Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial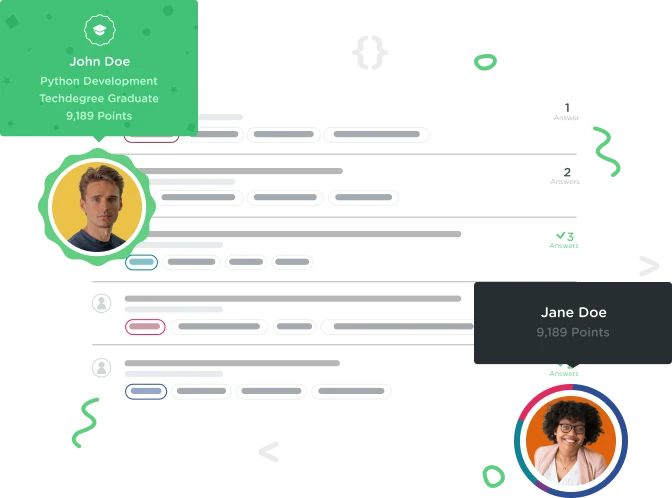
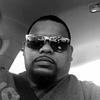
Shane Harnarine
2,562 PointsRuby Basics ("if" Statements) Cant get this to pass but it passes in my terminal. Please Help!
Define a method named check_speed that takes a single number representing a car's speed as a parameter. If the speed is over 55, check_speed should print the message "too fast". If the speed is under 55, check_speed should print the message "too slow". And if the speed is exactly 55, check_speed should print the message "speed OK".
def check_speed (speed)
if check_speed > 55
puts "too fast"
end
if check_speed < 55
puts "too slow"
end
if check_speed == 55
puts "speed OK"
end
end
check_speed 70
I don't why its not passing in treehouse. I use VS code and it passes. Any help would be appreciated. Thanks!
2 Answers
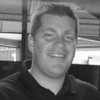
Mike Hickman
19,817 PointsHi Shane,
You're super close. The only issue is that you're continuing to use check_speed as the speed you're comparing against, which is only the name of the method you created. The speed (70 in your example) is being passed into the method as speed. check_speed should only used to define the method name, then not used again until you're actually calling the method on your last line.
Here's an example of the change:
def check_speed (speed)
if speed > 55 # speed instead of check_speed
puts "too fast"
end
end
check_speed 70 # actually calling the check_speed method and passing in 70 as **speed**
For example, if you defined your method as:
def check_speed (potato)
if potato > 55
puts "We'll pass in the same speed, but reference it as potato"
end
end
check_speed 70 # actually calling the check_speed method and passing in 70 as **potato**
You can still call your method at the bottom: check_speed 70, and then use potato > 55, potato < 55, potato == 55, etc in your if statements. Basically, the word you put in parenthesis after the method name is how you'll reference it within your method code.
Hope this makes sense. Have fun!
-Mike
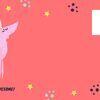
Ana María Benites Rodríguez
1,011 Pointsgreat, thanks for the nice explanation Michael Myers