Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial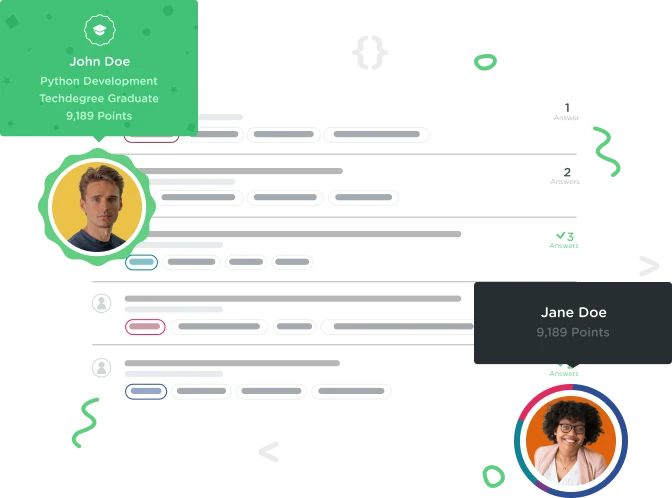
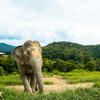
Noelle Szombathy
10,043 PointsSearching for multiple students in the database - shows info for student but also says no student found.
I am trying to take the Student Record Search a step further by allowing the user to type in multiple names (one at a time) and get results for all the students typed in, whether they were found in the database or not. I have the program working almost perfectly, but when I search for a student who exists, but is not the first student in the database (i.e. it doesn't work correctly for "Susan" or "Tony" but it does for "Dave") it prints out their information AND says that the student was not found in the database.
The code that I have so far is:
var search;
//Creates array to store records of multiple students in database
var searches = [];
var validStudents = [];
var message = "";
var student;
var studentName;
var noStudents = [];
var recordArray = [];
//Formatted message printed to the document in the "right place"
function print(message) {
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
//Pulls and returns information on a single student at a time
function getStudentReport(student){
var report = "<h2>Student: " + student.name + "</h2>";
report += "<p>Track: " + student.track + "</p>";
report += "<p>Achievements: " + student.achievements + "</p>";
report += "<p>Points: " + student.points + "</p>";
return report;
}
while (true){
//Asks user for input
search = prompt("Please enter a student's name or type 'quit' to end the program");
//Allows user to quit program
if (search.toLowerCase() === 'quit' || search === null){
break;
}
//Cycles through students database
for (var i = 0; i < students.length; i += 1) {
student = students[i];
//If search matches student's name in database then get that student's info and store it into an array
if (search === student.name) {
recordArray.push(getStudentReport(student));
validStudents.push(search);
print(recordArray);
} else {
//checks if name is stored in "noStudents" or "validStudents" lists
if (validStudents.indexOf(search) !== -1){
console.log("validStudents = " + validStudents);
} else if (noStudents.indexOf(search) !== -1) {
console.log("Already in the array");
console.log("noStudents = " + noStudents);
} else {
noStudents.push(search);
message = "No info found for: " + noStudents.join(", ");
}
}
}
//If search does not match student's name, store that name in an array to print out later
message += recordArray;
print(message);
}
2 Answers

KRIS NIKOLAISEN
54,967 PointsI think this is closer to what you are looking for
var search;
//Creates array to store records of multiple students in database
var searches = [];
var validStudents = [];
var message = "";
var student;
var studentName;
var noStudents = [];
var recordArray = [];
//Formatted message printed to the document in the "right place"
function print(message) {
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
//Pulls and returns information on a single student at a time
function getStudentReport(student){
console.log(student.name)
var report = "<h2>Student: " + student.name + "</h2>";
report += "<p>Track: " + student.track + "</p>";
report += "<p>Achievements: " + student.achievements + "</p>";
report += "<p>Points: " + student.points + "</p>";
return report;
}
while (true){
//Asks user for input
search = prompt("Please enter a student's name or type 'quit' to end the program");
//Allows user to quit program
if (search === null || search.toLowerCase() === 'quit'){
break;
}
//Cycles through students database
for (var i = 0; i < students.length; i += 1) {
student = students[i];
//If search matches student's name in database then get that student's info and store it into an array
if (search === student.name) {
recordArray.push(getStudentReport(student));
validStudents.push(search);
// print(recordArray);
}
}
if (validStudents.indexOf(search) !== -1){
console.log("validStudents = " + validStudents);
} else if (noStudents.indexOf(search) !== -1) {
console.log("Already in the array");
console.log("noStudents = " + noStudents);
} else {
noStudents.push(search);
message = "No info found for: " + noStudents.join(", ");
}
}
message += recordArray;
print(message);
For the most part I made two changes
(1) Move checking the validStudents and noStudents arrays for search
to after the for loop. This is so searching the entire students array is complete before doing so.
(2) Move printing the message to outside the while loop. This is so all searches are finished before concatenating the recordArray.
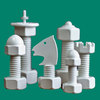
Steven Parker
229,744 PointsCurrently, the code that makes the "not found" determination and prints the message is inside the search loop. But a correct determination can only be made after the loop has checked all the entries.
A bit of re-organizing of the logic will be needed.
Noelle Szombathy
10,043 PointsNoelle Szombathy
10,043 PointsYes, that solved the problem! Thank you so much!