Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial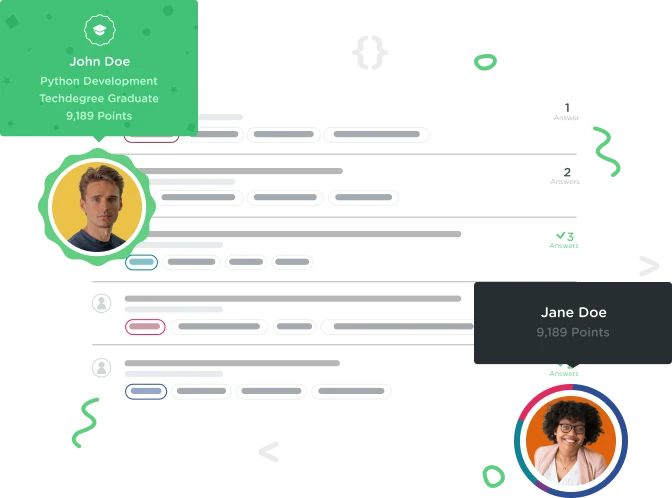

Yarstan Jae
Courses Plus Student 748 PointsRe-write the code to use a do...while loop. What's wrong with my code?
why this gives me an error?
var secret;
do{
secret += prompt("What is the secret password?");
}
while (secret !== "sesame") {
document.write("You know the secret password. Welcome.");
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
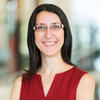
Louise St. Germain
19,424 PointsHi Yara,
You're getting an error for two reasons, both of which are small and easy to fix.
First, your line:
secret += prompt("What is the secret password?");
+= is the same thing as
secret = secret + prompt("What is the secret password?");
So it concatenates the new password to the old password and checks that. If the computer tries 100 times, you will have a gigantic password string of 100 passwords strung together, which is never going to match. Instead, just use a regular = sign, like you see on the first line of the original challenge.
// Do this:
secret = prompt("What is the secret password?");
Second, your while condition is good, but it doesn't take { } afterwards. The do-while template looks like this:
do{
// Stuff
}
while ([this condition is true])
// No curly braces after... the do-while is done now and
// the code just continues per normal after this.
So you just need to take out the set of curly braces after while (), and have the document.write statement by itself on a line (not inside curly braces).
Those two things should make it work! Good luck! :-)