Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial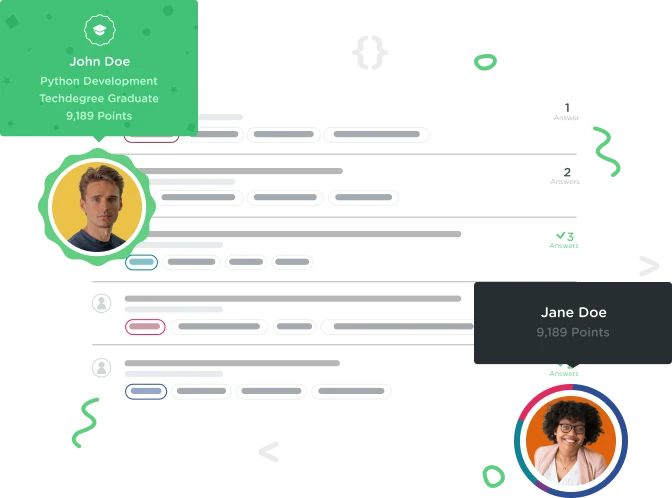
TJ Rogers
8,758 PointsRow Selector one step behind
Having trouble with Row selection in the Blog Reader project. in the NSLog that I set up to test the row selection operation, when I first select a row nothing happens. Then when I select another row, the NSLog returns the index number of the first row that was selected. Then when I select another row, it returns the index number of the row that was previously selected, and so forth. In other words, the selector operation is always one step behind the actual selection.
I don't know if this is related. But the row selector code in the tutorial vid is pre-populate in the TableViewController.m file. But in the most recent version of X-Code, it does not pre-populate and I needed to copy the code as it is written in the vid manually. Perhaps there is another step I need to take besides just copying the code? I'm at a loss here. In any case, here is my code. Thanks!
#import "TableViewController.h"
#import "BlogPost.h"
@interface TableViewController ()
@end
@implementation TableViewController
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
NSURL *blogURL = [NSURL URLWithString:@"http://blog.teamtreehouse.com/api/get_recent_summary/"];
NSData *jsonData = [NSData dataWithContentsOfURL:blogURL];
NSError *error = nil;
NSDictionary *dataDictionary = [NSJSONSerialization JSONObjectWithData:jsonData options:0 error:&error];
self.blogPosts = [NSMutableArray array];
NSArray *blogPostsArray = [dataDictionary objectForKey:@"posts"];
for (NSDictionary *bpDictionary in blogPostsArray) {
BlogPost *blogPost = [[BlogPost alloc] init];
blogPost.title = [bpDictionary objectForKey:@"title"];
blogPost.author = [bpDictionary objectForKey:@"author"];
blogPost.url = [NSURL URLWithString:[bpDictionary objectForKey:@"url"]];
[self.blogPosts addObject:blogPost];
}
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return self.blogPosts.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
BlogPost *blogpostRow = [self.blogPosts objectAtIndex:indexPath.row];
cell.textLabel.text = blogpostRow.title;
cell.detailTextLabel.text = blogpostRow.author;
return cell;
}
#pragma mark - Table view delegate
- (void)tableView:(UITableView *)tableView didDeselectRowAtIndexPath:(NSIndexPath *)indexPath {
NSLog(@"Row Selected: %d", indexPath.row);
}
@end
2 Answers
TJ Rogers
8,758 PointsUgh... nevermind, I just caught the problem. I used the "didDeselectRow..." method instead of "didSelectRow..."

Steve Weinstock
7,872 PointsI have been struggling with this problem of the table cells staying highlighted when I pressed them and not registering a checkmark until I selected another cell. Finally the answer. And just in case anyone else has this problem, here is the answer again, but highlighted (it took me awhile looking at TJ's answer to recognize what the difference was:
In both the CameraViewController.m and the EditFriendsViewController.m files, I had inadvertently the DESELECT version of the method rather than the SELECT version, i.e.:
- (void) tableView: (UITableView*)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
is correct
- (void) tableView: (UITableView*)tableView did
DeSelectRowAtIndexPath:(NSIndexPath *)indexPath
is wrong.
TJ Rogers
8,758 PointsToday - one year later - I made the same mistake, and tried to Google the problem. This came up (facepalm). Glad it helped you too :)