Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial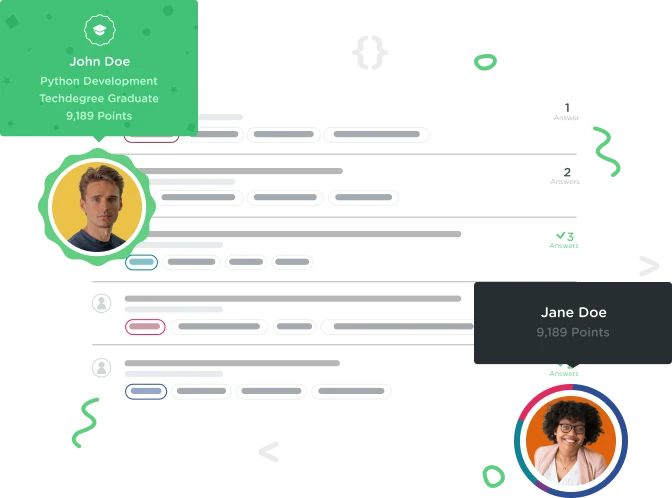

james white
78,399 PointsScoping error? ..and thinking about possible use of TryParse?
This code passes task 1 of 3:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
//try
//{
var numInput = int.Parse(Console.ReadLine());
//}
//catch(FormatException error)
//{
//Console.Write(“You must enter a whole number.”);
//}
string print = "";
for(int i = 0; i< numInput ; i++)
{
print = print + "Yay!";
}
Console.WriteLine(print);
}
}
}
However when I uncomment the try...catch lines (for task 2 of 3) suddenly I get an error:
Program.cs(19,31): error CS0103: The name `numInput' does not exist in the current context
Compilation failed: 1 error(s), 0 warnings
..where line 19 is the line that starts with 'for'
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
var numInput = int.Parse(Console.ReadLine());
}
catch(FormatException error)
{
//Console.Write(“You must enter a whole number.”);
}
string print = "";
for(int i = 0; i< numInput ; i++)
{
print = print + "Yay!";
}
Console.WriteLine(print);
}
}
}
So should that 'for' line be nested inside a finally somehow (maybe):
https://msdn.microsoft.com/library/k4hea629%28v=vs.100%29.aspx
The other confusing thing (and maybe I'm just over-thinking things...) is the Documentation video seemed to go out of it's way to show the use of TryParse when exploring that stackoverflow thread around time index 05:08.
.
Wouldn't using TryParse (instead of int.Parse) obviate the need to catch the FormatExecption?
https://teamtreehouse.com/library/c-basics/perfect/documentation
3 Answers
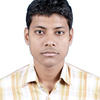
MD AFRID UDDIN MONDAL
2,877 PointsWhen you declaring "numInput" string variable in try block, it only exists in that block only. It does not mean anything outside of it. And that's why you got that scoping error. Just put your rest of the code inside your try block and everything will be fine. Just like that.........
Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
var numInput = int.Parse(Console.ReadLine());
string print = "";
for (int i = 0; i < numInput; i++)
{
print = print + "Yay!";
}
Console.WriteLine(print);
}
catch (FormatException error)
{
Console.Write("You must enter a whole number.");
}

james white
78,399 PointsDid a little more research in trying to do adjust catch for negative numbers (task 3 of 3)
and found this exception handling post which seems to recommend two catch statements.
http://www.dreamincode.net/forums/topic/200234-exception-handling/
So I'm wondering if this is the way to go....will have to play around with it when I have more time..

james white
78,399 PointsFor those who are looking for help with part 3 of 3 for the final course challenge this forum thread might be helpful:
james white
78,399 Pointsjames white
78,399 PointsThanks. That gets me past task 2 of 3, but then task 3 of 3 says:
Challenge Task 3 of 3
Add more input validation to your program by printing “You must enter a positive number.” if the user enters a negative number.
So I'm still wondering why TryParse (which seems to be faster and catches the exception) isn't the right way to go.
I found this article that says it has definite performance advantages:
http://blog.codinghorror.com/tryparse-and-the-exception-tax/