Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial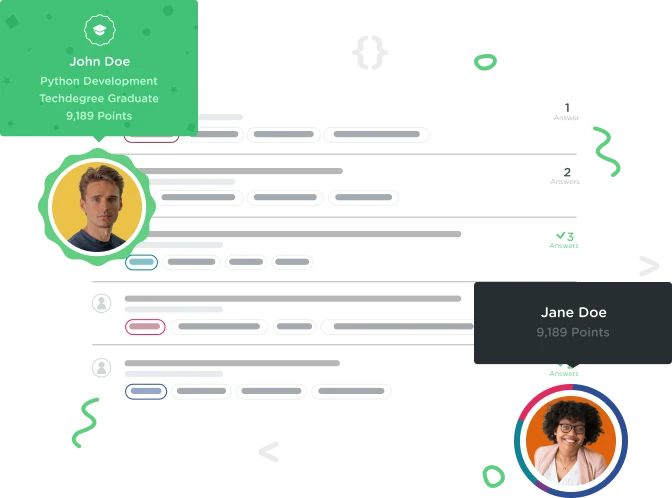

Michael Dawson
Courses Plus Student 2,719 PointsSaving Models and Redirecting code challenge
I'm well and truly stuck on the
Building Social Features in Ruby on Rails Building the Friendship UI Saving Models and Redirecting
code challenge! The requirement is to Set the flash success message to "Friendship created" if the @user_friendship is saved. But there seems to be very little context for this! What user friendship? We start with an empty 'new' method. Do we assume we need to copy the same code from our treebook application? Such as
def new
if params[:friend_id]
@friend = User.where(profile_name: params[:friend_id]).first
raise ActiveRecord::RecordNotFound if @friend.nil?
logger.info @friend
@user_friendship = current_user.user_friendships.new(friend_id: @friend)
else
flash[:error] = "Friend required"
end
rescue ActiveRecord::RecordNotFound
render file: 'public/404.html', status: :not_found
end
And then add something like @user_friendship.save if @user_friendship.save? flash[:info] = "Friendship created" end
?
As you can tell I'm fairly new to Ruby. But this challenge really confuses me, because unlike most other challenges, it's quite unclear what the requirements really are (to me). Any help appreciated!
27 Answers

Kjell Otto
1,614 PointsThe message here did the trick for me:
Jason Seifer 669 STAFF Posted 4 months ago
Hey Matthew! Try doing the following:
if @user_friendship.save
....
end
My complete block now looks like this and passes all tests:
class UserFriendshipsController < ApplicationController
def create
if params[:friend_id]
@friend = User.find(params[:friend_id])
@user_friendship = current_user.user_friendships.new(friend: @friend)
if @user_friendship.save
flash[:success] = "Friendship created."
redirect_to profile_path(@friend)
else
flash[:error] = "There was a problem."
redirect_to profile_path(@friend)
end
end
end
end
Hope this helps!
Greetings, Kjellski

jonathanrisinger
8,294 PointsHere is the solution:
def create @user_friendship = UserFriendship.new(params[:user_friendship]) if @user_friendship.save flash[:success] = "Friendship created." end end end
I think there should be a bit more specificity in instructions for the first line of code. The exact name of the :friend_id association for setting this instance variable is just left to guess work... ironically it was staring at me the whole time, but all the other examples we coded never implied that we should use this logic... and also we didn't know how the data base columns were named going in.
Maybe adding a new step or just giving it to us when we start would have saved some level of headache.
@user_friendship = UserFriendship.new(params[:user_friendship])
Hope this helps some people.

Andrew Merrick
20,151 PointsHere is the code that worked for me:
class UserFriendshipsController < ApplicationController
def create
# Write your code here
@user_friendship = UserFriendship.new
if @user_friendship.save
flash[:success] = "Friendship created."
else
flash[:error] = "There was a problem."
end
end
end
Part 2 states: If the @user_friendship variable cannot be saved, set the flash error message to "There was a problem."
So, all it's asking is to flash an error if the @user_friendship instance cannot be saved. It feels like a simple if and else statement.
if variable can be saved, do this. else, do that. I don't think it needs all of the extra params, etc - it just needs to flash a success or an error.
Does that make sense?
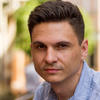
Nick Pettit
Treehouse TeacherHey Michael,
I don't know the answer to this, but I've notified Jason of your post so that he can respond.

Michael Dawson
Courses Plus Student 2,719 PointsThanks, I appreciate it.

Jason Seifer
Treehouse Guest TeacherHey Michael, is that what you put in the code challenge? You seem to be on the right track, just make sure to set the flash[:success] if the @user_friendship object is saved.

Michael Dawson
Courses Plus Student 2,719 PointsHi Jason - this is what I have in the code challenge:
class UserFriendshipsController < ApplicationController def new if params[:friend_id] @friend = User.where(profile_name: params[:friend_id]).first
raise ActiveRecord::RecordNotFound if @friend.nil?
@user_friendship = current_user.user_friendships.new(friend_id: @friend)
@user_friendship.save
if @user_friendship.save?
flash[:success]="Friendship created"
end
end
rescue ActiveRecord::RecordNotFound render file: 'public/404.html', status: :not_found end
end
But I'm getting an error: undefined method `create' for #<UserFriendshipsController:0x00000001749d88> (NoMethodError)
... which made me think that I needed to add a create method as well as the new method. I've tried this but haven't had any luck. Do you have any suggestions? Cheers, Mike :)

Jason Seifer
Treehouse Guest TeacherI fixed a small problem with the code challenge. Give it another try and let me know how it works out.

