Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial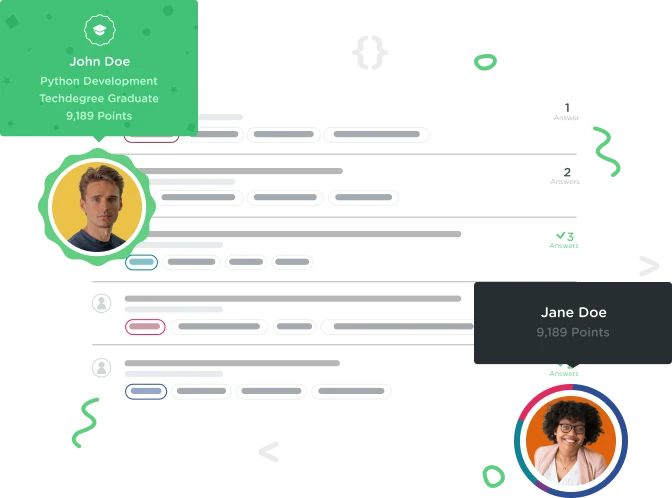

Sara Brothers
7,341 Pointsruby Booleans Challenge 1 of 1
Why am I getting an error?
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item == name
found = true
end
end
if found
return index
else
return nil
end
end
1 Answer

Chris Adamson
132,143 PointsTo accomplish this challenge you need to move the if found check inside the method, compare todo_item.name against name, increment the index each time the item isn't found, and when it is found exit out of the loop (to keep the index from incrementing again):
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
break
else
index += 1
end
end
return found ? index : nil
end