Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial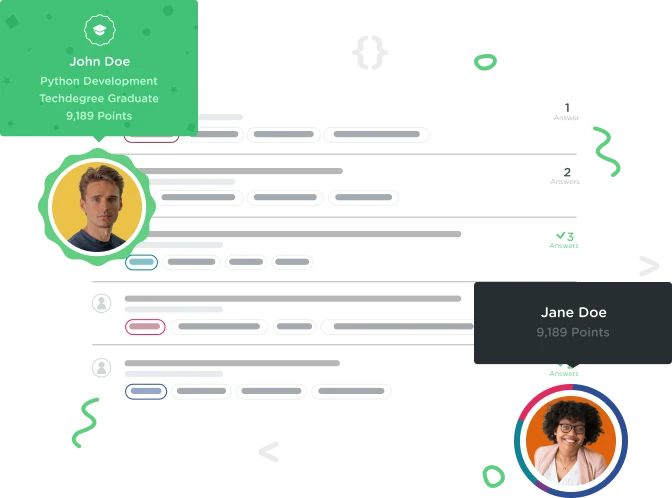

Peter Xu
3,553 PointsRunning 'node app.js' hangs after response is returned. Why is this happening?
I've done the example and have the code almost identical to the video tutorial.
var username = "peterxu2";
var http = require("http");
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
console.log(response.statusCode);
});
And my output looks like:
treehouse:~/workspace$ node app.js
200
Notice the command prompt doesn't display. It takes about 10 or more seconds before the prompt returns:
treehouse:~/workspace$ node app.js
200
treehouse:~/workspace$
Why does the request hang for so long? It seemed to return almost immediately in the video. I'm behind a corporate proxy now, could that be having any impact?
4 Answers
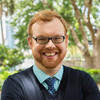
Andrew Chalkley
Treehouse Guest TeacherI think what's happening in your code is that "end" hasn't been implemented yet and the Node.js process needs to Timeout. The request has already been responded to because of the 200
. If it hadn't it would look like this...
treehouse:~/workspace$ node app.js
...for longer.
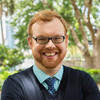
Andrew Chalkley
Treehouse Guest TeacherHey Peter
Check out the comments in the code. It now works.
Regards
Andrew
var username = "peterxu2";
var http = require("http");
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
var statcode;
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
//console.log(response.statusCode
statcode = response.statusCode;
//You need to implement 'data' for the 'end' to get triggered eventually.
response.on("data", function(){})
//You need to implement the response's end not the requests 'end'.
//And it needs to be inside the callback so you can access the response.
response.on("end", function() {
console.log("Status Code: " + statcode);
});
});

Kevin VanConant
1,833 PointsIt very well could. I'm behind a corp network as well and it can be laggy. At home fast as the videos no problem.

Peter Xu
3,553 PointsI don't think that's the issue.
var username = "peterxu2";
var http = require("http");
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
var statcode;
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
//console.log(response.statusCode
statcode = response.statusCode;
});
request.on("end", function() {
console.log("Status Code: " + statcode);
});
I implemented the "end" event, but it doesn't seem to be triggered.
treehouse:~/workspace$ node app.js
The response is being printed in get, but it doesn't seem to reach "end" for some reason.