Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial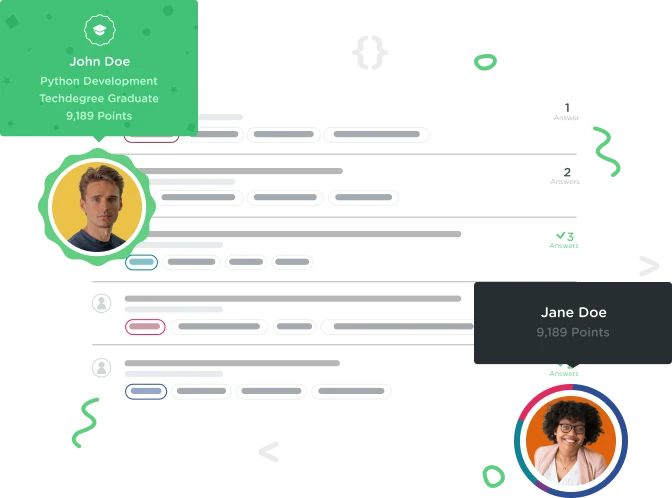
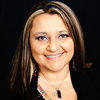
Milagro Scardigno
4,494 PointsRunning this in localhost, and keep getting a POST 404 (Not found)
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with jQuery</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script>
// var url = "http://website.com/posts";
// var data = {
// firstName : "Dave",
// lastName : "McFarland"
// };
// $.post(url,data,callback);
$(document).ready(function () {
// body...
//now we need to add an event handler that is when the visitor clicks the signup button will send out our AJAX request
$('form').submit(function(event) {
/* Act on the event */
event.preventDefault();//prevents the code from submitting, when the submit button is clicked
//HTML form have an action property, so we can set our url simply to the action property of the form
var url = $(this).attr("action"); //here we are using jquery to select the form and pull out its action attribute $(this) the url to server side program .attr("action") that is gonna process this form
//next
//lets capture the form data
//using plain Javascript you will have to generate a list of every form field, then go through that list, retrieve the name and the value for each form field and then encode all that
//fortunately jquery provides a simpler form: just select the form and run its serialize() method on it
var formData = $(this).serialize(); // now we have our url and data, now we need to run the post method
$.post(url, formData, function (response) {
// body...
//let the user know the form was submitted
//since we dont need the form or form fields on the page any longer, lets replace them
//this code select a div on the page and replace it with a message
$('#signup').html("<p>Thanks for signing up!</p>");
});//end of post
});
}); //end ready
/////OLD CODE//////
// $(document).ready(function() {
// $('form').submit(function(evt) {
// evt.preventDefault();
// var url = $(this).attr("action");
// var formData = $(this).serialize();
// //supports the ability to send more data meant to be stored in a database
// $.post(url, formData, function(response) {
// $('#signup').html("<p>Thanks for signing up!</p>");
// }); // end post
// }); // end submit
// }); // end ready
//////END OF OLD CODE///////
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Sign Up for Our Newsletter</h1>
<div id="signup">
<form method="post" action="/signup">
<label for="userName">Please type your name</label>
<input type="text" name="userName" id="userName"><br>
<label for="email">Your email address</label>
<input type="email" name="email" id="email"><br>
<label for="submit"></label>
<input type="submit" value="Signup!" id="submit">
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Any suggestions on what I am doing wrong?
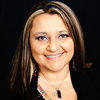
Milagro Scardigno
4,494 PointsI see the error after clicking the submit button. I added alerts on each function block to see if the functions are firing. Please ignore my comments since they are notes to reference later.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with jQuery</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script>
// var url = "http://website.com/posts";
// var data = {
// firstName : "Dave",
// lastName : "McFarland"
// };
// $.post(url,data,callback);
$(document).ready(function() {
// body...
alert('I am ready');
//now we need to add an event handler that is when the visitor clicks the signup button will send out our AJAX request
$('form').submit(function(evt) {
/* Act on the event */
evt.preventDefault();//prevents the code from submitting, when the submit button is clicked
alert('submit event happened');
//HTML form have an action property, so we can set our url simply to the action property of the form
var url = $(this).attr("action"); //here we are using jquery to select the form and pull out its action attribute $(this) the url to server side program .attr("action") that is gonna process this form
//next
//lets capture the form data
//using plain Javascript you will have to generate a list of every form field, then go through that list, retrieve the name and the value for each form field and then encode all that
//fortunately jquery provides a simpler form: just select the form and run its serialize() method on it
var formData = $(this).serialize(); // now we have our url and data, now we need to run the post method
$.post(url, formData, function (response) {
// body...
//let the user know the form was submitted
//since we dont need the form or form fields on the page any longer, lets replace them
//this code select a div on the page and replace it with a message
alert("I am getting to the response text to thank for signing up");
$('#signup').html("<p>Thanks for signing up!</p>");
});//end of post
});
}); //end ready
/////OLD CODE//////
// $(document).ready(function() {
// $('form').submit(function(evt) {
// evt.preventDefault();
// var url = $(this).attr("action");
// var formData = $(this).serialize();
// //supports the ability to send more data meant to be stored in a database
// $.post(url, formData, function(response) {
// $('#signup').html("<p>Thanks for signing up!</p>");
// }); // end post
// }); // end submit
// }); // end ready
//////END OF OLD CODE///////
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Sign Up for Our Newsletter</h1>
<div id="signup">
<form method="post" action="/signup">
<label for="userName">Please type your name</label>
<input type="text" name="userName" id="userName"><br>
<label for="email">Your email address</label>
<input type="email" name="email" id="email"><br>
<label for="submit"></label>
<input type="submit" value="Signup!" id="submit">
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
2 Answers
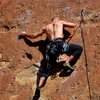
Shawn Flanigan
Courses Plus Student 15,815 PointsYour JavaScript is pulling the action
attribute from your form, with this bit of code:
var url = $(this).attr("action");
and trying to send data to that url with an AJAX request. In this instance, your form has an action of /signup
, which means your JavaScript will try to submit this information to localhost/signup
. In the project files, this page doesn't exist, so it's throwing a 404 error. In production, you'd probably have a directory called signup
, with a php file that would process the data.
Here, if you want to get rid of the 404 error, you could change the action
to /index.html
. It still won't process your form, but you should no longer see this error.
Hope this makes sense!
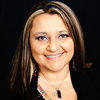
Milagro Scardigno
4,494 PointsNow that works!
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with jQuery</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script>
// // var url = "http://website.com/posts";
// // var data = {
// // firstName : "Dave",
// // lastName : "McFarland"
// // };
// // $.post(url,data,callback);
// $(document).ready(function() {
// // body...
// alert('I am ready');
// //now we need to add an event handler that is when the visitor clicks the signup button will send out our AJAX request
// $('form').submit(function(evt) {
// /* Act on the event */
// evt.preventDefault();//prevents the code from submitting, when the submit button is clicked
// alert('submit event happened');
// //HTML form have an action property, so we can set our url simply to the action property of the form
// var url = $(this).attr("action"); //here we are using jquery to select the form and pull out its action attribute $(this) the url to server side program .attr("action") that is gonna process this form
// //next
// //lets capture the form data
// //using plain Javascript you will have to generate a list of every form field, then go through that list, retrieve the name and the value for each form field and then encode all that
// //fortunately jquery provides a simpler form: just select the form and run its serialize() method on it
// var formData = $(this).serialize(); // now we have our url and data, now we need to run the post method
// $.post(url, formData, function(response) {
// // body...
// //let the user know the form was submitted
// //since we dont need the form or form fields on the page any longer, lets replace them
// //this code select a div on the page and replace it with a message
// alert("I am getting to the response text to thank for signing up");
// $('#signup').html("<p>Thanks for signing up!</p>");
// });//end of post
// });
// }); //end ready
/////OLD CODE//////
$(document).ready(function() {
$('form').submit(function(evt) {
evt.preventDefault();
var url = $(this).attr("action");
var formData = $(this).serialize();
//supports the ability to send more data meant to be stored in a database
$.post(url, formData, function(response) {
$('#signup').html("<p>Thanks for signing up!</p>");
}); // end post
}); // end submit
}); // end ready
//////END OF OLD CODE///////
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Sign Up for Our Newsletter</h1>
<div id="signup">
<form method="post" action="index.html">
<label for="userName">Please type your name</label>
<input type="text" name="userName" id="userName"><br>
<label for="email">Your email address</label>
<input type="email" name="email" id="email"><br>
<label for="submit"></label>
<input type="submit" value="Signup!" id="submit">
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
T H A N K Y O U !!!!!!
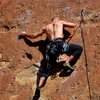
Shawn Flanigan
Courses Plus Student 15,815 PointsNo problem. Glad I could help!
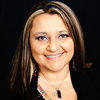
Milagro Scardigno
4,494 PointsAt the end the form in this case is being posted back to the index.html file!
<form method="post" action="index.html">
<label for="userName">Please type your name</label>
<input type="text" name="userName" id="userName"><br>
<label for="email">Your email address</label>
<input type="email" name="email" id="email"><br>
<label for="submit"></label>
<input type="submit" value="Signup!" id="submit">
</form>
Richard Duncan
5,568 PointsRichard Duncan
5,568 PointsHi Milagro, I can't reproduce. Could you tell me when are you seeing the error? is all the code meant to be commented out in your example (posted) or is that as a result of your own debugging efforts?