Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial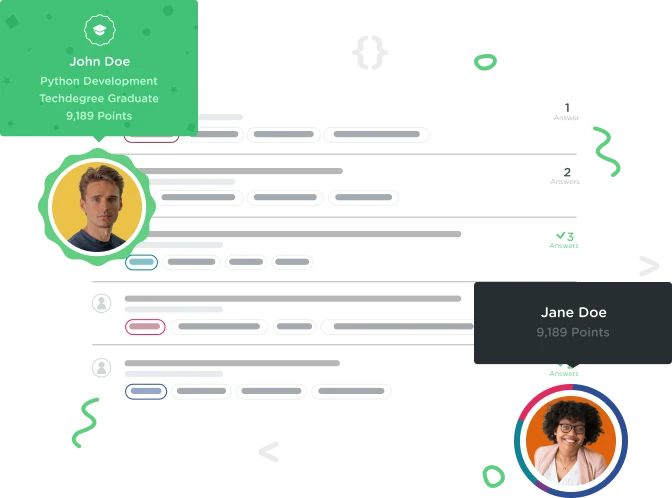

Thomas Katalenas
11,033 Pointssays int object is not iterable
def stats(our_dict):
list1 = []
for i in our_dict:
list1.append(i)
list1.append(len(our_dict[i]))
return list1
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(our_dict):
count = 0
max_count = 0
teacher = max(our_dict)
for i in our_dict:
count = len(our_dict[i])
if count > max_count:
max_count = count
if teacher == i:
return teacher
else:
return i
#def most_classes(our_dict):
# teacher = ' '
# count = 0
# max_count = 0
# for i in our_dict:
# count = len(our_dict[i])
# if count > max_count:
# max_count = count
# teacher = i
# return teacher
def num_teachers(our_dict):
return len(our_dict)
6 Answers

Thomas Katalenas
11,033 Pointstrying to create a list with the name and the number of classes it says int object not iterable. hmph i wonder why that error is there
anyway I found the solution
def stats(our_dict):
list1 = []
for i in our_dict:
j = []
j.append(i)
j.append(len(our_dict[i])) # create j a smaller list and then append that to list1
list1.append(j)
return list1

Thomas Katalenas
11,033 Pointsit works in my interpreter but treehouse error says: Looks like task 1 is no longer passing?
def courses(our_dict):
one_list = []
for i in our_dict:
one_list = one_list + our_dict[i]
return one_list
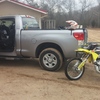
Ryan Ruscett
23,309 PointsHey,
Well, first thing is first. Only a sequence or otherwise known as a container is iterable. An iterable is defined as having a dunder method iter as one if it's built in methods.
For example
>>> a = 5
>>> type(a)
<class 'int'>
>>> dir(a)
['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
I create a variable called "a" and I assign it the integer 5. If I do type(a) it will tell me the type of object 'a' is. It returns integer. Ok so do a dir(a) which lists out the built in methods for that type of object. You can see there is not iter method in that list. Which means that an integer is not iterable. Now, let's make a list.
>>> a = [1,2,3,4,5]
>>> type(a)
<class 'list'>
>>> dir(a)
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
>>>
Now in the dir output, you can see iter. That means that a list is iterable. A list is a sequence and so is a string and dictionary.
Now I wont go into calling iter on an iterable to make an iterator and the use of next or next() depending on your python version. But the take home is... Integer is not a sequence but a single value. That means it's not iterable. To iterate over a single value offers no purpose.
Second part - Treehouse objectives test like this.
Test 1 - pass. Go to test two. Test 2 - tests for test 1 and 2 Test 3 - test for test 1, 2 and 3 test 4 - test for test 1, 2, 3 and 4
So if you are on say task 4. And it says task 1 is no longer passing. It's either because something you did messed up test 1 OR more likely, the code isn't compiling because there is an error and thus test one is failing.
I see in your last response it's courses - the method is not courses but "most_classes". That would fail step 1.
Hope this helps you out. You mentioned you found the answer so I wont go into that here.

Thomas Katalenas
11,033 Pointsno the i is the key in the dict and len is operates on the values to see how many their are.

Thomas Katalenas
11,033 Pointsthe answer is to create a seperate variable for classes = len(our_dict[i]) and then .append(classes)
perhaps it is saying that appending the len during an iteration is not alright
dir(len) does not show iterable
dir(append) doesn't even show up at all!?
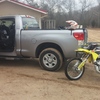
Ryan Ruscett
23,309 PointsYou need a variable that sits outside the loop that updates only when the length of the value is higher than the previous one. Each time that occurs, you need to update the teacher to reflect that.
dir(append) -- Doesn't work.
Append is an instance method of the class List. If you do dir(list) you will see append in the results. You can't dir(append). You can only dir(obj) and append is part of an object, but it's not an object.
Which is I will admit a bit confusing. Since dir(len) works because len() is actually a function which in turn is really an object and len() actually calls len. So if you dir(list) again you will also see len. Meaning that len(x) is really calling that objects built in len.
I think we are getting a bit more off track here. If you want to learn more about dir() you can google it.
If you want to get help on a method like append. try help(list.append).
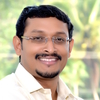
Aby Abraham
Courses Plus Student 12,531 Pointsdef most_classes(dict):
max_count = 0
str = ""
alist = dict.values()
for key, value in dict.items():
if len(value) > max_count:
max_count = len(value)
str = key
return str
def num_teachers(dict):
return len(dict.keys())