Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial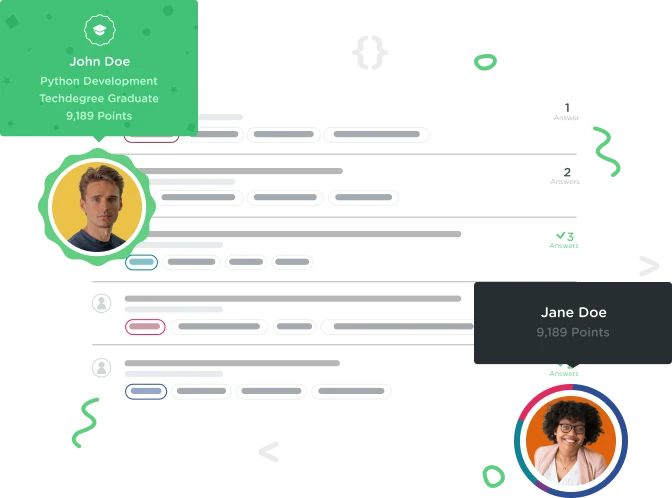
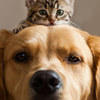
Devin Scheu
66,191 PointsRuby Help
Question: In the BankAccount class, implement a method called balance. The balance method should loop through transactions and calculate the current account balance.
Code:
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
add_transaction("Beginning Balance", 0)
end
def balance
x = 0
loop do
balance += transaction[x]
x +=1
end
return balance
end
def debit(description, amount)
add_transaction(description, -amount)
end
def credit(description, amount)
add_transaction(description, amount)
end
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
end
3 Answers

Clayton Perszyk
Treehouse Moderator 48,837 PointsHi Devin
I can spot a few reasons why your code isn't working:
First, you need to declare a variable named balance, and assign it an initial float value:
balance = 0.0
You should be using the each method on the the @transactions array, instead of a loop and iterator. Inside the block for the each method you receive each transaction in turn as a variable (below I've used transaction; each transaction is a hash with a :description and :amount); you can then add the value of each transaction's amount to the balance by using the key, :amount:
@transactions.each do |transaction|
balance += transaction[:amount]
end
Then you just have to return the balance, like you're already doing.

Anterrio Howard
2,625 PointsThis worked:
def balance balance = 0.0 @transactions.each do |transaction| balance += transaction[:amount] end return balance end

Jeremy Hurtt
2,940 PointsI got this challenge right on the first try, since we had just gone over doing this in the example right before...I was able to remember it easily because I've spent most of the last lesson trying to figure something out about the method this challenge is focusing on...
How does the program understand the variable transaction in this method? Throughout the rest of the code in the program, transaction is never defined or referenced....transactions with an 's' is, as is add_transaction, but not transaction. So when the method iterates through the array, how does the program know what it is adding to the balance with each Did I miss something?
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsThis does not seem to be working for me, here is my edited code:
Clayton Perszyk
Treehouse Moderator 48,837 PointsClayton Perszyk
Treehouse Moderator 48,837 PointsYou still want the method definition you had in your original code:
Also, you want to move return balance outside of the each block and place it before the close of your method definition.
Clayton Perszyk
Treehouse Moderator 48,837 PointsClayton Perszyk
Treehouse Moderator 48,837 PointsHere's what I came up with to pass the challenge: