Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial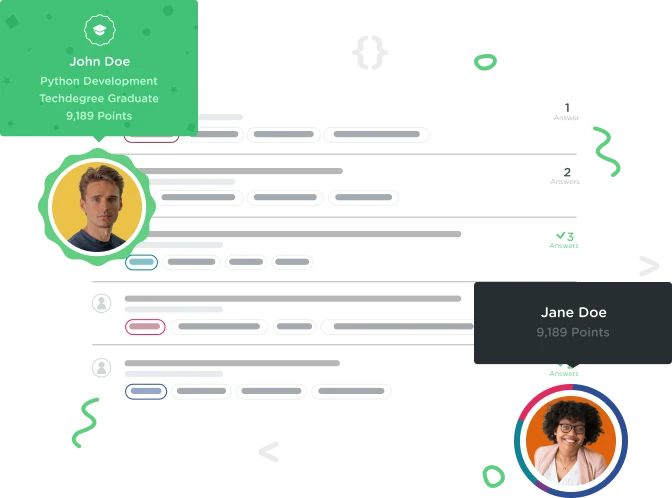

Ben Forrester
Courses Plus Student 5,357 PointsRuns fine in the playground
What's wrong?
// Enter your code below
func getTowerCoordinates(location: String) -> (lat: Double, lon: Double) {
let lat: Double
let lon: Double
switch location {
case "Eiffel Tower": (lat = 48.8582, lon = 2.2945)
case "Great Pyramid": (lat = 29.9792, lon = 31.1344)
case "Sydney Opera House": (lat = 33.8587, lon = 151.2140)
default: (lat = 0,lon = 0)
}
return (lat,lon)
}
3 Answers
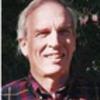
jcorum
71,830 PointsI guess the challenge meant it when it said you didn't have to name the return values. So you need to lose the lat lon:
func getTowerCoordinates(location: String) -> (lat: Double, lon: Double) {
Also, if a function returns two values in a tuple, you don't redeclare those values in the function. So you need to delete these two lines:
let lat: Double
let lon: Double
But the most important part is you need a return statement for each case (and the default):
func getTowerCoordinates(location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower":
return ( 48.8582, 2.2945)
case "Great Pyramid":
return ( 29.9792, 31.1344)
case "Sydney Opera House":
return ( 33.8587, 151.2140)
default:
return ( 0, 0)
}
}
The general rule is one return statement for each possible path through your code. Here there are 4 possible paths, and each needs its own return statement.
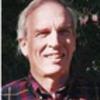
jcorum
71,830 PointsYes, it runs fine in a playground. It's not that you can't do it that way. Ben was asking, or so I thought, why it wouldn't work in the challenge. And, as it happens, if you don't name the return values and you leave out the let statements it works! Admittedly the editor is a bit picky, but if you want to complete the challenge you have to make some "compromises".

Greg Kaleka
39,021 PointsYes, I agree that's what Ben is asking. It works fine in the challenge, though, if you simply remove the names in the tuple. The rest of the code is fine.
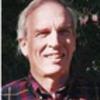
jcorum
71,830 PointsGood point. I didn't try all the possible variations before responding.