Michael Dawson
Courses Plus Student 2,719 PointsHi Jason. Thanks for the help, looks like you changed the method over to the create method? Unfortunately I'm still not having any luck. This is my code:
def create if params[:friend_id] @friend = User.find(profile_name: params[:friend_id])
@user_friendship = current_user.user_friendships.new(friend: @friend)
if @user_friendship.save
flash[:success] = "Friendship created"
redirect_to profile_path(@friend)
end
else
flash[:error] = "Friend required"
#redirect_to root_path
end
end
Thanks! In the meantime I'm just continuing on with other lessons. Thanks heaps for your work by the way, I've learnt a lot!

Njeri Chelimo
6,860 PointsHey Jason. I think there may still be a little problem with the challenge. I not sure I have fully understood the question. Am I meant to create the instance variable @user_friendship or does it exist? If I'm to create it, am I to do it the same way we did it in the project? Using the code in my user_friendships controller for direction, I have tried all solutions I can think of, but the challenge still does not pass.

Matthew Hartwig
3,941 PointsI'm also unable to pass the challenge...
def create
@user_friendship = current_user.user_friendships.new(friend: @friend)
@user_friendship.save
if @user_friendship.saved?
flash[:success] = "Friendship created"
end
end
Returns the following error:
Bummer! There was no flash success message set.

Jason Seifer
Treehouse Guest TeacherHey Matthew! Try doing the following:
if @user_friendship.save
....
end

Njeri Chelimo
6,860 PointsHey Jason! It still cannot pass for me.
class UserFriendshipsController < ApplicationController
def create
@user_friendship = current_user.user_friendships.new(friend: @friend)
if @user_friendship.save
flash[:success] = "Friendship created"
end
end
end

Jason Seifer
Treehouse Guest TeacherNjeri, do you have a @friend variable set up?

Njeri Chelimo
6,860 PointsThis is the code I am using. I have from the user_friendships_controller.rb file that I wrote while following the lesson: => It still doesn't pass.
class UserFriendshipsController < ApplicationController
def create
@friend = User.where(profile_name: params[:friend_id]).first
@user_friendship = current_user.user_friendships.new(friend: @friend)
if @user_friendship.save
flash[:success] = "Friendship created"
end
end
end

Michael Dawson
Courses Plus Student 2,719 PointsHi Jason. I just wanted to let you know that I'm still watching this thread for some clue as to what I'm doing wrong. Is it too much to ask for the correct code so we can all see what the problem is here? I've been keeping up with the code and have gone on ahead but haven't been able to make this challenge pass. Thanks, Michael

Jason Seifer
Treehouse Guest TeacherHi Michael,
There appears to be a small bug in the code challenge. Sorry about that! For now, try using the following method of creating a user friendship:
@friend = User.find(params[:friend_id]) @user_friendship = UserFriendship.new(user: current_user, friend: @friend)

Njeri Chelimo
6,860 PointsHi Jason. Thanks for your response. Unfortunately, this does not pass :| Getting, not flash message set, after setting it, @user_friendship is saved.

jonathanrisinger
8,294 PointsI've got the same problem.
Here is my ruby block:
def create @friend = User.find(params[:friend_id]) @user_friendship = UserFriendship.new(user: current_user, friend: @friend) if @user_friendship.save flash[:success] = "Friendship created" end end end
I believe that should work (I tried it in both Chrome and Firefox).... is there a problem on the server side?
If not could you please just post the correct answer for everyone.

jonathanrisinger
8,294 PointsI got to part three on this and got stuck again. I get the error:
No redirect occurred.
Which like the last time I believe I have the right syntax but redirect is not communicating with the server.
Here is one iteration of the code I used (which uses the code Jason provided above).
def create
@friend = User.find(params[:friend_id])
@user_friendship = UserFriendship.new(user: current_user, friend: @friend)
if @user_friendship.save
flash[:success] = "Friendship created."
redirect_to profile_path(@friend)
else
flash[:error] = "There was a problem."
end
end end
I've also tried many many different hash strings to try and connect to the friend column in the array. None have worked so far... I wish I new how the database columns were labeled... Please send us more info on this.
Thanks

jonathanrisinger
8,294 PointsThe instructions were again unclear.
The answer to my last post is this:
redirect_to profile_path(@friend)
Has to be in the "else" clause... clearly I was putting it in the "if" statement like we did in the "Creating the Friendship" video. In the code challenge there is no reason to even create a friend instance variable, even though the line implies one, just be sure on part three to throw the redirect command in the "else" clause.

Michael Dawson
Courses Plus Student 2,719 PointsHi Jonathan.
Thanks for the info on part one. I'd tend to agree, this question just seemed to be missing quite a bit of context - or at least to ruby learners. The other challenges build on each other in a pretty logical way but this one stumped me somewhat.
Perhaps that could be taken in as feedback Jason?
Cheers, Michael

Njeri Chelimo
6,860 PointsThanks Jonathan! :)

Shehryar Khan
Courses Plus Student 7,520 PointsNone of these suggestions seem to currently pass the tests as is.

Shehryar Khan
Courses Plus Student 7,520 PointsAll of this and the problem is not in the code. It's in the text validation for the flash message. The directions do not require a period, but the validation is looking for "Friendship created.", not "Friendship created". Adding the period makes the test pass with the properly scoped @user_friendship.
class UserFriendshipsController < ApplicationController def create @friend = User.find(params[:friend_id]) @user_friendship = current_user.user_friendships.new(friend: @friend) if @user_friendship.save flash[:success] = "Friendship created." end end end

Shehryar Khan
Courses Plus Student 7,520 PointsTo Jonathan's point about the redirect_to method: it doesn't need to be inside the if/else block at all. I put it outside the block and the test passed.
Though, in practice, this is probably not what we'd want.

Matt Witek
18,320 Pointsyeah, i dont think you want the redirect after the if else.. this should be updated.