Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial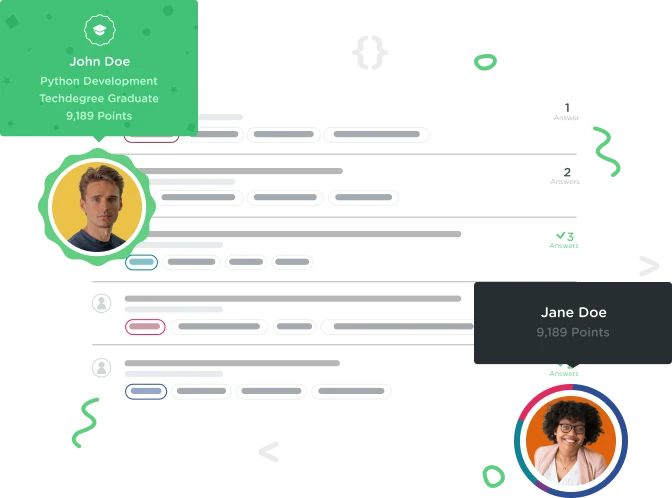

Jason Chow
4,428 PointsRuby Operators and Control Structures (valid_command?)
def valid_command?(command) if (command = gets.chomp.downcase) && (command = gets.chomp.upcase) return true end end
please help.
def valid_command?(command)
if (command = gets.chomp.downcase) && (command = gets.chomp.upcase)
return true
end
end
2 Answers

Galen Cook
2,463 PointsYou didn't really ask a question, but I believe your problem is that you are using the single equals sign (which sets a variable's value) rather than the double equals sign (which compares two values).
Replace your =
with ==
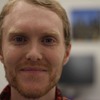
david carter
9,677 Pointsyeah, without a question it's not that clear what you need. here are some things i see about the code:
- right now, it will always return true, no matter what you supply as an argument. The reason is that both statements
command = gets.chomp.downcase
and
command = gets.chomp.upcase
are going to be coerced into truthy statements. and truthy && truthy is true in ruby. even if you don't provide any input and just hit Enter when prompted for input (once for each gets.chomp
call), the statement:
"" && ""
evaluates to
""
which is truthy so it will return true. You're basically just reassigning the variable command
twice to whatever comes in through std.in..
It's not clear what a valid command is. even if you replace the single equals with double equals, I can only see the statement evaluating to true if there are no letters involved.. just numbers or punctuation.. how could any string downcased be equivalent to the same string upcased? are commands only valid if they are non-alphabetic?
Lastly, you should have either an
else return false
or put a false statement at the end of the method so that the method always will, as the question mark implies, evaluate to a boolean value.
Hope that helps
- If i knew what you were trying to accomplish with this code, I might be able to provide more constructive feedback.
IF valid commands are only those that return
true
when the command.upcase is equal to the command.downcase, the following code will work:
def valid_command?
cmd = gets.chomp
cmd.downcase == cmd.upcase
end