Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial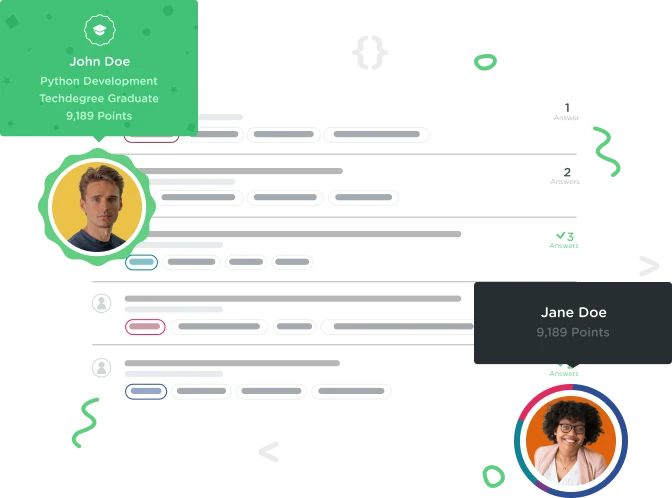

Abubakar Gataev
2,226 PointsSame idea as the last one. My loopy function needs to skip an item this time, though. Loop through each item in items ag
please help me i don't have an idea about this one.
def loopy(items): for item in items: if .index(0) = "a" : continue else: print(item)
3 Answers
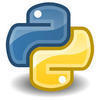
Gerald Wells
12,763 PointsThe challange is asking you to only print items that do not start with 'a'. So an implementation in psuedo code:
for each item in items:
if that item does not start with a:
print the item
Here is an example using something a bit easier to understand than the next:
def loopy(items):
final_lyst = []
for item in items:
if not item.startswith('a'):
final_lyst.append(item)
print(final_lyst)
Here is an example using list comprehension:
def loopy(items):
items = [ item for item in items if not item.startswith('a')]
print(items)

Abubakar Gataev
2,226 PointsI changed my question could you check it again please?
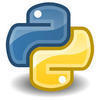
Gerald Wells
12,763 PointsProblem 1
- It would be wise for you to start testing your code in the python emulator, if you have quetions as to why something isn't working there is a built in
help()
function that will give you insight.
# Inside the interpreter
>>> help(list.index)
# From help()
index(...) method of builtins.list instance
L.index(value, [start, [stop]]) -> integer -- return first index of value.
Raises ValueError if the value is not present.
Problem 2
- So a common pitfall I believe every new programmer has is performing unecessary checks that makes there code redundant.
def loopy(items):
for item in items:
# ----- This block -------
if .index(0) = "a" :
continue
# ----- End Block -----
else:
print(item)
From the block of code I have marked above.... what if instead of checking to see if the first index is an 'a', you check to see if it isn't.
When you are finding that the value is an 'a' you are continue
ing ... seems unecessary. Instead look at this.
def loop(items):
for item in items:
if 'a' != item[0]:
print(item)