Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial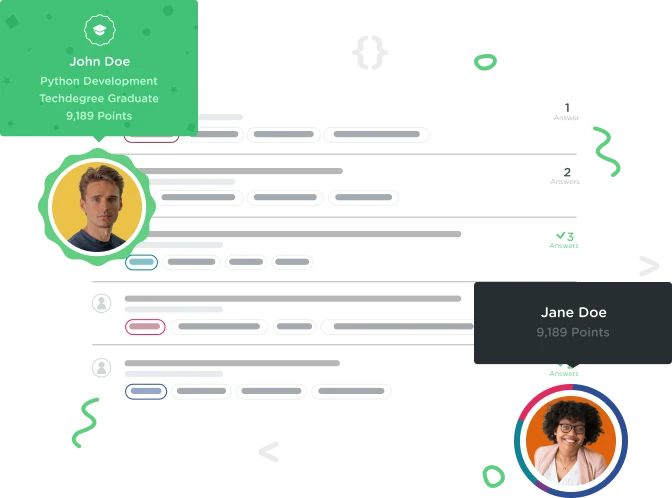
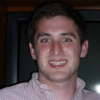
Richard Luick
10,955 PointsRuby Blocks Extra Credit
Hello everyone, I worked on the extra credit for the blocks section of Ruby Foundations and believe I came up with something that works. My program asks for a number of iterations, runs the benchmarking, and then puts out a sorted array with the iterations times. I am open to critiquing and would like to know if anyone has any suggestions or better ways to go about this. If anything in my program is against "protocol" please let me know. My biggest concerns with my program are using the "time_array" as a global variable and also if I should have used a separate method for asking the user how many iterations. Thank you.
class SimpleBenchmarker
def self.go(&block)
$time_array = Array.new
print "How many times should we run the program: "
how_many = gets.chomp.to_i
puts "-----Benchmarking Started-----"
start_time = Time.now
puts "Start Time:\t#{start_time}\n\n"
how_many.times do |a|
print "."
block.call
end
print "\n\n"
end_time = Time.now
puts "End Time:\t#{end_time}\n"
puts "-----Benchmarking Finished-----"
puts "Result:\t\t#{end_time - start_time} seconds"
puts " The run times for the iterations from quickest to longest: "
print $time_array.sort
end
end
SimpleBenchmarker.go do
time = rand(0.1..1.0).round(5)
$time_array.push(time)
sleep time
end
4 Answers
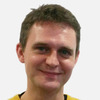
Joel Brennan
18,300 PointsHi Richard
I am just starting Ruby foundations for a couple of weeks now. Your code was really helpful to me when trying the extra credit for the 'blocks' section. Thank you.
I had it in my mind that I wanted to do something a little different (maybe I misunderstood the question).
I wanted to have the bench marking test perform a number of times then display the results for each test.
I had trouble with variables inside/ outside the class (I'm not quite understanding that part), when I learn more I will come back to that. If anyone could help with the correct way to acheive this, I would appreciate any advice.
I finally got something that resembles what I was hoping for, although I'm pretty sure it's not the right way to go about it (I had fun trying...anyways) this was my code for the assignment:
class SimpleBenchmarker
def self.go(how_many=i, &block)
puts "-----------------------------Benchmarking started----------------------------------"
start_time = Time.now
puts "Start Time:\t#{start_time}\n\n"
how_many.times do |a|
print "."
block.call
end
print "\n\n"
end_time = Time.now
puts "End Time:\t#{end_time}\n"
puts "-----------------------------Benchmarking finished---------------------------------"
puts "Result:\t\t#{end_time - start_time} seconds"
total = end_time - start_time
puts "Total = #{total}"
$results.push total
end
end
$results = Array.new
def run_test
puts "The running results are: #{$results.sort}"
puts "How many iterations would you like to run this test for? (0 or blank to exit): "
i = 0
i = gets.chomp.to_i
SimpleBenchmarker.go i do
time = rand(0.1..1.0)
sleep time
end
if i > 0
run_test
else
return nil
end
end
run_test
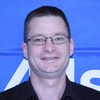
Seth Reece
32,867 PointsThank you Richard Luick and Joel Brennan. The .round and .to_i helped me. I then played around with it. I wanted to pretty up the output, and clean it up a bit. I also wanted to add an "s" to the end of each iteration time, and not display it as an array. It might be a worthless endeavor, but I am stubborn. Anyways:
class SimpleBenchmarker
def self.go(how_many=1, &block)
$sorted_time = Array.new
puts "\n--------------Benchmarking started----------------"
start_time = Time.now
puts "Start Time:\t#{start_time}\n\n"
how_many.times do |a|
print "."
block.call
end
print "\n\n"
end_time = Time.now
puts "End Time:\t#{end_time}\n"
puts "-------------Benchmarking finished----------------\n\n"
result_time = end_time - start_time
puts "Total time:\t\t#{result_time.round(3)} seconds\n\n"
puts "The run times for the iterations from shortest to longest was: #{$sorted_time.sort.join("s, ")}s\n\n"
end
end
print "How many times? "
t = gets.to_i
SimpleBenchmarker.go t do
time = rand(0.1..1.0).round(3)
$sorted_time.push(time)
sleep time
end
Results in:
How many times? 5
--------------Benchmarking started----------------
Start Time: 2014-10-30 18:36:13 -0400
.....
End Time: 2014-10-30 18:36:15 -0400
-------------Benchmarking finished----------------
Total time: 2.039 seconds
The run times for the iterations from shortest to longest was: 0.151s, 0.291s, 0.297s, 0.4s, 0.897s
Feedback would be cool as I don't know if I reached this result in the best way.

David Jones
1,635 PointsRichard Luick - does yours work? I get this:
PS C:\Users\David\treehouse\projects\ruby\source\blocks> ruby benchmarker_extra.rb How many times should we run the program: 3 -----Benchmarking Started----- Start Time: 2014-09-21 20:11:25 -0700
...
End Time: 2014-09-21 20:11:27 -0700 -----Benchmarking Finished----- Result: 1.669095 seconds The run times for the iterations from quickest to longest: [0.23917, 0.51652, 0.90964]PS C:\Users\David\treehouse\projects\ruby\source\blocks>
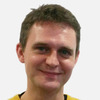
Joel Brennan
18,300 PointsHi Richard,
Thanks, I hope you are well.
I think mine (kind of) works, but in a different way. ie: the total result of the benchamarking test is stored in an array. If I then chose to run a further test that is pushed also into the same array. If I run a third test that total is pushed in and so on.
I'm pretty sure my code is messy and not right on this, but I kind of got it working and was looking for advice to make it right.
The array is sorted quickest to longest.
I thought at first glance your method was sorting each iteration in a single test, where as I wanted to sort the total time of each test until the user exited the program. I think your method is right. (I just had an idea and I wanted to try something different).
*I Just looked at the question again. "Try expanding upon that by asking the user how many iterations they would like to run and then sorting the output from the quickest to slowest finding."
Your method is correct. Mine is sorting the total of each test, so therefore wrong. Sorry :)
Cheers
Joel