Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial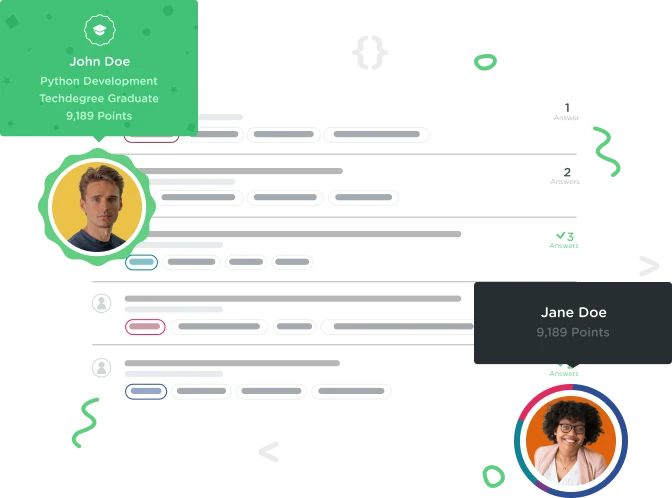

Jacob Corkran
2,774 PointsSave and load shopping list - found fix for \n on load
If you are having issues when loading your saved shopping list were it continually adds \n to each item creating spaces in your saved list, this will fix it. Just add [:-1] to the items you are appending back to your shopping list and it will strip out the \n. Here is an example:
with open(file_name, READ) as my_file:
for items in my_file:
shopping_list.append(items[:-1])
This was a major problem with the proper loading and saving of my shopping list, really had me stumped for awhile. Thought I would share because it was a really simple fix, only 5 characters.
Here is my full project:
import os
# make a list to hold onto items
shopping_list = []
# variables for open function
file_name = 'saved_list.txt'
WRITE = 'w'
READ = 'r'
def clear():
# clear the console
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def show_help() :
# print out instuctins on how to use the app
print("""
Enter 'DONE' when your are finished adding items.
Enter 'HELP' for the help message.
Enter 'SHOW' to see your current list of items.
""")
def show_list():
# print out the list
clear()
print("Here's your list:")
for item in shopping_list :
print(item)
def add_to_list(new_item) :
# add new items to out list
shopping_list.append(new_item)
print("added {}. List now has {} items. \n".format(new_item, len(shopping_list)))
def load_list():
# Check if file exist and load saved list
try:
with open(file_name, READ) as my_file:
for items in my_file:
shopping_list.append(items[:-1])
except:
# if file doesnt exist
return
def save_list():
# save current list for later
print('')
save_items = input("Would you like to save your shopping list? Y/n ").lower()
if save_items == 'n':
clear()
print("Thanks, your list will not be saved \n")
else:
with open(file_name, WRITE) as file:
for item in shopping_list:
file.write("{}\n".format(item))
clear()
print("Thanks, your list has been saved \n")
def main():
print("What should we pick up at the store?")
load_list()
show_help()
while True :
#ask for new item
new_item = input("Add Item > ")
if new_item == 'DONE' :
break
elif new_item =='HELP' :
show_help()
continue
elif new_item =='SHOW' :
show_list()
continue
add_to_list(new_item)
show_list()
save_list()
main()
1 Answer

Boban Talevski
24,793 PointsThat's a nifty trick :).
Before I checked this post, I discovered the handy string function rstrip which strips whitespace from the string (including the newline/return character) and solves the problem as well. Just adding this for anyone else facing the same issue.
def load_list():
with open('shopping_list.txt') as list_file:
for line in list_file:
shopping_list.append(line.rstrip())
Keith Ostertag
16,619 PointsKeith Ostertag
16,619 PointsThanks for sharing! I wouldn’t have thought of using a splice in quite that way...
BTW- you might look at using "wb" instead of 'w' to write to your file for compatibility with python2 (something I'm still investigating)