Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial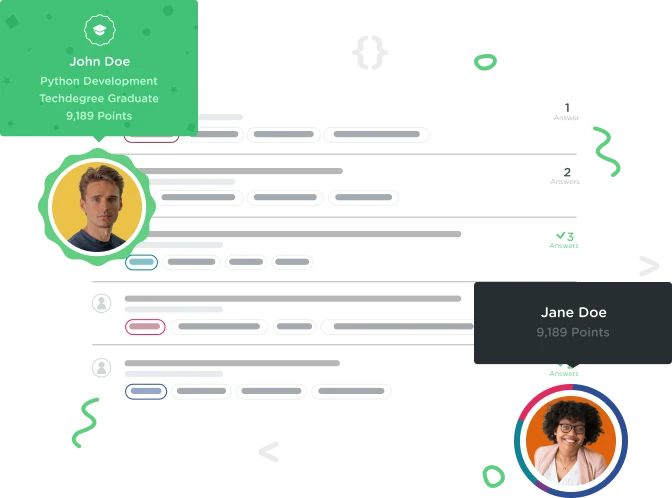

Isaac Stong
4,083 PointsRPG Roller 2 of 2
I'm having an error Can't get the length of hand I've looked at other posts here and it seems like very similar code is working for others any help is appreciated thanks
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(20)
from dice import D20
class Hand(list):
@classmethod
def roll(cls, number):
hand = cls
for rolls in range(number):
hand.append(D20())
return hand
@property
def total(self):
return sum(self)
3 Answers
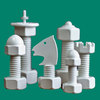
Steven Parker
229,732 PointsYou're very close! But when you create a new instance, place parentheses like you would when calling a function:
hand = cls()

Than Win Hline
1,679 PointsI don't understand this solution. Why we are using hand=cls() and what is the purpose of using classmethod here? I'm stuck with this solution
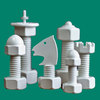
Steven Parker
229,732 PointsNote the example invocation shown in the instructions is "Hand.roll(2)
", so it's obvious that the class itself (and not an instance) is being used to access the method. That lets you know that it should be implemented as a class method.
The instructions also say "I want to get back an instance of Hand ...", and calling "cls()
" is the best-practice way to create a new instance inside a class method.

Than Win Hline
1,679 PointsThank you for the reply. Now I understood
Isaac Stong
4,083 PointsIsaac Stong
4,083 PointsThanks for the quick response Steven! I did as you said and added in the parentheses to pass cls as an instance, unfortunately, this did not get rid of the length problem the same error seems to pop up any ideas on this? Also I should add it complains about not being able to get the length of Hand with a capital H I don't know if that helps any.
Steven Parker
229,732 PointsSteven Parker
229,732 PointsIf I paste your code directly into the challenge and make just that one change, it passes.
Perhaps restart your browser before you try again?
Isaac Stong
4,083 PointsIsaac Stong
4,083 PointsI restarted the browser a few hours later and all is working thank you for the help!