Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial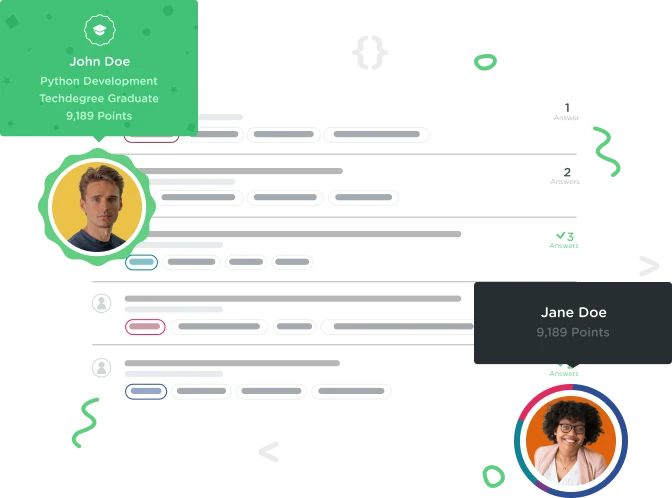

tshuutheniemvula
7,251 PointsRibbit: Null pointer at User Adapter
I have extensively modified the Ribbit application in terms of design and some functionality but I've been getting a null pointer error that originates at:
String email = user.getEmail().toLowerCase();
line of UserApapter. The app loads but as soon as it reaches Main activity, it crashes. Debug shows there's a view that returns as empty but I can't identify which one it is. My UserAdapter code is as follows:
package com.thetechguys.penamoroo1p3.adapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.TextView;
import com.thetechguys.penamoroo1p3.utils.MD5util;
import com.parse.ParseUser;
import com.squareup.picasso.Picasso;
import com.thetechguys.penamoroo1p3.R;
import java.util.List;
/**
* Created by TheBank on 17/2/15.
*/
public class UserAdapter extends ArrayAdapter<ParseUser>{
protected Context mContext;
protected List<ParseUser> mUsers;
public UserAdapter(Context context, List<ParseUser> users) {
super(context, R.layout.message_item, users);
mContext = context;
mUsers = users;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.user_item, null);
holder = new ViewHolder();
holder.userImageView = (ImageView)convertView.findViewById(R.id.userImageView);
holder.nameLabel = (TextView)convertView.findViewById(R.id.nameLabel);
holder.checkImageView = (ImageView)convertView.findViewById(R.id.checkImageView);
convertView.setTag(holder);
}
else {
holder = (ViewHolder)convertView.getTag();
}
ParseUser user = mUsers.get(position);
String email = user.getEmail().toLowerCase();
if (email.equals("")) {
holder.userImageView.setImageResource(R.drawable.avatar_empty);
}
else {
String hash = MD5util.md5Hex(email);
String gravatarUrl = "http://www.gravatar.com/avatar/" + hash +
"?s=204&d=404";
Picasso.with(mContext)
.load(gravatarUrl)
.placeholder(R.drawable.avatar_empty)
.into(holder.userImageView);
}
holder.nameLabel.setText(user.getUsername());
GridView gridView = (GridView)parent;
if (gridView.isItemChecked(position)) {
holder.checkImageView.setVisibility(View.VISIBLE);
}
else {
holder.checkImageView.setVisibility(View.INVISIBLE);
}
return convertView;
}
private static class ViewHolder{
ImageView userImageView;
TextView nameLabel;
ImageView checkImageView;
}
public void refill(List<ParseUser> users) {
mUsers.clear();
mUsers.addAll(users);
notifyDataSetChanged();
}
}
3 Answers

Daniel Hartin
18,106 PointsHi
Sorry it's taken a while, I've been busy with work. Okay so if this line is causing trouble have you used debug mode to see what the value of users is when you initialise the class? if null is passed into class as a parameter there will be no ParseUser object in the List.
Try something like the below
ParseUser user = mUsers.get(position);
String email = "";
if (user != null)
email = user.getEmail().toLowerCase();
if (email.equals("")) {
holder.userImageView.setImageResource(R.drawable.avatar_empty);
}
This code checks to make sure the ParseUser object has been initialised so shouldn't error. Failing this I would suggest stepping through your code using breakpoints to look at the values inside the variables, I find the majority of bugs can be spotted doing this.
Hope this helps Daniel

tshuutheniemvula
7,251 PointsHey Dan! Happy New New and all!
Thanks for the suggestions! I've been stepping through the code in Debug mode for a while now and funny thing is there seems to be no major null values expressed in debugger, both email and users actually get the correct values from Parse when I step over but when I run it, for some reason it crashes. It's very frustrating to say the least.

