Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial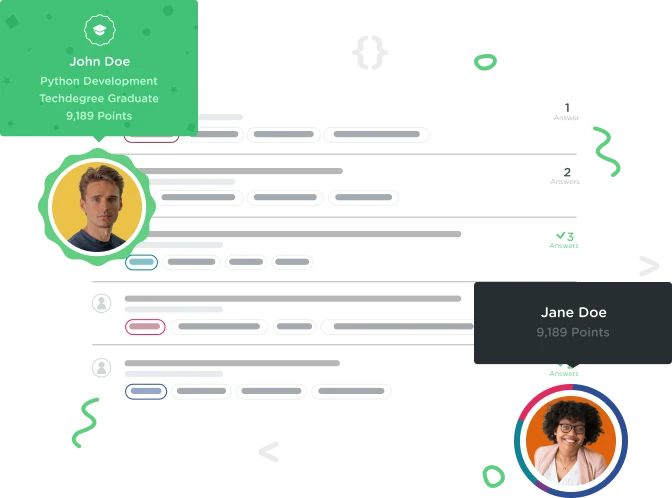
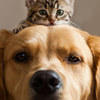
Devin Scheu
66,191 PointsRuby Help
Question: Now that the add_transaction method exists, make the add_transaction method append a hash to the @transactions array. The hash should have the keys description and amount, as symbols, and values that match the arguments to the method.
Code:
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
end
def add_transaction(description, amount)
@transactions.append({"description" => description, "amount" => amount})
end
end
2 Answers

Clayton Perszyk
Treehouse Moderator 48,862 Pointshi Devin
First you need to change the hash in the add_transaction method so that the keys are symbols, not strings:
{:description => description, :amount => amount}
Also, I looked at the ruby docs and couldn't find an append method for Array, so you'll need to use the push method or the << operator:
@transactions.push({:description => description, :amount => amount})
or
@transactions << {:description => description, :amount => amount}

Tiru Otilia
2,274 Pointsclass BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
end
def add_transaction(description,amount)
@transactions.push(description: description, amount: amount)
end
end
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsThanks