Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial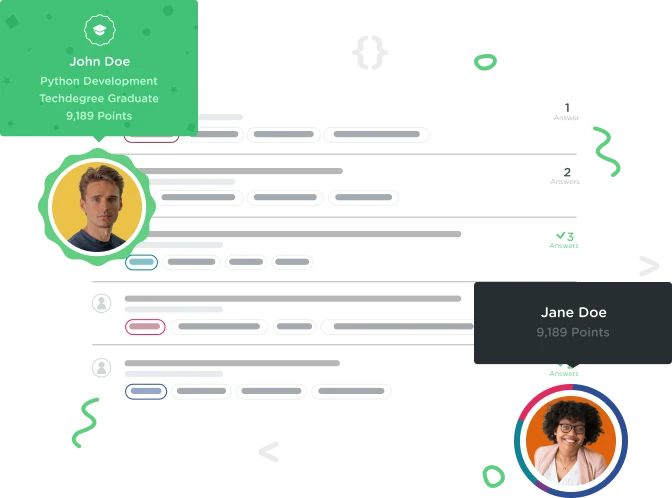
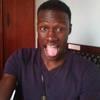
Paul Otieno
6,035 PointsScore
My code isn't working
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player=1):
self.player = self.current_score[1]
self.player += 1
1 Answer
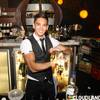
Micheal Allen
19,311 PointsHere's a break down of how to pass this challenge.
class Game:
def __init__(self):
self.current_score = [0, 0]
# self.current_score is a list of ints (integers)
# each int represents player_1 and player_2
# [0,0] = [player_1 , player_2]
# we'll need to use list indexing to target these players easily
# to target the first list item, we need to use 0, not 1
# to target player_1 we will use self.current_score[0]
# to target player_2 we will use self.current_score[1]
def score(self, player):
# score takes an argument called player.
if player == 1:
# if player_1 scores, increment self.current_score[0] by 1
self.current_score[0] += 1
# score is now self.current_score = [1, 0]
elif player == 2:
# if player_2 scores, increment self.current_score[1] by 1
self.current_score[1] += 1
# score is now self.current_score = [0, 1]
# if player_1 has scored 3 times and player_2 has scored 5 times
# the self.current_score would = [3, 5]
Be sure to pay close attention to how far your method, function or class blocks are indented. The code for your score method block was nested inside of the init method due to extra indentation.
Hope this helps!
Paul Otieno
6,035 PointsPaul Otieno
6,035 PointsThanks. Works like a charm