Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial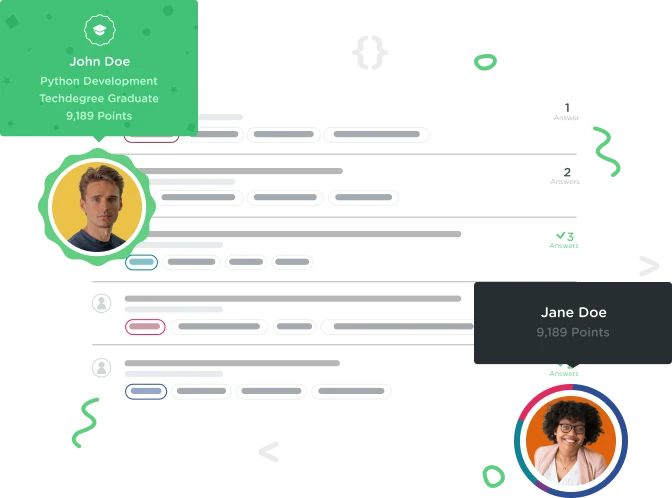

Edward Benzenberg
2,760 PointsScrabble exercise - iterate through char array and return array total. What is wrong with my code?
I don't have a compiler error but I get the message - Bummer, did you forget to create a method that excepts Chars.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(String getHand) {
int handCount = 0;
for (char pcs : getHand.toCharArray()) {
handCount ++;
}
return handCount;
}
}
2 Answers

Cindy Lea
Courses Plus Student 6,497 PointsYou have your string & int declarations different from mine in function decalration and your if different, this works for me:
public String getTileCount() {
int counter = 0;
for (char tile: mHand.toCharArray()) {
if(this.hasTile(tile)){
counter++;
}
}
return Integer.toString(counter);
}

Humayun Shimul
1,630 PointsYou need pass char as an argument instead of a String in getTileCount(char letter); Then use for each loop to compare each character from the mHand String to the char passed as an argument.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char letter) {
int handCount = 0;
char[] mHandArray=mHand.toCharArray();
for (char pcs : mHandArray) {
if(pcs==letter){
handCount ++;
}
}
return handCount;
}
}
```
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsCindy Lea - Again I have had to add Markdown to your reply!
While your participation in the Community is great, PLEASE refer to the "Markdown Cheatsheet" to see how to properly post code snippets in the forum so the students you are helping are able to read and understand the code you are providing. Even an experienced coder will have problems reading your code without markdown.
If you need further reference, here is a post by Dave McFarland (Treehouse Staff Instructor) on how to Properly post code in the Forum.
Thank-You
Jason
Treehouse Moderator
Cindy Lea
Courses Plus Student 6,497 PointsCindy Lea
Courses Plus Student 6,497 PointsOkay Json, but when I look at the text on my screen it likes fine. All nicely typed... Thank you for pointing me to the infor for adding code to forum. I think this just be noted at the top of the forum screen for other people to see at all times. Or is it already there?
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsThank-you Cindy Lea
When you type the answer, it will look okay in the box you are typing, but without the markdown, once you hit the Post button, all the code will condense down to one line with no line breaks. So, for example, the code here that I formatted, without it, it looked like this:
public String getTileCount() { int counter = 0; for (char tile: mHand.toCharArray()) { if(this.hasTile(tile)){ counter++; } } return Integer.toString(counter); }
The link to the Cheatsheet is actually right under the box where you type your answer.
Keep Coding!