Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial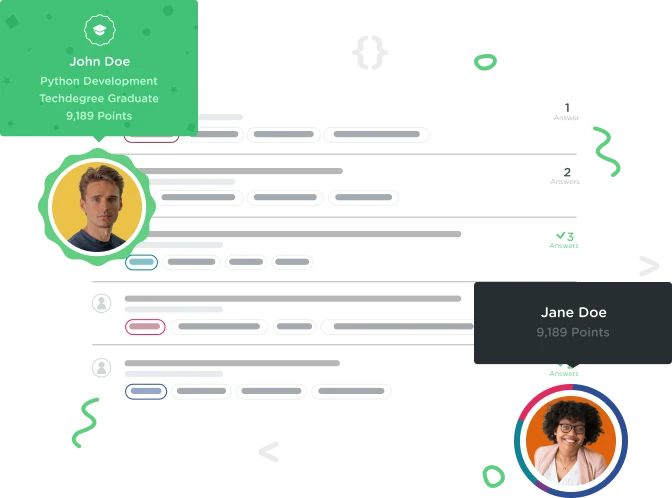

Joseph LoForti
9,942 PointsSearching for News Headlines - I cannot follow this video as the microsoft website has totally changed since this video
How do i find the code she copies and pastes in this video. The microsoft web page she links to has changed since this video was uploaded.
1 Answer

Joe Whitehead
2,179 PointsI had the same issue, thankfully after a bit of searching and re-watching the videos I've a solution that works. Helpful resources for me were:
From the API I was able to see what the json response would have in it so I could pick what I wanted to deserialize and it simply looks like this:
public class NewsSearch
{
[JsonProperty(PropertyName = "value")]
public List<NewsResult> NewsResults { get; set; }
}
public class NewsResult
{
[JsonProperty(PropertyName = "name")]
public string Headline { get; set; }
[JsonProperty(PropertyName = "description")]
public string Summary { get; set; }
[JsonProperty(PropertyName = "category")]
public string Category { get; set; }
[JsonProperty(PropertyName = "datePublished")]
public DateTime DatePublished { get; set; }
}
You can add more properties but I only wanted these ones.
Then I used the SDK example to help with getting the web result, feeding it into the StreamReader and JsonTextReader to deserialize it into the NewsResult list.
public static List<NewsResult> GetNewsForPlayer(string playerName)
{
string subscriptionKey = "YOUR_API_KEY";
string endpoint = "https://api.bing.microsoft.com/v7.0/news/search";
var results = new List<NewsResult>();
Console.WriteLine("Searching the Web for: " + playerName);
// Construct the URI of the search request
var uriQuery = endpoint + "?q=" + Uri.EscapeDataString(playerName);
// Perform the Web request and get the response
WebRequest request = HttpWebRequest.Create(uriQuery);
request.Headers["Ocp-Apim-Subscription-Key"] = subscriptionKey;
HttpWebResponse response = (HttpWebResponse)request.GetResponseAsync().Result;
var serializer = new JsonSerializer();
using (var reader = new StreamReader(response.GetResponseStream()))
using (var jsonReader = new JsonTextReader(reader))
{
results = serializer.Deserialize<NewsSearch>(jsonReader).NewsResults;
}
return results;
}
The rest of it can then follow on with the tutorial to loop through your players and output the results
var topTenPLayers = GetTopTenPlayers(players);
foreach (var player in topTenPLayers)
{
List<NewsResult> newsResults = GetNewsForPlayer(string.Format("{0} {1}", player.FirstName, player.SecondName));
foreach(var result in newsResults)
{
Console.WriteLine(string.Format("Date: {0:f}\r\nHeadline: {1}\r\nSummary: {2}\r\n", result.DatePublished, result.Headline, result.Summary));
Console.ReadKey();
}
}
Hope this helps, as a fellow student I would also appreciate if someone notices I have done anything which is bad practice or any improvements that can be made to the code.
Christian Loveridge
3,063 PointsChristian Loveridge
3,063 PointsUnfortunately this is somewhat of a trial-by-fire until they remake this course. Ideally they'd just make their own API for you to hit, you'd think.
The video does give you an idea of what to look for, but you'll have to just focus on the concepts rather than step-by-step instructions. In short, they've already given you all the tools necessary to fly the nest up to this point: programs, classes, web requests, reading documentation, getting API keys, and handling the output...and now it's time to put it all together as if you were hired to do this for a job.
To get the API key, I just googled "Bing news API" and got strated for free. Create/log into an Azure account, and create a new Bing Resource (Free). The API keys are given to you inside Azure in that resource.
WebClient() seems to be deprecated, so use HttpClient() instead -- Microsoft has a tutorial of how to use it to make a news request, along with the headers if needed. Look up additional tutorials if something isn't clicking, and ChatGPT is an amazing resource for breaking coding issues down further if you can't quite get it.
I can't find a good example of the JSON output on Microsoft's site, but you can store the JSON response in a string and then copy the string into a new class and proceed from there.
To anyone reading this far, good luck! You got this!