Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial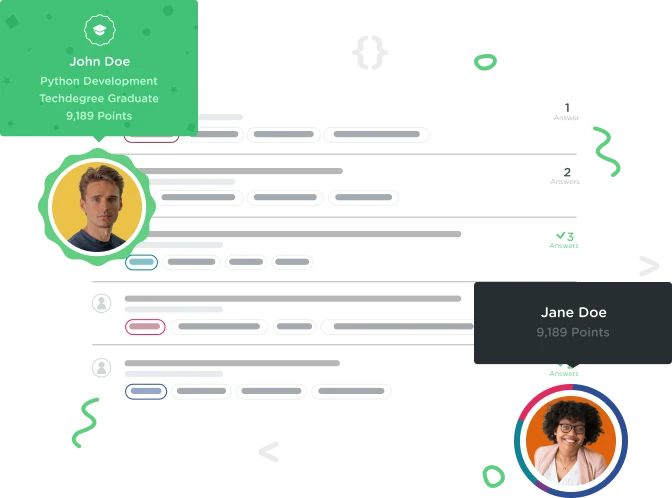

Kamran Ismayilov
5,753 PointsScrablePlayer
Please pay attention at my code. I actually finished this challenge. But I do not understand one thing. Is not "isHand" the same as "mHand.indexOf(tile) >= 0;
when I used firstone it did not work, but the secondone worked. Why?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
boolean isHand = mHand.indexOf(tile) >= 0;
mHand += tile;
}
public boolean hasTile(char tile) {
if(isHand) {
return true;
} else {
return false;
}
}
}
2 Answers

rtholen
Courses Plus Student 11,859 Points- This should not compile as written. You have a variable "isHand" being used in the member function hasTile(char tile) but it is not defined as either a local or member variable. Java won't let you use variables without first declaring their type. You did declare "isHand" as a local variable in the addTile(char tile) member function, but that declaration is not "usable" in the "hasTile(char tile)" function. Local variables are only "usable" inside the braces they are declared.
- You are correct that "isHand" is the same as "mHand.indexOf(tile) >= 0", because you set them equal. :)
- You might also note that "mHand.indexOf(tile) >= 0" implements in code what is being asked by "hasTile(char tile)" in English. In other words, "hasTile(char tile)" should be computing the result of "mHand.indexOf(tile) >= 0", not "addTile(char tile)".
Christopher Augg
21,223 PointsKamran,
In the code you have provided, you are declaring isHand within the addTile method making it local to that method only. Therefore, when you attempt to use isHand in the hasTile method, you are attempting to use a variable that has not been declared.
As a side note, the addTile method should only be responsible for adding the tile being passed into it.
public void addTile(char tile) {
mHand += tile;
}
I would also like to mention that an if statement is not required in the hasTile method since you are already getting a boolean from mHand.indexOf(tile) >= 0; You can simply return that statement.
public boolean hasTile(char tile) {
return mHand.indexOf(tile) >= 0;
}
Hope that helps.
Regards,
Chris