Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial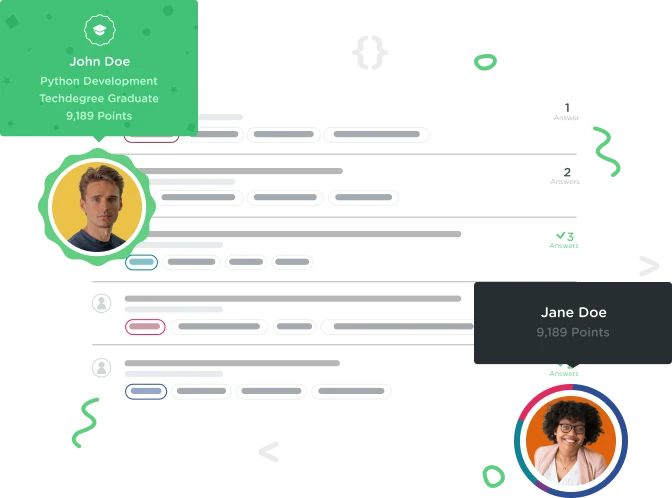

Marcel Pavel
Full Stack JavaScript Techdegree Student 962 Points(RGB + HEX) My solution for the JS Refractor Challenge
Here is my take on the JS Refractor Challenge. I wanted to try some random generated HEX values too. My initial code was 14 lines long, but then I stumbled upon a function made by a user named Dimitry K. in Paul Irish's blog that is only one line of code.
I decided to split the page into 2 columns so I can compare the two codes better. Here is my workspace
The CSS
#color {
text-align: center;
}
div.half {
width: 50%;
float: left;
}
div.wrap {
display: inline-block;
width: 100px;
height: 100px;
}
div.container {
width: 50px;
height: 50px;
border-radius: 50%;
margin: auto;
}
div.info {
display:block;
font-size: 11px;
color: #888;
font-style: italic;
}
The RGB method
/*
RANDOM RGB VALUES GENERATOR
*/
var html = ''; //declaring a blank variable in the global scope for writing to the document
var rgbColor; //declaring the variable in the global scope which stores the rgb value for the random color
/*
this is the function that creates the random rgb value and stores the information
into the rgbColor variable. When the function is called in the for loop, the rgbColor variable
will have different values.
*/
function rgbShuffle() {
var red = Math.floor(Math.random() * 256 ); //declaring a variable for the red value
var green = Math.floor(Math.random() * 256 ); //declaring a variable for the green value
var blue = Math.floor(Math.random() * 256 ); //declaring a variable for the red value
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; //combines the red, green and blue values to form the rgb value and stores it in the rgbColor variable
}
/*
this is the loop that creates circle divs with random colors generated by calling the rgbShuffle() function.
It also has an info div which writes to the document the rgb value for every circle div generated.
*/
for (var i = 0; i < 20; i++) {
rgbShuffle();
html += '<div class="wrap"><div class="container" style="background-color:' + rgbColor + '"></div><div class="info">' + rgbColor + '</div></div>'; //increments the randomly generated divs by 1 until there are 20 divs generated.
}
document.write('<div class="half"><h1>The RGB method</h1>' + html + '</div>'); //writes the randomly generated divs to the document
The HEX method
/*
RANDOM HEX VALUES GENERATOR
Below is a program that generates random HEX values instead or RGB values.
It's basically the same program as above, except for the function hexShuffle()
*/
var htmlHEX = '';
var hexColor;
/*
The solution I found came from Paul Irishβs blog - 2009 from a user named Dimitry K
(http://www.paulirish.com/2009/random-hex-color-code-snippets/#comment-2619723663).
Initialy my solution was with 14 lines of code, but his has just 1 line of code. Awesome
*/
function hexShuffle() {
/*
//the "000000" string at the begining is in case the Math.random returns 0.
If that's the case the function would give an error. So the Math.random generates a random number,
then that number is converted into a base 16 string with letters and numbers, then only the charaters f
rom 2 to 7 are sliced, transformed to uppercase and then only the 6 characters from the right to the left are kept.
*/
hexColor = '#' + ("000000" + Math.random().toString(16).slice(2, 8).toUpperCase()).slice(-6);
}
for (var i = 0; i < 20; i++) {
hexShuffle();
htmlHEX += '<div class="wrap"><div class="container" style="background-color:' + hexColor + '"></div><div class="info">' + hexColor + '</div></div>';
}
document.write('<div class="half"><h1>The HEX method</h1>' + htmlHEX + '</div>');
What do you guys think? Can I improve the code further?