Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial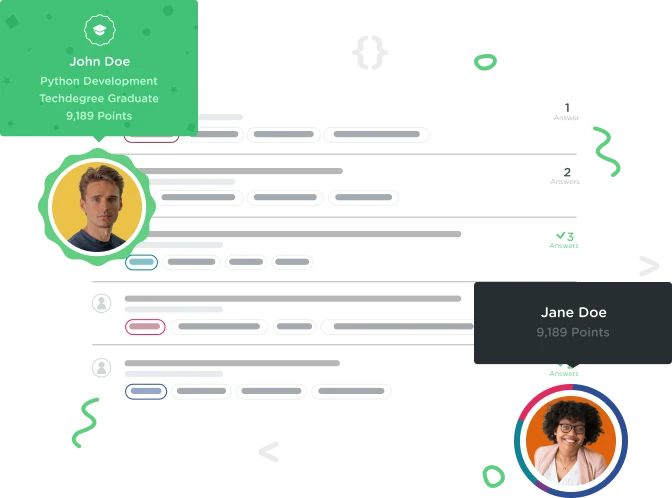

Erik Nuber
20,629 PointsRuby - Grocery List adding in lots more code (just sharing)
Trying to use as much as has been learned up until this point. Just sharing at how I elaborated on the lesson with more interactivity
Here we have code that was created during the lesson, I commented anyway
def create_list #create a new list giving it both a name and making empty array for items
print "What is the list name?"
name = gets.chomp
hash = {"name" => name, "items" => Array.new}
return hash
end
def add_list_item # add both item and quantity of that item to the list
print "What is the item called? "
item_name = gets.chomp
print "How many do you want? "
quantity = gets.chomp.to_i
hash = {"name" => item_name, "quantity" => quantity}
return hash
end
def lineSeperator(character="-") # create seperation on the list to make it easier to read
puts character * 80
end
def print_list(list) # print out the final list of items
puts "List: #{list['name']}"
lineSeperator()
list["items"].each do |item|
puts "\tItem: " + item['name'] + "\t\t\t" +
"Quantity: " + item['quantity'].to_s
lineSeperator()
end
end
This is where I deviated from the lesson. Rather than just add a couple items to the list, I figured this gives an option to have as many items added to the list as the user would like. I created the variable moreItems as an empty string and use it to store a Yes or No value there after. The Until/Do forces a user to either answer yes or no as it is tested for.
def addItemsToList # find out if more items should be added to the list
moreItems = "" #create an empty variable
#create a loop to verify a Yes or NO answer has been made
until (moreItems == "Y" || moreItems == "YES" || moreItems == "N" || moreItems == "NO") do
puts "Do you want to add an item to your list? (Y/N)"
moreItems = gets.upcase.chomp
end
return moreItems #returns a Yes or No
end
Here I created a couple methods to respond to whether the user wants to see the list they created or not. For outputList, you have to pass in the list that was created so that the print_list function can then use it. The noOutputList just gives a formal response if the user doesn't want to see the list.
def outputList(list)
puts "Here is your list: \n"
print_list(list)
end
def noOutputList
puts "Okay well maybe later. Have a beautiful time."
end
I created the variable here for moreItems that finds out if items should be added to the list as a while/do loop has a condition that must be met; you have to set up that condition outside of the loop. This way, the user could choose not to add an item to the list at all. The While/Do loop repeats the same code over and over while the user wants to add an item to the list responding "yes" to that choice, a "no" will exit the loop.
list = create_list #call to create the list
moreItems = addItemsToList #call for first time to see if items should be added in
while (moreItems == "Y" || moreItems == "YES") do #create a loop to add items onto list
list["items"].push(add_list_item())
moreItems = addItemsToList
end
Here I find out if the user wants to see the list or not. I could have once again added in a check for a yes or no response but, used a case statement instead which has a response for that as well.
puts "Do you want to see your shopping list? (Y/N)"
answer = gets.upcase.chomp
case answer
when "Y"
outputList(list)
when "YES"
outputList(list)
when "N"
noOutputList
when "NO"
noOutputList
else
puts "Uh OH, you didn't say yes or no."
end
zanslitinskis
2,049 Pointszanslitinskis
2,049 PointsDone something same, but add without creating def.Also added calculation of items in cart