Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial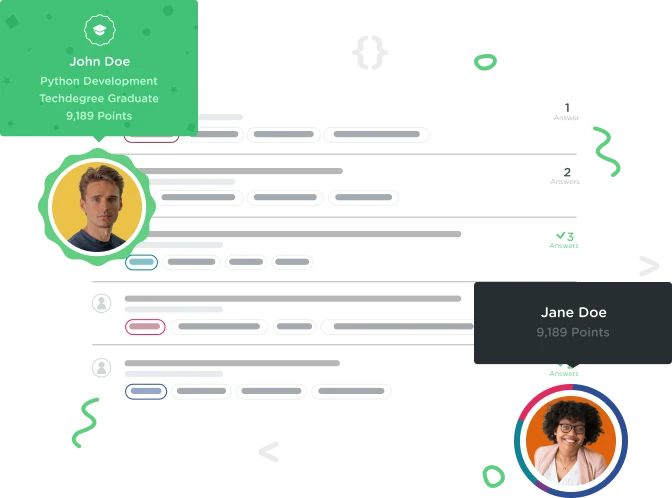

Cortney Burgess
10,758 PointsRight now, the three <td> elements have information about the original movie as static pieces of text. Replace each of those with PHP commands that INSTEAD display information about the new movie from the array instead. (Be sure to leave the <td> tags int
<?php $movie = array( "title" => "The Empire Strikes Back", "year"=>"1980",
"director" => "Irvin Kershner",
"imdb_rating"=> "8.8",
"imdb_ranking"=> "11"); ?>
<h1><?php echo $movie["title"]; ?> (1980)</h1>
<tr>
<th>Director</th>
<td>Irvin Kershner</td>
</tr>
<tr>
<th>IMDB Rating</th>
<td>8.8</td>
</tr>
<tr>
<th>IMDB Ranking</th>
<td>11</td>
</tr>
</table>
16 Answers
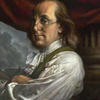
Jeff Busch
19,287 PointsHi GURJAP,
I realize it can be confusing mixing php with html, and there are different ways of doing it, but it's a big part of php. After some practice it will become easier. Below is the code.
Jeff
<?php
$movie = array(
"title" => "The Empire Strikes Back",
"year" => 1980,
"director" => "Irvin Kershner",
"imdb_rating" => 8.8,
"imdb_ranking" => 11
);
?>
<h1><?php echo $movie["title"]; ?> (<?php echo $movie["year"]; ?>)</h1>
<table>
<tr>
<th>Director</th>
<td><?php echo $movie["director"]; ?></td>
</tr>
<tr>
<th>IMDB Rating</th>
<td><?php echo $movie["imdb_rating"]; ?></td>
</tr>
<tr>
<th>IMDB Ranking</th>
<td><?php echo $movie["imdb_ranking"]; ?></td>
</tr>
</table>
Also, if you look just below the text box area you will see something that says Markdown Cheetsheet. Also, there is a post here titled: Posting Code to the Forum by Dave McFarland. If you don't understand that maybe this will make sense: How to display code at Treehouse.

Cortney Burgess
10,758 Points <th>Director</th>
<td>
<?php echo $movie["director"]; ?> </td>
<th>IMDB Rating</th>
<td>
<?php echo $movie["imdb_rating"];?> </td>
<th>IMDB Ranking</th>
<td>
<?php echo $movie["imdb_ranking"]; ?> </td>
</tr>
</table>
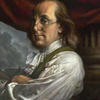
Jeff Busch
19,287 PointsHi Cortney,
I don't see any code related to the question. Below is a snippet of the code.
Jeff
<tr>
<th>Director</th>
<td><?php echo $movie["director"]; ?></td>
</tr>
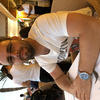
Matthew Underhill
20,363 PointsHi Cortney,
All you have to do is echo each part of the array instead of leaving the plain text there. Each part of the $movie array can be referenced using the square brackets, followed by the array element in quotation marks. Jeff has done one example for you above.

Cortney Burgess
10,758 Pointsi typed this in and it is not working
<td> Director <?php echo $movie["director"]; ?> IMDB Rating <?php echo $movie["imdb_rating"]; ?> IMDB Ranking <?php echo $movie["imdb_ranking"]; ?> </td>
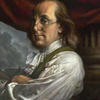
Jeff Busch
19,287 PointsCortney,
You are supposed to leave the html intact. I'll give you the first table row.
<tr>
<th>Director</th>
<td><?php echo $movie["director"]; ?></td>
</tr>

Cortney Burgess
10,758 Points <th>Director</th>
<td>
<?php echo $movie["director"]; ?> </td>
<th>IMDB Rating</th>
<td>
<?php echo $movie["imdb_rating"];?> </td>
<th>IMDB Ranking</th>
<td>
<?php echo $movie["imdb_ranking"]; ?> </td>
</tr>
</table>

