Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial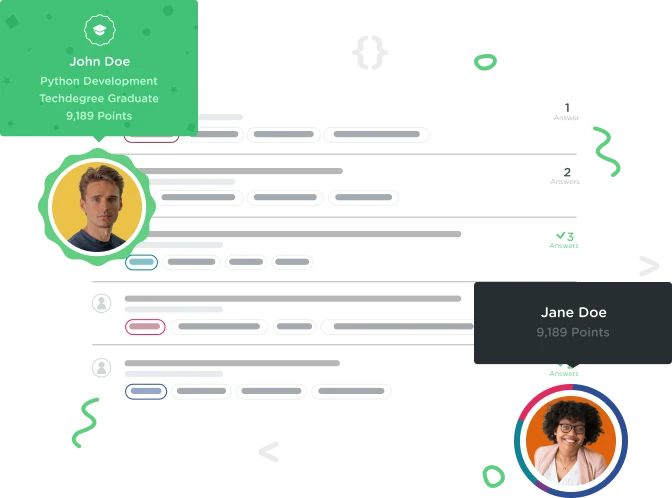

Jared Gross
2,686 PointsRibbit project: converting table view to scrolling table view
Hey guys, I was playing around with the Treehouse demo code and a scrollingTableView code I found on the internet last night and was able to integrate the two codes together and get everything working just fine. Then I mistakenly saved over my project with a previous version of itself and haven't been able to get it back working again. I've gotten it back to where It is receiving the messages from Parse but not displaying them in thumbnail form on the scrollingTableView and also when I tap on the messages I am not taken to the detailViewController like I had set up my segue to do. If anyone has any idea where I am going wrong this time around please give me a heads up! Cheers!
@interface AlbumsViewController ()<PPImageScrollingTableViewCellDelegate>
@property (strong, nonatomic) NSArray *images;
@end
@implementation AlbumsViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.title = @"Albums";
[self.tableView setBackgroundColor:[UIColor grayColor]];
self.moviePlayer = [[MPMoviePlayerController alloc] init];
}
- (void)viewWillAppear:(BOOL)animated {
[super viewWillAppear:animated];
PFQuery *query = [PFQuery queryWithClassName:@"Messages"];
[query whereKey:@"recipientIds" equalTo:[[PFUser currentUser] objectId]];
[query orderByDescending:@"createdAt"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error: %@ %@", error, [error userInfo]);
}
else {
// We found messages!
self.messages = objects;
[self.tableView reloadData];
NSLog(@"Retrieved %d messages", [self.messages count]);
}
}];
}
pragma mark - UITableViewDataSource
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return [self.messages count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
PPImageScrollingTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
PFObject *message = [self.messages objectAtIndex:indexPath.row];
cell.textLabel.text = [message objectForKey:@"senderName"];
NSString *fileType = [message objectForKey:@"fileType"];
if ([fileType isEqualToString:@"image"]) {
cell.imageView.image = [UIImage imageNamed:@"icon_image"];
NSLog(@"it's an image");
}
else {
cell.imageView.image = [UIImage imageNamed:@"icon_video"];
NSLog(@"it's a video");
}
return cell;
}
pragma mark - UITableViewDelegate
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
return 150;
}
pragma mark - PPImageScrollingTableViewCellDelegate
- (void)scrollingTableViewCell:(PPImageScrollingTableViewCell *)scrollingTableViewCell didSelectImageAtIndexPath:(NSIndexPath*)indexPathOfImage atCategoryRowIndex:(NSInteger)categoryRowIndex
{
self.selectedMessage = [self.messages objectAtIndex:categoryRowIndex];
NSString *fileType = [self.selectedMessage objectForKey:@"fileType"];
if ([fileType isEqualToString:@"image"]) {
[self performSegueWithIdentifier:@"showDetail" sender:self];
}
else {
// File type is video
PFFile *videoFile = [self.selectedMessage objectForKey:@"file"];
NSURL *fileUrl = [NSURL URLWithString:videoFile.url];
self.moviePlayer.contentURL = fileUrl;
[self.moviePlayer prepareToPlay];
[self.moviePlayer thumbnailImageAtTime:0 timeOption:MPMovieTimeOptionNearestKeyFrame];
// Add it to the view controller so we can see it
[self.view addSubview:self.moviePlayer.view];
[self.moviePlayer setFullscreen:YES animated:YES];
}
[self.selectedMessage saveInBackground];
}
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([segue.identifier isEqualToString:@"showDetail"]) {
[segue.destinationViewController setHidesBottomBarWhenPushed:YES];
DetailViewController *imageViewController = (DetailViewController *)segue.destinationViewController;
imageViewController.message = self.selectedMessage;
}
}
@end
1 Answer
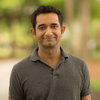
Amit Bijlani
Treehouse Guest TeacherYou need to check to see if you actually have a segue in your storyboard called "showDetail". If not, make sure the names match up.