Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial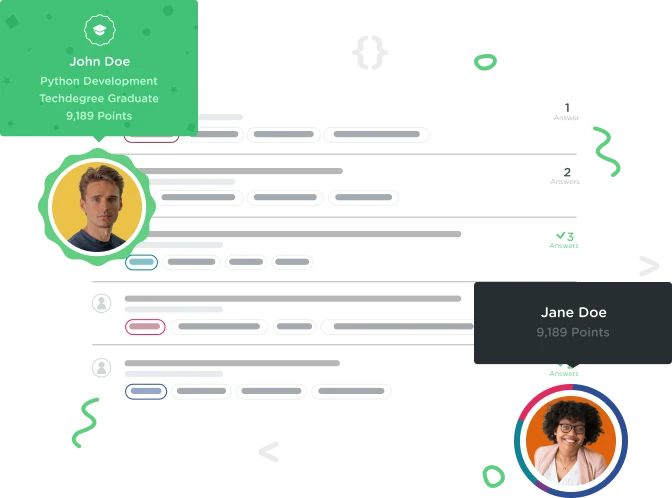

Trisha Escorpiso
551 PointsScope of a variable
I am new in python coding and I just don't understand why I did not received an error message when I use the variables "a" and "b" in the "else" block. What I expected is to received an error since the variables "a" and "b" is inside the "try" block, I am assumed that I can only use those variable inside the "try" block. Thank you in advance.
def add (num1, num2):
try:
a = float(num1)
b = float(num2)
except ValueError:
return None
else:
return a + b
def add (num1, num2):
try:
a = float(num1)
b = float(num2)
except ValueError:
return None
else:
return a + b
2 Answers
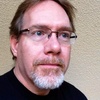
Chris Freeman
Treehouse Moderator 68,423 PointsThe built-in try
function does not create its own scope. Modules, classes, and functions create scope.
A complete description of Python scopes and namespaces in the docs.
Although scopes are determined statically, they are used dynamically. At any time during execution, there are at least three nested scopes whose namespaces are directly accessible:
• the innermost scope, which is searched first, contains the local names
(The variables a
and b
)
• the scopes of any enclosing functions, which are searched starting with the nearest enclosing scope, contains non-local, but also non-global names
(Arguments num1
and num2
. If assigned, then they become local)
• the next-to-last scope contains the current module’s global names
(The add
function)
• the outermost scope (searched last) is the namespace containing built-in names
(try
, except
, else
, float
, return
, None
, and ValueError
)
Post back if you need more help. Good luck!!!

Ben Reynolds
35,170 PointsIn most cases this would be true but try blocks are an exception to the rule in that they do not create their own scope. Instead, those two variables would end up in the scope of the add function.
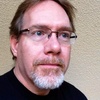
Chris Freeman
Treehouse Moderator 68,423 PointsIn which cases would it be true that a
and b
would be in a new scope?

Ben Reynolds
35,170 PointsBy that i mean the variables would normally be local to the block in which they're declared such as inside a function or class. To my knowledge a try block doesn't have that effect though and the variables declared within it are still available to other code in the same function.
In which cases would it be true that a and b would be in a new scope?
My first thought was a variable declared in a loop within a function, as opposed to a try block. Wouldn't the variable be local to that loop?
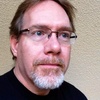
Chris Freeman
Treehouse Moderator 68,423 PointsThe loop variable used in a for
loop is usable outside the loop – after it ends. Thus it retains the last value used in looping even if the loop terminates early due to a break
.
Trying to use loop variable before the loop will raise an UnboundLocalError: local variable referenced before assignment