Daniel Hartin
18,106 PointsHi
It seems strange to have everything have a value in debug mode but not while running. Have you made sure to only try adding data to the view once your asynchronous task (which is querying parse) has completed. It might help to see your main activity code as well if you can post it please

tshuutheniemvula
7,251 PointsHi,
my main activity code as follows:
public class MyActivity extends FragmentActivity implements ActionBar.TabListener {
public static String TAG = MyActivity.class.getSimpleName();
public static final int TAKE_PHOTO_REQUEST = 0;
public static final int TAKE_VIDEO_REQUEST = 1;
public static final int PICK_PHOTO_REQUEST = 2;
public static final int PICK_VIDEO_REQUEST = 3;
public static final int MEDIA_TYPE_IMAGE = 4;
public static final int MEDIA_TYPE_VIDEO = 5;
public static final int FILE_SIZE_LIMIT = 1024*1024*10; //5MB
protected Uri mMediaUri;
protected DialogInterface.OnClickListener mDialogListener =
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
switch (i) {
case 0: //Picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if (mMediaUri == null){
//display error
Toast.makeText(MyActivity.this, R.string.error_external_storage, Toast.LENGTH_LONG).show();
}
else{
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: //Video
Intent takeVideoIntent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_VIDEO);
if (mMediaUri == null) {
//display error
Toast.makeText(MyActivity.this, R.string.error_external_storage, Toast.LENGTH_LONG).show();
}
else{
takeVideoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
takeVideoIntent.putExtra(MediaStore.EXTRA_DURATION_LIMIT, 10);
takeVideoIntent.putExtra(MediaStore.EXTRA_VIDEO_QUALITY, 0);
startActivityForResult(takeVideoIntent,TAKE_VIDEO_REQUEST);
}
break;
case 2: //Choose Pic
Intent choosePhotoIntent = new Intent(Intent.ACTION_GET_CONTENT);
choosePhotoIntent.setType("image/*");
startActivityForResult(choosePhotoIntent, PICK_PHOTO_REQUEST);
break;
case 3: //Choose Video
Intent chooseVideoIntent = new Intent(Intent.ACTION_GET_CONTENT);
chooseVideoIntent.setType("video/*");
Toast.makeText(MyActivity.this,getString(R.string.video_message), Toast.LENGTH_LONG).show();
startActivityForResult(chooseVideoIntent, PICK_VIDEO_REQUEST);
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
// To be safe, you should check that the SDCard is mounted
// using Environment.getExternalStorageState() before doing this.
if (isExternalStorageAvailable()) {
//Get Uri
//1. Get ex storage directory
String appName = MyActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(
Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES), appName);
//2. Create own subdirectory
if (!mediaStorageDir.exists()) {
if (!mediaStorageDir.mkdirs()) {
Log.e(TAG, "Failed to create directory");
return null;
}
}
//3.Create file name
//4.Create the file
File mediaFile;
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
if (mediaType == MEDIA_TYPE_IMAGE) {
mediaFile = new File(path + "IMG_" + timestamp + ".jpeg");
} else if (mediaType == MEDIA_TYPE_VIDEO) {
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
} else {
return null;
}
Log.d(TAG, "File" + Uri.fromFile(mediaFile));
//5. return file's Uri
return Uri.fromFile(mediaFile);
}
else {
return null;
}
}
private boolean isExternalStorageAvailable() {
String state = Environment.getExternalStorageState();
if (state.equals(Environment.MEDIA_MOUNTED)){
return true;
}
else{
return false;
}
}
};
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
* may be best to switch to a
