Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial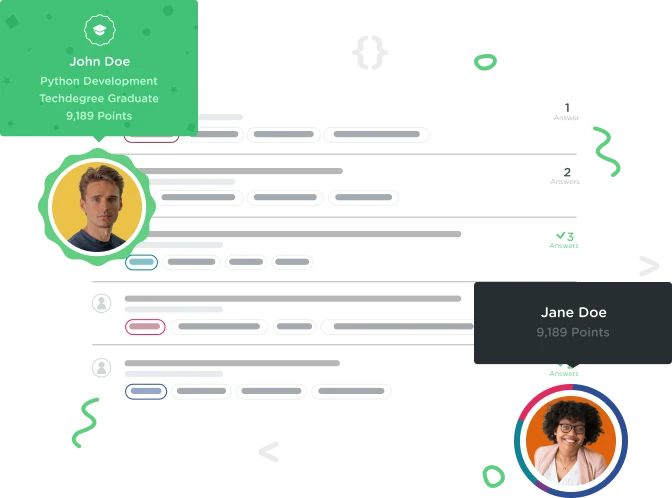

luke brusseau
2,268 PointsRuby operators and control structures &&
I am a bit confused on what im doing wrong
def valid_command?(command)
if command = ("y") || ("yes") || ("Y") || ("YES") ||
return "true"
end
end
2 Answers
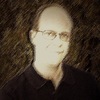
Jason Anders
Treehouse Moderator 145,860 PointsHi Luke,
You are on the right track and very close. First you have an extra ||
at the end on the conditional. Second, so Ruby knows that all those values are being evaluated as one condition, you just need to wrap them all in a set of parenthesis.
def valid_command?(command)
if command = (("y") || ("yes") || ("Y") || ("YES"))
return "true"
end
end
Keep Coding! :)
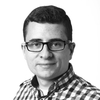
Tomasz Jaśkiewicz
5,399 PointsHi, Luke!
I assume that you want to check if command belongs to this list: "y", "yes", "Y", "YES"
.
If you want to check whether command is valid you can do smth like this:
def valid_command?(command)
command_list = %w(y yes)
command_list.include?(command.downcase)
end
command_list = %w(y yes)
This line will create an array of strings: ['y', 'yes']
command.downcase
downcase
method will return string in lowercase: "YEs".downcase -> "yes"
command_list.include?(command.downcase)
include?
method invoked on array (command_list) will return true
if our array (['y', 'yes']
) includes our string (eg. 'y'
) or false
if not. Our valid_command?
method will return finally the same value as last line defined in our method. We do not need a return
statement here.
What's wrong about your code is that you can't leave ||
at the end of line because Ruby waits for next argument then.
Beware of using =
and ==
operators. The first one is an assignment operator and it won't compare anything. ==
is a operator you're looking for.
Keep going! Ask questions and enjoy! Good job! :)
Sources:
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsDo this instead:
and yes, do this:
return "true"
into
return true