Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial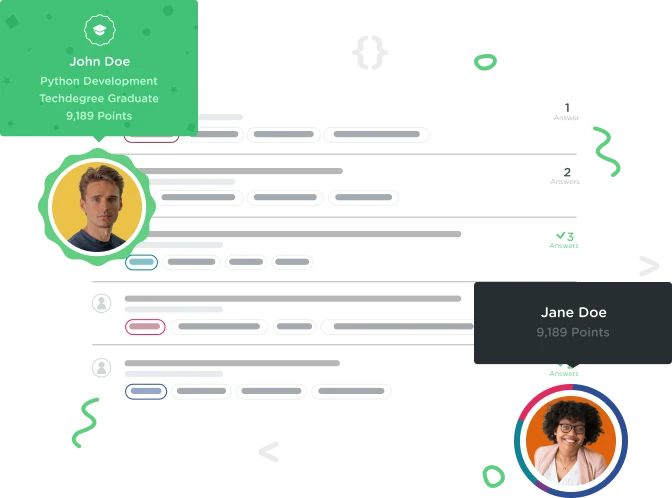

Siddharth Pande
9,046 Pointsreview my code please
// 1: Select the element with the ID 'about'.
// Store the element in the variable `about`.
const about = document.getElementById('about');
about.style.border = "2px solid firebrick";
// 2: Select all the <h2> elements in the document.
// Set the color of the <h2> elements to a different color.
const h2 = document.querySelectorAll("h2");
h2.forEach(function(elem){
elem.style.color = "red"
});
// 3: Select all elements with the class '.card'.
// Set their background color to the color of your choice.
document.querySelectorAll('.card').forEach(function(elem){
elem.style.backgroundColor="yellow"
});
// 4: Select only the first <ul> in the document.
// Assign it to a variable named `ul`.
var ul = document.querySelector('ul');
ul.style.border = "2px solid indigo";
// 5: Select only the second element with the class '.container'.
// Assign it to a variable named `container`.
var container = document.querySelector('.container:nth-of-type(2)');
container.style.backgroundColor = "royalblue";
// 6: Select all <a> elements that have a 'title' attribute.
// Set their color value to the color of your choice.
var a = document.querySelectorAll('[title]');
console.log(a);
a.forEach(function(elem){
elem.style.color="yellow"
});
1 Answer
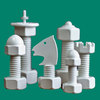
Steven Parker
229,784 PointsThis looks pretty good except for this item:
// 5: Select only the second element with the class '.container'.
var container = document.querySelector('.container:nth-of-type(2)');
This might work, but only if the first element with that class does not happen to be the second item of it's type in the same parent (and the second one is). Otherwise, this might select some other element having that class.
To be sure to select the second element with the class '.container', regardless of type, siblings, or parent element:
// 5: Select only the second element with the class '.container'.
var container = document.querySelectorAll(".container")[1];
This uses a different method but is similar to what is done in the video.
Siddharth Pande
9,046 PointsSiddharth Pande
9,046 PointsThanks for reply. The point you discussed is note worthy and I will implement your suggestions. I too was unsure of the nth of type selectors.