Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial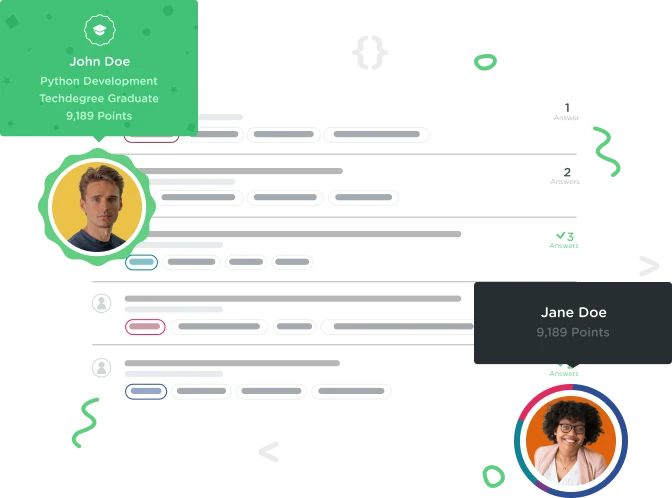
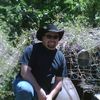
Mike Smith
11,863 PointsSass Question - Multiple Class Names
I am trying to accomplish the following type of CSS:
.posting,
.hero,
.code,
.ical,
.links {
background-color: whitesmoke;
font-family: monospace;
border-radius: 10px;
margin: 5px auto;
padding: 0px 10px;
max-height: 400px;
overflow: auto;
}
I would like to group them instead of adding a definition for each individual class.
Would it make more sense to have multiple classes defined such as:
<div class="box posting"></div>
and then add only the specific changes to the second class identifier? Or, is there a way to accomplish this with Sass? A good example would be the grid.css file used in the Front-End Web Design.
8 Answers
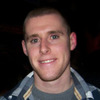
Michael Pare
6,358 PointsYeah, that would work fine now! i still don't see how it benefits you to separate .grid and the column declaration classes. You can get DRY output results if you move your current .grid class into a %extend like below and include it in your @for loop:
%grid-column {
margin: 0 2% 1% 0;
float:left;
display:block;
}
@for $i from 1 through 12 {
.grid_#{$i}, {
width: unquote((8.5 * $i - 2)+"%");
@extend %grid-column;
}
}
.alpha{margin-left:0;}
.omega{margin-right:0;}
which produces
.grid_1, .grid_2, .grid_3, .grid_4, .grid_5, .grid_6, .grid_7, .grid_8, .grid_9, .grid_10, .grid_11, .grid_12 {
margin: 0 2% 1% 0;
float: left;
display: block;
}
.grid_1 {
width: 6.5%;
}
.grid_2 {
width: 15%;
}
.grid_3 {
width: 23.5%;
}
.grid_4 {
width: 32%;
}
.grid_5 {
width: 40.5%;
}
.grid_6 {
width: 49%;
}
.grid_7 {
width: 57.5%;
}
.grid_8 {
width: 66%;
}
.grid_9 {
width: 74.5%;
}
.grid_10 {
width: 83%;
}
.grid_11 {
width: 91.5%;
}
.grid_12 {
width: 100%;
}
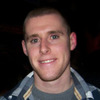
Michael Pare
6,358 PointsAre you saying that you don't want to list off the individual classes .posting, .hero, .code, etc. when they share the same code?
If so, you could do that with sass by using a %extend like this:
%box {
background-color: whitesmoke;
font-family: monospace;
border-radius: 10px;
margin: 5px auto;
padding: 0px 10px;
max-height: 400px;
overflow: auto;
}
Then you can semanitcally name classes in your stylesheet like:
.box posting {
@extend %box; /*extend styles from %box to this class */
/* any additional styles that apply only to .box-posting */
}
More on extends: http://sass-lang.com/documentation/file.SASS_REFERENCE.html#extend

Leonardo Hernandez
13,798 PointsDRY - Don't repeat yourself.
IMO - your second solution of multiple classes is more effective.
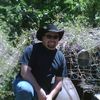
Mike Smith
11,863 PointsThank you for the quick responses. Sometimes just writing it down so others can understand the question helps me answer it. Ultimately, to save coding space and time, I would probably opt for the second solution. My curiosity is to find a way to duplicate the syntax that occurs in the grid.css file that is similar to the first box in my question.
As for clarity and compacting of the code, I think the grid.css would look a lot like this in implementaion:
@for $i from 1 through 12 {
._#{$i}, {
width: unquote((8.5 * $i - 2)+"%");
}
}
.grid {
margin: 0 2% 1% 0;
float: left;
display: block;
}
.alpha{margin-left:0;}
.omega{margin-right:0;}
would produce:
._1 { width: 6.5%; }
._2 { width: 15%; }
._3 { width: 23.5%; }
._4 { width: 32%; }
._5 { width: 40.5%; }
._6 { width: 49%; }
._7 { width: 57.5%; }
._8 { width: 66%; }
._9 { width: 74.5%; }
._10 { width: 83%; }
._11 { width: 91.5%; }
._12 { width: 100%; }
.grid { margin: 0 2% 1% 0; float: left; display: block; }
.alpha{margin-left:0;}
.omega{margin-right:0;}
Use would be like:
<div id="box1" class="grid _2 alpha"></div>
<div id="box2" class="grid _3"></div>
<div id="box3" class="grid _3"></div>
<div id="box4" class="grid _4 omega"></div>
The only difference in the HTML would be a space between the 'grid' and '_8' instead of 'grid_8'.
Any thoughts?

Leonardo Hernandez
13,798 PointsI like the way that reads for its use!
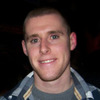
Michael Pare
6,358 PointsOut of curiousity, what is the point of separating the .grid class from the column width classes? Aren't you always going to couple them with grid? With that code, you'd also need to remove the "grid" class from either the first or last column or else you will end up with a width of 102%, unless you override the right hand margin with another class like .last {margin-right:0;}
If that works for you and your comfortable then go for it, haha, I've just never seen that type of naming convention before.
You may also want to make box1 and box2 classes as well, since having too many IDs can be a maintenance headache.
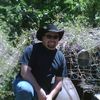
Mike Smith
11,863 PointsGood points Michael. What I was after was two-fold. One is to eliminate repetitive coding (DRY) and the second is to eliminate unnecessary bloat in the CSS file.
I edited the above code to reflect what you were describing. In the original grid.css, there were additional declarations ' .alpha{margin-left:0;}' and '.omega{margin-right:0;}' to address the extra margins. the first <div> would include 'alpha', last <div> would include 'omega'.
To better understand a new language (Sass to me right now) I try to refactor old working code and use it to teach myself the new tricks. Along the way, I find new shortcuts and improvements to speed up the loading and reduce coding errors.
That's why I love the discussion. It all helps me fine-tune the coding.
Thank-you!
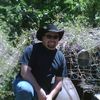
Mike Smith
11,863 PointsYES! That was the answer I was looking for. I was having trouble deciding where to put the extends. I was having a mental block.
Thank-you!
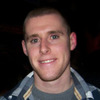
Michael Pare
6,358 PointsGlad I could help :)