Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial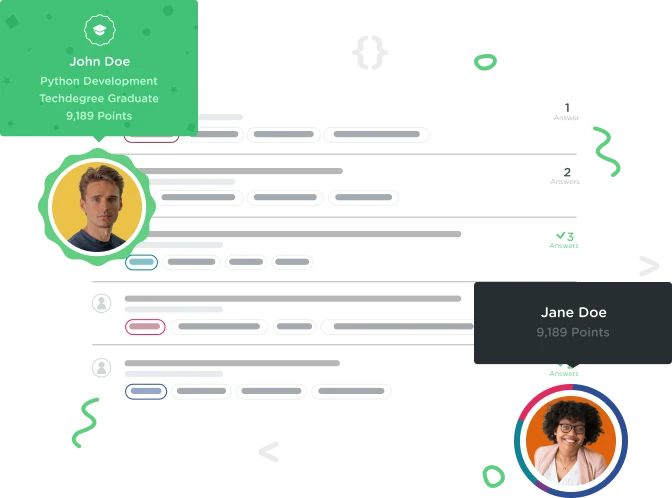
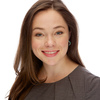
Jacqueline Boltik
Courses Plus Student 11,153 PointsRuby on Rails - How to switch images onMouseover while keeping path
I'm trying to have the image change from a closed box (boxexperiment.jpg) to an open box onMouseover (boxexperiment2.jpg). There are two things I'm having trouble figuring out:
1) The first image disappears onMouseover, but the second image does not render. You can see the page here: https://stormy-atoll-5906.herokuapp.com/shop
If you hover over the Snow Days box you can see what I'm talking about. I'm not trying to do an animation, just switching one image for another.
Here's the code:
<a><%= link_to image_tag("boxexperiment.jpg", onMouseover: 'this.src="/assets/images/boxexperiment2.jpg"'), snowdays_path, id:"logo" %></a>
I suspect my path to the second image is not correct but I haven't been able to find anything else online or figure it out on my own.
2) I'd like to keep the same link for the second image. So if you click on the image after hovering it takes you to the correct page (in my case snowdays_path).
Appreciate any help!
5 Answers
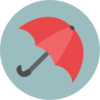
Nathan Brown
229 PointsJacqueline,
Unfortunately I believe mouseover was deprecated in Rails 4. The problem you are having is with 'this.src' in
onmouseover="this.src="/app/assets/images/boxexperiment2.jpg""
'this.src' is referring to /shop/app/assets/images/boxexperiment2.jpg instead of your asset pipeline.
I think the best way to handle this is with some easy jQuery in your app/assets/javascript file. In your view make two divs, something like this:
<div class="closed-box"><%= link_to image_tag("boxexperiment.jpg"), snowdays_path %></div>
<div class="open-box"><%= link_to image_tag("boxexperiment2.jpg"), snowdays_path %></div>
You might want to consider renaming your jpegs semantically as "open_box.jpg" and "closed_box.jpg". It will make it easier for you or your employees to know what these are when you return to your code in the years ahead.
In your app/assets/stylesheets add this:
.open-box {
display: none;
}
Then write some jQuery like so:
$(document).ready(function() {
$(".closed-box").mouseenter(function() {
$(".open-box").show();
$(".closed-box").hide();
$(this).hide();
});
$(".open-box").mouseout(function() {
$(".closed-box").show();
$(".open-box").hide();
$(this).hide();
});
});
Let me know if you have any questions or encounter any problems trying to implement this.
Nathan
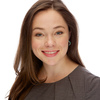
Jacqueline Boltik
Courses Plus Student 11,153 PointsNathan,
Thank you so much! Everything seemed to work :) One last question. The image only changed on hover after I refresh the page (this happens both locally and in production).
Should I go ahead and put the jQuery in the HTML? Right now my javascript is in the head of application.html.erb so it should be loading before the page. I went ahead and changed it to coffeescript (what I'm using) in my javascript file.
https://stormy-atoll-5906.herokuapp.com/shop
Maciej - appreciate you editing my post to make it readable.
Thank you both for your help - I had been stuck on this for awhile.
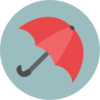
Nathan Brown
229 PointsI am fairly sure that this is a turbolinks problem. There are two solutions.
The easy solution is just to delete //= require turbolinks
from the javascript manifest file. You will find that at app/assets/javascripts/application.js.
The other solution I'm aware of would be to add the jquery-turbolinks gem. jquery-turbolinks
Add the gem to your Gemfile:
gem "jquery-turbolinks"
and bundle install
Then add //= require jquery.turbolinks
to the javascript manifest file(referenced above). Make sure it is below //= require jquery
and above //= require turbolinks
.
I wouldn't recommend putting your JavaScript in the body for a rails app.
I'm rather confident that either of these will work for you. Let me know how things go or if you have questions.
Nathan
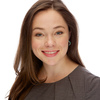
Jacqueline Boltik
Courses Plus Student 11,153 PointsNathan - thanks for your response. I think you're right about it being a turbolinks issue but unfortunately the issue hasn't been resolved.
I tried both solutions but it still doesn't seem to be loading properly the first time. After adding the jquery-turbolinks gem it doesn't switch images locally, but it does after refreshing the page (exactly like before) in production.
Let me know if you can think of anything else to try. If not you've been really helpful already and I'll continue searching around on stackoverflow ;)
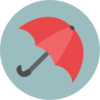
Nathan Brown
229 PointsJacqueline,
I will put on my thinking cap...
May I ask a question? Where are you writing the jQuery? Is it in the application.html.erb view or did you include it in a folder inside app/assets/javascripts/?
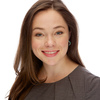
Jacqueline Boltik
Courses Plus Student 11,153 PointsAppreciate it, just knowing the issue is with Turbolinks helps narrow my search. I just watched the Railscast on Turbolinks which was informative but still no solution.
I put the jQuery you provided in app/assets/javascripts. But first I converted the code you suggested to coffee script so it would run on my application.
Thanks again for your help!
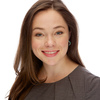
Jacqueline Boltik
Courses Plus Student 11,153 PointsHere's what ended up working. I changed
$(document).ready(function()
to $(document).on "page:change", ->
The markup is slightly different on mine since I converted to coffee.
After making that small change everything worked nicely both locally and in production (after running rake assets:precompile of course first). If you're having this same issue you can read more about what's happening and how to fix it here http://guides.rubyonrails.org/working_with_javascript_in_rails.html
Big thanks to Nathan in alerting me that this was a turblolinks issue :)
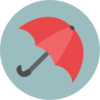
Nathan Brown
229 PointsJacqueline,
Good work hunting down that solution and thanks for posting that answer. I'm not very familiar with CoffeeScript but I read section 5.2 Page Change Events and I learned a bit. Great work on your site as well. It’s nice to see that box behaving like it should.
Nathan
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsI edited your post to show the code (it was hidden). I agree with Nathan, jQuery is the best way to handle these things.