Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial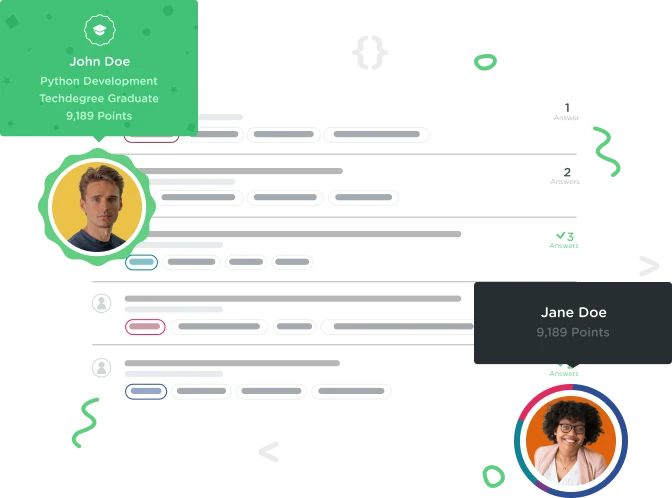
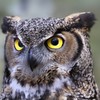
avremel
8,448 PointsRuby operators code challenge
<h1>This is the challeng: </h1> Modify the "valid_command?" method to return true when passed the following values: "y", "yes", "Y", or "YES".
Here is what I tried:
def valid_command?(command) if (command == "y") || (command == "yes") || (command == "Y") || (command == "YES") valid_command? = true end end
def valid_command?(command)
if (command == "y") || (command == "yes") || (command == "Y") || (command == "YES")
valid_command? = true
end
end
Why is this not working?
5 Answers
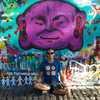
Andrew Stelmach
12,583 PointsYou got most of it right. I don't have the depth of knowledge to guide you towards an answer, but I know what it should be:
def valid_command?(command)
if (command == "y") || (command == "yes") || (command == "Y") || (command == "YES")
return true
end
end
Personally, I don't know why you can't just say 'return' instead of 'return true', because I would have thought that 'return' would return 'true' i.e. the method would evaluate to true.
Maybe someone can enlighten us.
EDIT: the code is 'DRY-ist' as:
def valid_command?(command)
command == "y" || "yes" || "Y" || "YES"
end
Personally, this is how I would write it - it's readable enough for most developers.
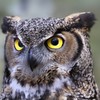
avremel
8,448 PointsThanks for the quick response
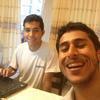
Wilfredo Casas
6,174 Pointsare these '||' the same as &&?
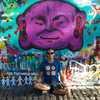
Andrew Stelmach
12,583 PointsWilfredo Casas , no; it means 'or'.
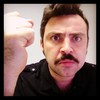
codebully
2,306 PointsYou can DRY this up with as well:
def valid_command?(command)
if (command.downcase == "y") || (command.downcase == "yes")
return true
end
end
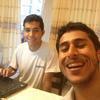
Wilfredo Casas
6,174 Pointsare these '||' the same as &&?

adrian silva
4,959 Pointsi used the case and when condition but that didnt work i just want to know why or is it just how it is ? def valid_command?(command) case command when command == "y" return true when command == "yes" return true when command == "Y" return true when command == "YES" return true end end

Saloni Tulsiyan
Front End Web Development Techdegree Student 971 Pointsdef valid_command?(command) if (command == "y") || (command == "yes") || (command == "Y") || (command== "YES") return "true" end end

Sandra Hogan
8,657 PointsWilfredo Casas '||' and '&&' are 2 different symbols. The pipes( || ) mean 'or' and the '&&' mean 'and'
Bisrat Teshome
1,028 PointsBisrat Teshome
1,028 PointsRemoving the parenthesis (since you already used double quotes) will solve the problem.
def valid_command? (command)
if command == "y" || command == "yes" || command == "Y" || command == "YES"
return "true"
end
end