Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial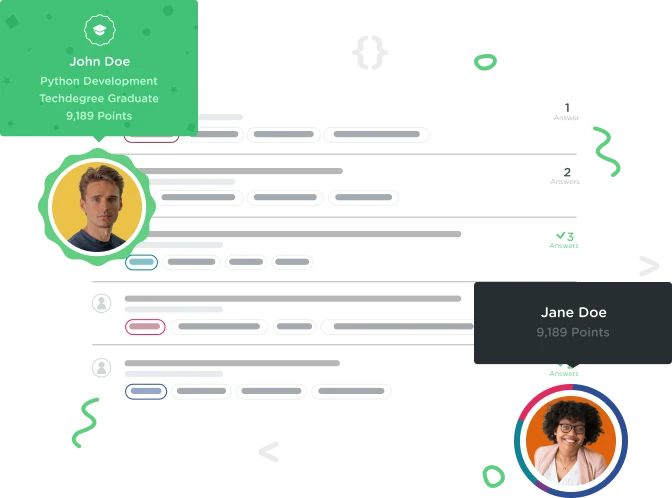
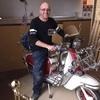
jon kelson
5,149 PointsRoundButton super.init what is wrong with my super init method ?
class Button { var width: Double var height: Double
init(width:Double, height:Double){ self.width = width self.height = height }
func scaleBy(points: Double){ width += points height += points } }
class RoundButton: Button { var cornerRadius: Double = 5.0
init(cornerRadius: Double){ self.cornerRadius = cornerRadius super.init(width: width, height: height)
}
}
3 Answers
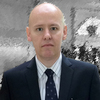
Nathan Tallack
22,159 Pointsclass Button {
var width: Double
var height: Double
init(width:Double, height:Double) {
self.width = width
self.height = height
}
func scaleBy(points: Double) {
width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
init(cornerRadius: Double) {
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
}
}
Ok, I think I have formatted your code correctly. Good idea to use markdown (enclosing your code in tripple ` marks) to ensure it formats right.
You are passing width and height into your super init when those properties are not part of your init method. So you are going to need to take those in also. Your class would then look like this.
class RoundButton: Button {
var cornerRadius: Double = 5.0
init(cornerRadius: Double, width: Double, height: Double) {
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
}
}
You would create the object with an initializer call like this.
let myRoundButton = RoundButton(cornerRadius=2.5, width=20.0, height=5.0)
That way you are passing in all the paramaters your class initializer is going to need to satisify its own initialization and the parents initialization.
One thing you could do is assume your button is a circle in which case you could use the radius value to extrapolate the width/height (same) value. Then your class would look like this.
class RoundButton: Button {
var cornerRadius: Double = 5.0
init(cornerRadius: Double) {
self.cornerRadius = cornerRadius
super.init(width: cornerRadius*2, height: cornerRadius*2)
}
}
That way you only need to pass in the cornerRadius. But in my honest opinion the class would better be called a CircleButton. ;)
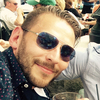
Brian Steele
23,060 Pointsclass RoundButton: Button {
var cornerRadius: Double = 5.0
init(cornerRadius: Double, width: Double, height: Double){
self.cornerRadius = cornerRadius
super.init(width: width, height: height)
}
}
You need to pass the params that are being called with the super.init method into the sub classes init method.
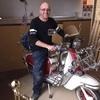
jon kelson
5,149 Pointsok thanks everyone I tried this late last night and it worked even though I'm not sure if it adds up mathematically.
class RoundButton: Button { var cornerRadius: Double = 5.0 init(width: Double, height: Double, cornerRadius: Double){ self.cornerRadius = cornerRadius super.init(width: 5.0, height: 5.0) } }