* {@link android.support.v13.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_my);
ParseAnalytics.trackAppOpened(getIntent());
ButterKnife.inject(this);
//ParseUser.logOut();
ParseUser currentUser = ParseUser.getCurrentUser();
if(currentUser==null){
GoToLogin();
}
else{
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getActionBar();
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this,getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
// When swiping between different sections, select the corresponding
// tab. We can also use ActionBar.Tab#select() to do this if we have
// a reference to the Tab.
mViewPager.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
actionBar.addTab(
actionBar.newTab()
.setIcon(mSectionsPagerAdapter.getIcon(i))
.setTabListener(this));
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
if (requestCode == PICK_PHOTO_REQUEST || requestCode == PICK_VIDEO_REQUEST) {
if (data == null) {
Toast.makeText(this, getString(R.string.general_error), Toast.LENGTH_LONG).show();
} else {
mMediaUri = data.getData();
}
Log.i(TAG, "Media URI: " + mMediaUri);
if (requestCode == PICK_VIDEO_REQUEST) {
//make sure file less than 10MB
int fileSize = 0;
InputStream inputStream = null;
try {
inputStream = getContentResolver().openInputStream(mMediaUri);
fileSize = inputStream.available();
} catch (FileNotFoundException e) {
Toast.makeText(this, getString(R.string.filesize_error), Toast.LENGTH_LONG).show();
return;
} catch (IOException e) {
Toast.makeText(this, getString(R.string.filesize_error), Toast.LENGTH_LONG).show();
return;
} finally {
try {
inputStream.close();
} catch (IOException e) {
}
}
if (fileSize >= FILE_SIZE_LIMIT) {
Toast.makeText(this, getString(R.string.file_size_warning), Toast.LENGTH_LONG).show();
return;
}
}
} else {
//add to gallery
Intent mediaScanIntent = new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
mediaScanIntent.setData(mMediaUri);
sendBroadcast(mediaScanIntent);
}
Intent recipientIntent = new Intent(this, RecipientsActivity.class);
recipientIntent.setData(mMediaUri);
String fileType;
if (requestCode == PICK_PHOTO_REQUEST || requestCode == TAKE_PHOTO_REQUEST) {
fileType = ParseConstants.TYPE_IMAGE;
} else {
fileType = ParseConstants.TYPE_VIDEO;
}
recipientIntent.putExtra(ParseConstants.KEY_FILE_TYPE, fileType);
startActivity(recipientIntent);
}
else if(resultCode != RESULT_CANCELED){
Toast.makeText(this,R.string.general_error, Toast.LENGTH_LONG).show();
}
}
private void GoToLogin() {
Intent intent = new Intent(this,LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.my, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int itemId = item.getItemId();
switch(itemId){
case R.id.action_logout_item:
ParseUser.logOut();
GoToLogin();
break;
case R.id.action_goto_settings:
Intent intent3 = new Intent(this, SettingsActivity.class);
startActivity(intent3);
break;
case R.id.Createpicture:
Intent intent1= new Intent(this, MotionViewsActivity.class);
startActivity(intent1);
break;
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
break;
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
break;
//return super.onOptionsItemSelected(item);
//default: return super.onOptionsItemSelected(item);
}
return super.onOptionsItemSelected(item);
}
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
@Override
protected void onResume() {
super.onResume();
AppEventsLogger.activateApp(this);
}
@Override
protected void onPause() {
super.onPause();
// Logs 'app deactivate' App Event.
AppEventsLogger.deactivateApp(this);
}
}
I'll try looking at what the async task returns also.

