Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial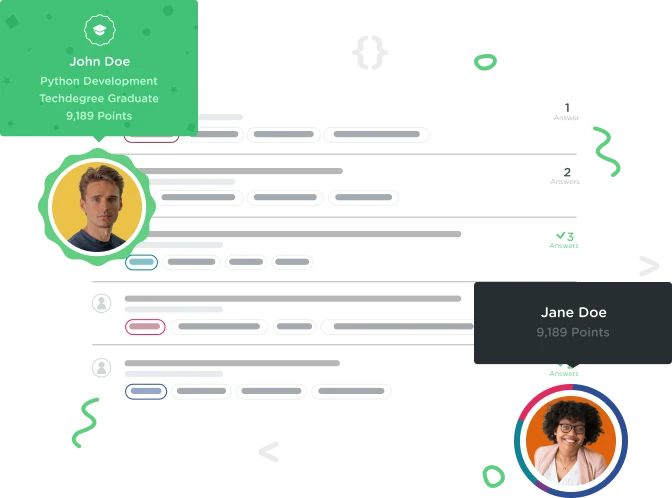

davidreyes2
3,422 PointsSearch Javascript Code
When I preview this code, the console is giving me an error of
SyntaxError: missing } in compound statement search.js:23:2
I've looked over the code over and over and looked through the braces and they seem to be correct. Can you please help me!? :(
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while (true) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit.");
search = search.toLowerCase();
if ( search === 'quit') {
break;
} else if ( search === 'list') {
print(inStock.join(', '));
break;
} else {
if (inStock.indexOf(search) > -1) {
print('Yes, we have ' + search + ' in the store. ');
}
else {
print(search + ' is not in stock.' );
}
}
And when I add where I think the brace go, I get this error SyntaxError: expected expression, got keyword 'else' search.js:20:4
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while (true) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit.");
search = search.toLowerCase();
if ( search === 'quit') {
break;
} else if ( search === 'list') {
print(inStock.join(', '));
break;
} else {
if (inStock.indexOf(search) > -1) {
print('Yes, we have ' + search + ' in the store. ');
}
} else {
print(search + ' is not in stock.' );
}
}
2 Answers
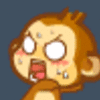
shadryck
12,151 PointsYour code is messed up a little bit at the second Else statement.
} else {
if
You should write it like Else if statement. As example;
var stock = ['apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread'];
var exitLoop = false;
function isInStock(check){
var checked = stock.indexOf(check);
while (exitLoop === false) {
if (checked >= 0) {
alert(check + " is in stock.");
exitLoop = true;
} else if (check.toLowerCase(", ") === 'list') {
alert(stock.join(', '));
exitLoop = true;
}
else if (!check || check === "quit") {
alert('Exiting program');
exitLoop = true;
} else {
alert("Something went wrong.");
break;
}
}
}
isInStock(prompt("Search for a product in our store. Type \'list\' to show all of the produce and \'quit\' to exit."));
//EDIT: sorry I had to edit this post a couple times, I'm not the best writer in the night :) //EDIT: if a mod happends to pass by, any idea why the code block wont be recognized as javascript? Its so grey.

Iain Simmons
Treehouse Moderator 32,305 PointsIt's actually where you have the last else
, it's proceeded by two closing/right curly braces. Move one of those to the end and it should work. You'll probably need to fix up your indenting for things to look clear also.
Kevin Korte
28,149 PointsKevin Korte
28,149 Pointsshadryck I got the code syntax highlighting to work. The parser doesn't always figure it out, but you can manually force it to highlight correctly by adding the language after the 3 ticks ( ``` ). You can do stuff like css, html, php, js, and so on.
Hope that helps and thanks for writing an answer.
shadryck
12,151 Pointsshadryck
12,151 Points@Kevin Korte Sweet, it's a lot easier than adding spaces like in stack overflow. Thanks for the tip! Also is there a way to force linebreaks? br> and /n dont seem to do the trick. The markdown cheatsheet didn't mention it either.
Kevin Korte
28,149 PointsKevin Korte
28,149 PointsNot that I'm aware of yet unless you hit enter twice to drop down 2 lines, but that gets ugly and long. I'm not super great with Markdown though so maybe there is a better solution.