Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial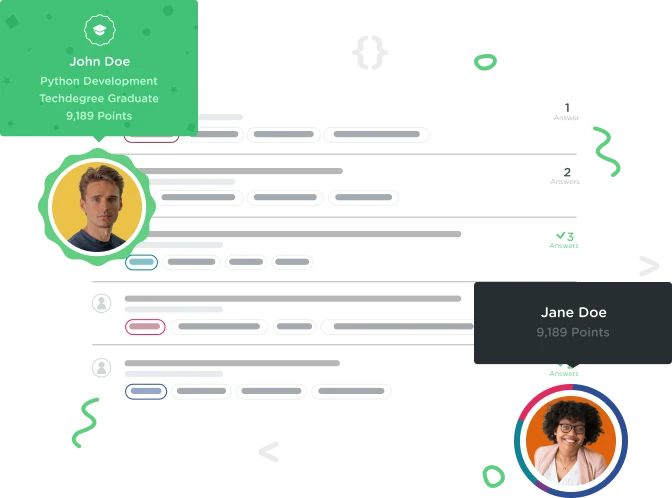
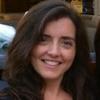
dotz
6,733 PointsRuby Docs each_line basic question
This is the example on Ruby Docs:
print "Example one\n"
"hello\nworld".each_line {|s| p s}
print "Example two\n"
"hello\nworld".each_line('l') {|s| p s}
print "Example three\n"
"hello\n\n\nworld".each_line('') {|s| p s}
produces:
Example one
"hello\n"
"world"
Example two
"hel"
"l"
"o\nworl"
"d"
Example three
"hello\n\n\n"
"world"
{|s| p s}.... **What does p mean? print?
I know its a basic one but it was not something that produced results when googled and Jason's example differs.
Thank you.
OK this isn't easy to read, here is the link to Ruby docs each_line http://ruby-doc.org/core-1.9.3/String.html#method-i-each_line
3 Answers

Charles Smith
7,575 Pointsedit: moved to answer section, oops.
Let's look at example 1:
"hello\nworld".each_line {|s| p s}
What's happening is that it's looking for a line in that string, passing each line into the codeblock as a variable ('s', in this case) and then acting on that variable by printing it. Actually, in this case, it's not printing, it's using p, which is puts.inspect. This is why you still see the \n char in the output.
So it reads "hello\n", passes into the block that prints it, sees the new line created by the newline character, and then reads world (which is it's own line) and passes into the block.
Example 2 and 3 are much the same, except this time you've supplied an operator to split the string at. Example 2 splits at the first and second 'L' in Hello, while the third splits at the empty string '', effectively splitting anytime there is a line break.
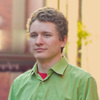
Gene Osborne
8,630 PointsI believe that "p" works like "puts" except that it displays more information about what is being displayed. For example, you could set number = 1 and a_string = "1". Using just "puts" with either of them would display the same thing: '1'. On the other hand, using "p" would result in '1' for number and '"1"' for a_string. Notice the quotes are printed with the string when "p" is used.
So, if you replaced the "p" in the example from Ruby docs with "puts", it would still run, but the words would not be in quotes and the '\n' characters (which are traditionally invisible characters) would not show up.
{|s| puts s} is simply saying for each substring denoted as 's' , 'puts s'
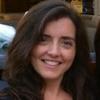
dotz
6,733 Pointsaha! p is puts.inspect! Thank you!
David Clausen
11,403 PointsDavid Clausen
11,403 PointsClarification,
p is puts with inspect being called on the object that it receives.
puts object.inspect vs puts object. p = puts object.inspect
puts.inspect object would throw an error so p =/= puts.inspect
other-words p is just a function that adds inspect like so:
If you made this method you can call it the same way