Daniel Hartin
18,106 PointsOkay I can't see anywhere in your code where the GridView is initalised and the ArrayAdapter is referenced. Is that in another class extending Fragment? I'm assuming something goes wrong when the parent activity tries to inflate the fragment view, can you post your fragment code as well please (or wherever the gridview is referenced)?
Daniel

tshuutheniemvula
7,251 PointsYeah I was thinking I should have posted Fragment code instead, here it is:
public class FriendsFragment extends Fragment {
public static final String TAG = FriendsFragment.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendRelation;
protected ParseUser mCurrentUser;
protected GridView mGridView;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.user_grid, container, false);
mGridView = (GridView)rootView.findViewById(R.id.friendsGrid);
TextView emptyTextView = (TextView)rootView.findViewById(android.R.id.empty);
mGridView.setEmptyView(emptyTextView);
return rootView;
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity().setProgressBarIndeterminate(true);
ParseQuery<ParseUser> query = mFriendRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
getActivity().setProgressBarIndeterminate(false);
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
if(mGridView.getAdapter() == null){
UserAdapter adapter = new UserAdapter(getActivity(), mFriends);
mGridView.setAdapter(adapter);}
else{
((UserAdapter)mGridView.getAdapter()).refill(mFriends);
}
}
else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}

tshuutheniemvula
7,251 PointsHi Dan,
Have you managed to take a look at fragment code?

Daniel Hartin
18,106 PointsHi, Sorry I hadn't got back in touch I've been busy recently with uni. I'm struggling to see where it's going wrong if i'm honest. I would suggest splitting up your main activity into separate classes as there is an awful lot going on in the same code and is quite confusing (but only to make it easier for you, if that's how you work).
I'm currently working on an app which contains an expandable listview inside of a fragment inside a tabbed view so it is not a million miles away from what you're trying to achieve I think, my code goes a different way around the problem and is nowhere near finished but does work and I can't spot the snag with your code where it differs from mine.
I assume you have already checked the normal culprits i.e. that there is a GridView inside your user_grid layout with the id of friendsGrid?
I'm sorry I can't be of anymore help to you. Perhaps someone better than I can assist you?
Thanks Daniel
tshuutheniemvula
7,251 Pointstshuutheniemvula
7,251 PointsLogcat:
Process: com.thetechguys.penamoroo1p3, PID: 32572 java.lang.NullPointerException at com.thetechguys.penamoroo1p3.adapters.UserAdapter.getView(UserAdapter.java:53) at android.widget.AbsListView.obtainView(AbsListView.java:2743) at android.widget.GridView.makeAndAddView(GridView.java:1366) at android.widget.GridView.makeRow(GridView.java:346) at android.widget.GridView.fillDown(GridView.java:288) at android.widget.GridView.fillFromTop(GridView.java:422) at android.widget.GridView.layoutChildren(GridView.java:1254) at android.widget.AbsListView.onLayout(AbsListView.java:2564) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.widget.RelativeLayout.onLayout(RelativeLayout.java:1055) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.widget.FrameLayout.layoutChildren(FrameLayout.java:453) at android.widget.FrameLayout.onLayout(FrameLayout.java:388) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.support.v4.view.ViewPager.onLayout(ViewPager.java:1594) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.widget.FrameLayout.layoutChildren(FrameLayout.java:453) at android.widget.FrameLayout.onLayout(FrameLayout.java:388) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at com.android.internal.widget.ActionBarOverlayLayout.onLayout(ActionBarOverlayLayout.java:459) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.widget.FrameLayout.layoutChildren(FrameLayout.java:453) at android.widget.FrameLayout.onLayout(FrameLayout.java:388) at android.view.View.layout(View.java:15752) at android.view.ViewGroup.layout(ViewGroup.java:4881) at android.view.ViewRootImpl.performLayout(ViewRootImpl.java:2358) at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:2071) at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1256) at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:6632) at android.view.Choreographer$CallbackRecord.run(Choreographer.java:813) at android.view.Choreographer.doCallbacks(Choreographer.java:613) at android.view.Choreographer.doFrame(Choreographer.java:583) at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:799) at android.os.Handler.handleCallback(Handler.java:733) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:146) at android.app.ActivityThread.main(ActivityThread.java:5598) at java.lang.reflect.Method.invokeNative(Native Method) at java.lang.reflect.Method.invoke(Method.java:515) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1283) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1099) at dalvik.system.NativeStart.main(Native Method)