Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial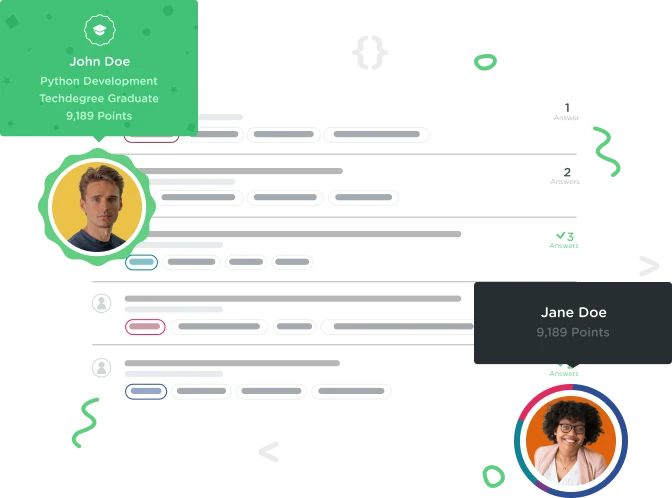

Reuben Haley
Python Development Techdegree Student 233 PointsRounding Up Values
from the video
import math
def split_check(total,number_of_people):
cost_per_person = math.ceil(total / number_of_people)
return cost_per_person
amount_due = (split_check(555,5))
print("Each person owes ${}".format(amount_due))
Is it acceptable to do this round up the amount_due instead of the cost_per_person or the vice versa?
import math
def split_check(total,number_of_people):
cost_per_person = total / number_of_people
return cost_per_person
amount_due = math.ceil(split_check(555,5))
print("Each person owes ${}".format(amount_due))
1 Answer
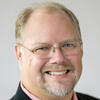
Jason Larson
8,361 PointsConsidering that you're rounding up to the nearest integer, I don't think there would be an issue. There's a margin of error of up to 99 cents this way, so I can't imagine that you'd run into a split that would cause an issue. Even if you weren't rounding by this much, the difference would be minute, so I wouldn't worry about it. Someone who is very good with math may take exception, or if you were doing something that was concerned with the specificity out several decimal points, but for this type of real-world application, either way is fine.
Stylistically, I would recommend that you do the rounding within the function to keep the code cleaner so that all calculations related to splitting the check are handled in one place, and if you decide to change something, everything is together when you're looking at the code. You're not having to jump back and forth between the function and the section where the function is called. I know in this tiny example, that doesn't seem important, but when you're dealing with hundreds or thousands of lines of code, it can make a big difference, especially when troubleshooting.