Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial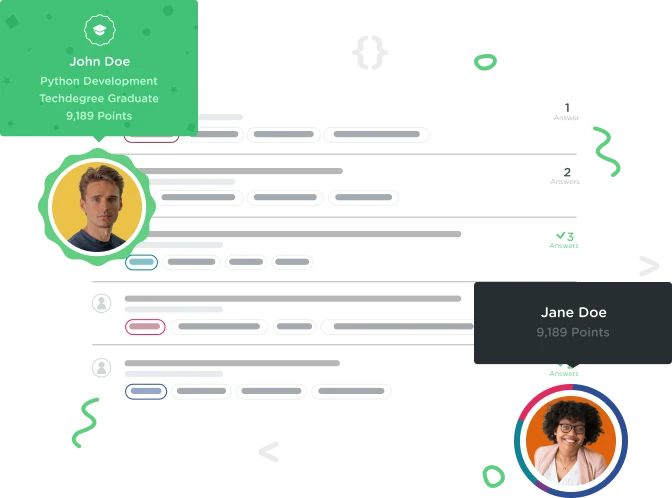

habtezion dawit
1,918 Pointssame error keeps coming up...
my code looks good but for i keep getting the same error
./com/example/Blog.java:30: error: for-each not applicable to expression type for (String category: post.getCategory()) { ^ required: array or java.lang.Iterable found: String Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
and i can't figure out what the problem is...
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String,Integer> getCategoryCounts () {
Map<String,Integer> categoryCounts = new HashMap<String,Integer>();
for (BlogPost post: mPosts) {
for (String category: post.getCategory()) {
Integer count = categoryCounts.get(category);
if(count == null){
count = 0;
}
}
}
}
}
3 Answers

andren
28,558 PointsThe error message is actually pretty descriptive:
"for-each not applicable to expression type for (String category: post.getCategory()) { ^ required: array or java.lang.Iterable found: String"
It is saying that for-each loops only work on lists or classes that are Iterable, but you gave it a string, which are neither of those things.
In other words the problem is that getCategory() returns a category (as the name suggest) not a list of categories, which is what the for-each loop is expecting.
The solution to this problem is pretty simple, since getCategory() just returns a single string you don't need to loop over it, so just remove the for loop and use getCategory() in the places where you are currently using the category variable you define in your for loop.
Your code will still generate errors after making this fix though as your code is incomplete, you need to increment the count, add it to the map, and then return the map. After adding the code to do that you should be able to pass the challenge.

habtezion dawit
1,918 Pointsi tried that Andren and either you are incorrect or i'm not applying your instructions correctly, i'm thinking that its the latter.
i don't want you to give me the answer but i need to understand what exactly i'm doing wrong...
PLEASE HELP!

andren
28,558 PointsWell is there any specific error you are getting? It's difficult to give you much more hints than I already did without literally giving you the answer, when I was testing your code for errors the 3 things I mentioned above were the exact things I added to the code to make it pass the challenge.
The only thing I can do is make my instructions a bit more specific, in your code you check if the count is null and set the count to 0 if it is, that is fine but after doing that you need to increment the count to reflect the fact that an instance of that category has been found, after increasing that counter by 1 you need to add it back to the categoryCounts map, when you put it back you need to specify the category name as the key and the count variable as the value.
Then you need to return the map, though be careful that the return statement is placed outside of your for loop, if it is placed inside the for loop then your method will end before you have actually gone through all of the blog posts.
I won't post the solution code directly in this post since you said you didn't want me to post it, but if you do end up stuck then I have posted the solution for you here, so that you can take a look at it as a last resort.

habtezion dawit
1,918 PointsThanks Andren, i tried it myself several times but i couldn't get it until i had a look at what you posted. i am now able to see where and what mistakes were...
thank you