Cortney Burgess
10,758 Pointsstill not working
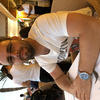
Matthew Underhill
20,363 PointsTry removing the space at the end of your closing PHP brackets and the closing TD tags.
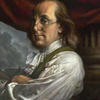
Jeff Busch
19,287 PointsCotney,
If you look at my example you will see that there are two table cells (th /th and td /td) per table row (t r /tr). Both th and td are table cells; th is a table-header-cell and td is table-data-cell. In your code example you have at least three table cells in a table row, at least as far as I can see. Yu can see this in the code challenge although it is not indented.
Hope this helps, Jeff
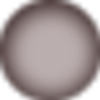
Wes Laing
3,497 Points<?php $movie = array( "title" => "The Empire Strikes Back" , "year" => "1980" , "director" =>"Irvin Kershner","imdb_rating"=>"8.8","imdb_ranking"=>"11"); ?>
<h1><?php echo $movie["title"]; ?> (<?php echo $movie["year"];?>)</h1> <table> <tr> <th>Director</th> <td><?php echo $movie["director"];?></td> <th>IMDB Rating</th> <td><?php echo $movie["imdb_rating"];?></td> <th>IMDB Ranking</th> <td><?php echo $movie["imdb_ranking"];?></td> </tr> </table>
why doesn't this work
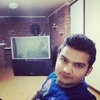
GURJAP SINGH
7,556 PointsHere is my code, however I am getting an error "HTML IS NOT QUITE RIGHT"
<?php $movie = array ( title => "The Empire Strikes Back", year => 1980, director => "Irvin Kershner", imdb_rating => 8.8, imdb_ranking => 11
); ?>
<h1><?php echo $movie["title"] ?> (<?php echo $movie["year"] ?>)</h1>
<table> <tr> <th>Director</th> <td><?php echo $movie["director"]; ?></td> </tr>
<tr> <th>IMDB Rating</th> <td><?php echo $movie["imdb_rating"]; ?></td> </tr> <tr> <th>IMDB Ranking</th> <td><?php echo $movie["imdb_ranking"]; ?></td> </tr> <tr> </table>
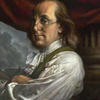
Jeff Busch
19,287 PointsHi GURJAP,
You have left-out all the html. And in the array, remember that the keys are also strings. You are missing two semicolons.
Jeff
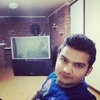
GURJAP SINGH
7,556 PointsThanks for replying Jeff.
I guess i posted the old code. However I am stuck at step 7.
here is the code (sorry for the layout) and
error "Bummer! It looks like the rating is displayed in the browser, but the HTML around it does not seem to be correct. Please double-check the spacing and other formatting."
<?php $movie = array("title" => "The Empire Strikes Back", "year" => 1980, "director" => "Irvin Kershner", "imdb_rating" => 8.8,"imdb_ranking" => 11); ?> <h1><?php echo $movie["title"]; ?> (<?php echo $movie["year"]; ?>)</h1>
<table> <tr> <th>Director</th> <td><?php echo $movie["director"]; ?></td> </tr> <tr> <th>IMDB Rating</th> <td><?php echo $movie["imdb_rating"]; ?> </td> </tr> <tr> <th>IMDB Ranking</th> <td><?php echo $movie["imdb_ranking"]; ?></td>
</table>
is it possible if you can show me the final code as I am unable to complete the Stage without it.
Thanks
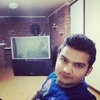
GURJAP SINGH
7,556 PointsThank You very Much Jeff. You are the best.
i compared my code and looked exact same but I don't know where I was getting the error. I am happy that I have completed the Stage. Appreciate your help.

Raz Tzvi
Courses Plus Student 6,323 Points<?php $movie = array( "title" => "The Empire Strikes Back", "year" => 1980, "director" => "Irvin Kershner", "imdb_rating" => 8.8, "imdb_ranking" => 11 ); ?>
<h1><?php echo $movie["title"] . $movie["year"]; ?>)</h1>
<table> <?php foreach ($movie as $ key => $val) {
echo "<tr><th>" . $key . "</th><td>" . $val . "</td></tr>"; } ?> </table>

Joy Manuel
6,464 Points<?php
$movie = [ "title" => "The Empire Strikes Back", "year" => 1980, "director" => "Irvin Kershner", "imdb_rating" => 8.8, "imdb_ranking" => 11, ]; ?>
<h1><?php echo $movie["title"] . $movie["year"]; ?></h1>
<table>
<tr> <th>Director</th>
<td><?php echo $movie["director"]; ?></td>
</tr>
<tr> <th>IMDB Rating</th>
<td><?php echo $movie["imdb_rating"]; ?></td>
</tr>
<tr> <th>IMDB Ranking</th>
<td><?php echo $movie["imdb_ranking"]; ?></td>
</tr>
</table>