Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial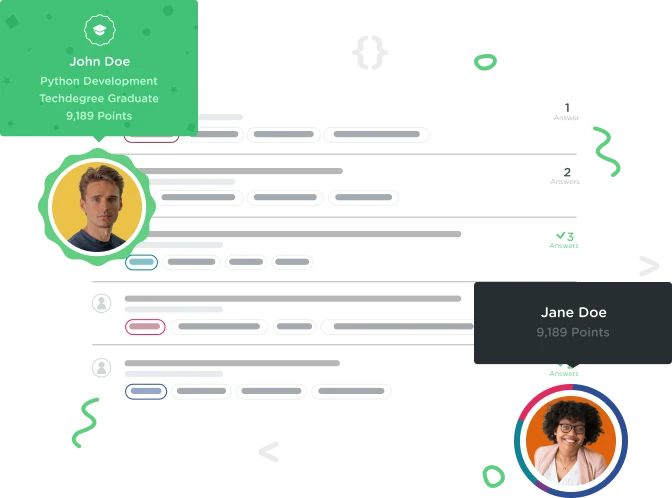
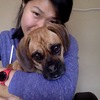
Seyoung Kwak
9,091 PointsScope of variation (Shadowing-Javascript)
How can I log out "Ryan"?
var person = "Sarah";
function whatever() {
person = "Ryan";
}
person = "Nick";
Answer with the explanation would be greatly appreciated!
3 Answers
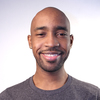
David Tonge
Courses Plus Student 45,640 Pointsvar person = "Sarah";
function whatever() {
var person = "Ryan";
return person; }
person = "Nick";
console.log(whatever());
console.log(person);
To get the function to return "Ryan" you must do two things: first set a local variable in the function and make sure your function returns the local variable using the "return" keyword. Here's where it gets a little tricky. Let's say you set the variable in the function without the "var" keyword as you did above and returned "person"...person would then be equaled to "Ryan" in the function then set to "Nick" outside the function since you assigned "Nick" to the variable person outside the function.
Short Answer: var "person" was a global variable, so unless it is set locally in the function then it will remain global and share the same place in memory changing every time you assign something to it.
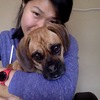
Seyoung Kwak
9,091 PointsHi David, I'm bit confused.. My initial question was variable("Ryan") without 'var'. As you said, there are two questions in the course. One with 'var' and one without. If you put 'var' in front of "Andrew", "Nick" is the answer. If you remove 'var', the answer becomes "Andrew". I just don't understand why the answer is "Andrew".
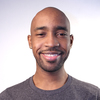
David Tonge
Courses Plus Student 45,640 PointsThere's two types of variables in Javascript.
Global and Local.
Without the "var" keyword the variable "person" remains Global.
The code cascades, so calling whoGotTheFunc() function after 'person = "Nick"' assigns "Andrew" to person.
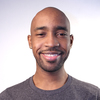
David Tonge
Courses Plus Student 45,640 Pointshere's an analogy I found online: http://www.quora.com/What-are-global-and-local-variables-in-JavaScript

Flora Karami
Front End Web Development Techdegree Student 14,947 PointsHi Seyoung, because when you don't use the 'var', you are shadowing the global variable with the local variable, meaning you are assigning the new value "Andrew" to the global "var person".
Seyoung Kwak
9,091 PointsSeyoung Kwak
9,091 PointsThis was actually from the quiz(Javascript Foundation). The answer was: whatever(name); console.log(name);
I wasn't trying to test anyone but I just don't understand why this print out "Ryan", not "Nick". Any explanation?
David Tonge
Courses Plus Student 45,640 PointsDavid Tonge
Courses Plus Student 45,640 PointsYou're mixing up the syntax from the quiz. There's two questions on that quiz. one says:
What will console log out in the following code?
The answer to this question is "Nick".
Here's my explanation from above and an added bit for clarity:
var "person" is a global variable, so unless it is set locally in the function then it will remain global and share the same place in memory changing every time you assign something to it. The variable inside of whosGotTheFunc() and the variable outside of the function are two different variables. In this case the person variable will inherent any value assigned to it outside the function and local variable, inside the function, stays the same.
This returns Andrew because without the "var" keyword the person variable is still going to be global. Then the whosGotTheFunc() function is called after "person = "Nick" so whosGotTheFunc() then changes person to "Andrew"