here
toggleList.addEventListener('click', () => {//code from line 76 to line 100 was written by guil hernandez
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display = 'block';
}
else {
toggleList.textContent = 'Show list';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild(li);
addItemInput.value = '';
}); //code from line 91 to here is written by guil hernandez
formButtons(); //This is a function Call to form the three buttons on the page