Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial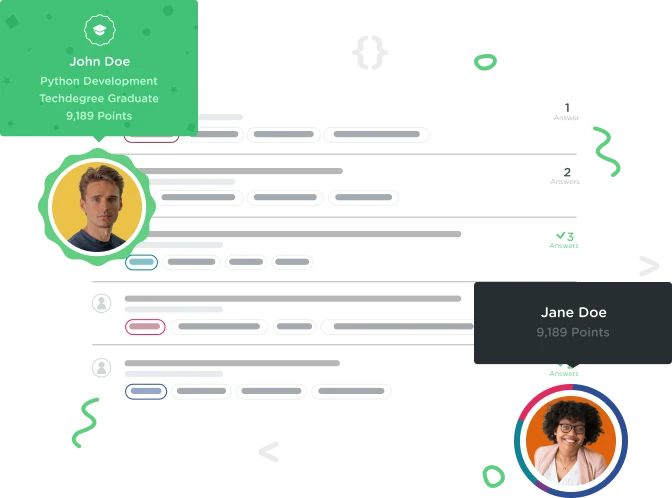

Kevin Miner
Courses Plus Student 2,831 PointsRuby Objects and Classes extra credit: using the inject method.
The objective of the extra credit is to use the inject method to calculate the balance of the bank_account instance of the BankAccount class. I am a little fuzzy on how inject works but I feel worse about not knowing how to pass/specify the hash values of the transaction array into the injection method.
The original balance method works and has been commented out in favor of the ill-fated attempt at a balance method that calculates the balance using inject.
Would appreciate someone taking a look! Thanks in advance!
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
add_transaction("Beginning Balance", 0)
end
def balance
balance = 0
@transactions.each do |transaction|
balance += transaction[:amount]
end
balance
end
def debit(description, amount)
add_transaction(description, -amount)
end
def credit(description, amount)
add_transaction(description, amount)
end
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
end
1 Answer
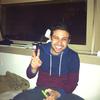
Avram Stern
12,604 PointsHey Kevin Miner give this a try
def balance
@transactions.inject(0.0) { |balance, transaction| balance + transaction[:amount] }
end
The inject method is kind of difficult to understand, but basically what it does is that it takes a collection and reduce it to a single value. Going over the ruby documentantion of the inject method might confuse you as it did with me. So just look for examples and go from there.
Hope this works!