Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial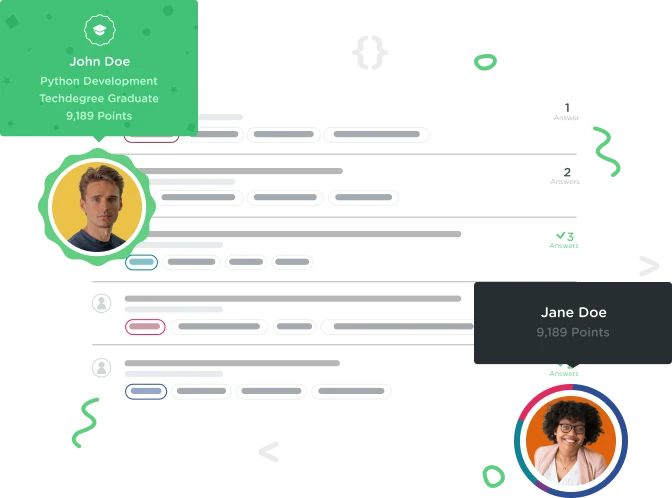

anonymous123
5,794 Pointsround() function python
why does round(4.5) return 4? why does round(-4.5) return -4?
1 Answer
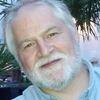
Jeff Muday
Treehouse Moderator 28,716 PointsGood question-- round() will round a value to the "nearest" integer. But if you are on the "knife's edge" of the rounding point you can get what seems like unpredictable results.
Visit this link for further details (14. Floating Point Arithmetic: Issues and Limitations):
https://docs.python.org/2/tutorial/floatingpoint.html
Consider the following program where we refine a string and convert it to floating point. This program will explore the limits of the string format() method as well as float() type cast and round()
# start with a string representation of x
x = '4.49'
# loop keeps adding nines to the end of a string
# e.g. 4.49, 4.499, 4.4999, etc.
for _ in range(100):
x = x + '9'
print 'x = "{}"'.format(x)
print 'float(x) = {}'.format(float(x))
print 'round(float(x)) = {}'.format(round(float(x)))
Ok... here is the seemingly abberant behavior as we approach 4.5. I am using a 32 bit Python 2.7. Your results will be a little different with a 64 bit python, but there will be a "crossover" point as I show below.
# note the float value is not quite 4.5
x = "4.49999999999"
float(x) = 4.49999999999
round(float(x)) = 4.0
# but now the float value becomes 4.5, but it still rounds to 4.0
x = "4.499999999999"
float(x) = 4.5
round(float(x)) = 4.0
x = "4.4999999999999"
float(x) = 4.5
round(float(x)) = 4.0
x = "4.49999999999999"
float(x) = 4.5
round(float(x)) = 4.0
x = "4.499999999999999"
float(x) = 4.5
round(float(x)) = 4.0
# at this point, the rounding of our 4.5 changes to 5.0 here
x = "4.4999999999999999"
float(x) = 4.5
round(float(x)) = 5.0
x = "4.49999999999999999"
float(x) = 4.5
round(float(x)) = 5.0
anonymous123
5,794 Pointsanonymous123
5,794 PointsI read the document that you linked to your response and i read that this problem only really happens to decimal fractions. "Unfortunately, most decimal fractions cannot be represented exactly as binary fractions. A consequence is that, in general, the decimal floating-point numbers you enter are only approximated by the binary floating-point numbers actually stored in the machine."