Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial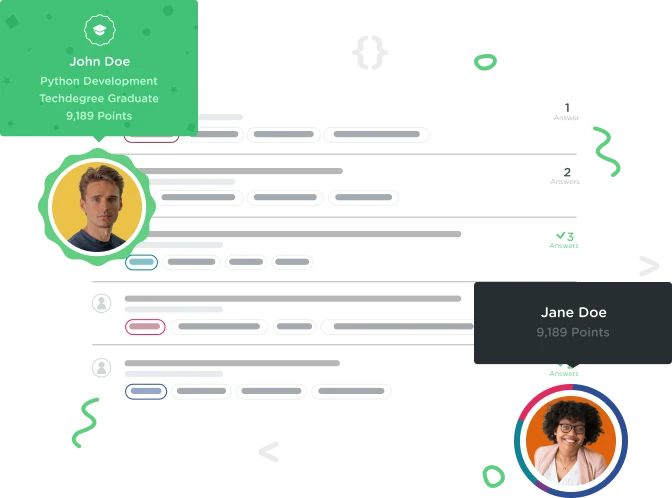
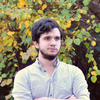
Caleb Sylvest
7,642 PointsRspec Failure/Error on test
I'm running into errors when running tests for both my todo_item_spec.rb
and todo_list_spec.rb
tests.
This is what I see when I run the todo_item_spec.rb
Calebs-MBP:odot calebsylvest$ bin/rspec spec/models/todo_item_spec.rb
F
Failures:
1) TodoItem should belong to todo_list
Failure/Error: it { should belong_to(:todo_list) }
Expected TodoItem to have a belongs_to association called todo_list (TodoItem does not have a todo_list_id foreign key.)
# ./spec/models/todo_item_spec.rb:4:in `block (2 levels) in <top (required)>'
Finished in 0.00813 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/models/todo_item_spec.rb:4 # TodoItem should belong to todo_list
Randomized with seed 7661
And this is what I see when I run the todo_list_spec.rb
Calebs-MBP:odot calebsylvest$ bin/rspec spec/models/todo_list_spec.rb
F
Failures:
1) TodoList should have many todo_items
Failure/Error: it { should have_many(:todo_items) }
Expected TodoList to have a has_many association called todo_items (TodoItem does not have a todo_list_id foreign key.)
# ./spec/models/todo_list_spec.rb:4:in `block (2 levels) in <top (required)>'
Finished in 0.00928 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/models/todo_list_spec.rb:4 # TodoList should have many todo_items
Randomized with seed 52819
I've checked and double-checked the code in my files but still having this issue. Downloaded the project files and replaced my files with the project files, same issue. So, I'm not sure where the connection issue is. Below is the code I'm working with:
My Code
todo_list.rb
class TodoList < ActiveRecord::Base
has_many :todo_items
validates :title, presence: true
validates :title, length: { minimum: 3 }
validates :description, presence: true
validates :description, length: { minimum: 5 }
end
todo_item.rb
class TodoItem < ActiveRecord::Base
belongs_to :todo_list
end
todo_list_spec.rb
require 'spec_helper'
describe TodoList do
it { should have_many(:todo_items) }
end
todo_item_spec.rb
require 'spec_helper'
describe TodoItem do
it { should belong_to(:todo_list) }
end
Any suggestions on what the issue is and how to fix it?
I'm working on my local machine and have had no problems up to this point.
Thanks, Caleb
2 Answers
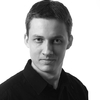
Maciej Czuchnowski
36,441 PointsThis part of the error is the most important one:
TodoItem does not have a todo_list_id foreign key.
Check your db/schema.rb file and see if you have this field in todo_items table. If not, check if any of the migrations were not applied. Create a migration that adds this id field if need be :)

morgan segura
Courses Plus Student 6,934 PointsThe first failure you have: 'belongs_to' and it should be 'belong_to'
the second one you have: 'have_many' and it should be 'has_many'
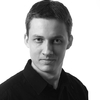
Maciej Czuchnowski
36,441 PointsThese actually come from shoulda_matchers and are correct when used in tests - RSpec knows how to translate them.
Rob Castellucci
10,268 PointsRob Castellucci
10,268 PointsI'm dealing with this same issue. I checked and I have this field in the todo_items table. The only difference between instructor version of schema.rb and my version is the version number which is not the issue. Double checked by using their version number and it still fails. Any ideas what else could be happening?