Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial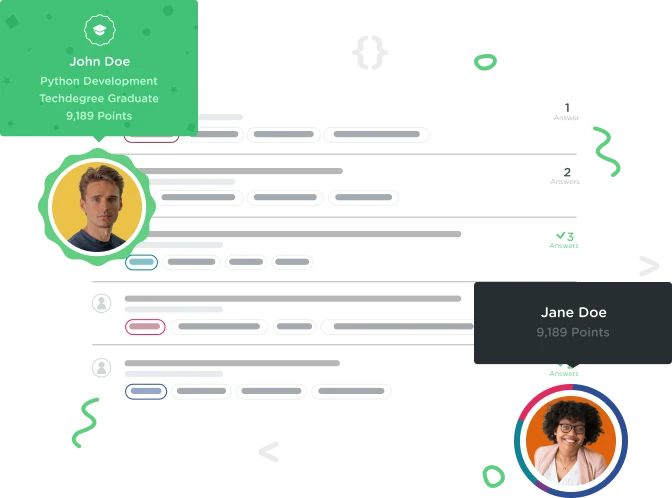
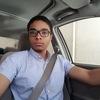
Luqman Shah
3,016 PointsSecond Attempt - Please Review My Code
var questions = 5;
var correct = 0
var one = prompt( "Which popular snack has the mixture of chocolate and peanut butter?" );
if ( one.toLowerCase() === "reese's" || one.toLowerCase() === "reeses" ) {
alert("Correct!");
correct +=1
} else {
alert("Wrong!");
correct -=1
}
var two = prompt( "What's 2 x 2?" );
if ( parseInt(two) === 4 || two.toLowerCase === 'four' ) {
alert("Correct!");
correct +=1
} else {
alert("Wrong!");
correct -=1
}
var three = prompt("Fill in the blank: Jackie ____ played a cop in A Police Story");
if ( three.toLowerCase() === "chan" ) {
alert("Correct!");
correct +=1
} else {
alert("Wrong!");
correct -=1
}
var four = prompt("Who is our current president?");
if ( four.toLowerCase() === "donald trump" || four.toLowerCase() === "donald j trump" || four.toLowerCase() === "trump" ) {
alert("Correct!");
correct +=1
} else {
alert("Wrong!");
correct -=1
}
var five = prompt("html is a programming language, true or false?");
if ( five.toLowerCase() === "false" ) {
alert("Correct!");
correct +=1
} else {
alert("Wrong!");
correct -=1
}
if ( correct === 5 ) {
alert("Nice! You've earned a gold crown!");
} else if ( correct <= 4 ) {
alert("Good! You've earned a silver crown");
} else if ( correct <= 3 ) {
alert("Good! You've earned a silver crown");
} else if ( correct <= 2 ) {
alert("Ok! You've earned a bronze crown");
} else if ( correct === 0 ) {
alert("Sorry, you lose!");
}
2 Answers
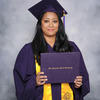
Manjila Nakarmi
7,449 Pointsall of your correct +=1 or correct-=1 dont end with semi colon.May be that is the issue? even when you declare correct variable the semicolon is missing

Seth Kroger
56,415 Pointsif ( correct === 5 ) {
alert("Nice! You've earned a gold crown!");
} else if ( correct <= 4 ) {
alert("Good! You've earned a silver crown");
} else if ( correct <= 3 ) {
alert("Good! You've earned a silver crown");
} else if ( correct <= 2 ) {
alert("Ok! You've earned a bronze crown");
} else if ( correct === 0 ) {
alert("Sorry, you lose!");
}
You have correct less than or equal to n in your if statements, which means you'll only get as far as the second, because any score that isn't 5 will be 4 or less. (semi-colons at the end of statements are optional in JavaScript so it's not an error, but it is inconsistent to use them sometimes and omit them in others)
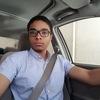
Luqman Shah
3,016 PointsSo that's a good thing? I see, but it's good practice generally speaking, right?
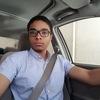
Luqman Shah
3,016 PointsOk so I watched the solution video. here's how he wrote it:
if (correct === 5) {
document.write('gold crown');
} else if (correct >= 3) {
document.write('silver crown');
} else if (correct >= 1) {
document.write('bronze crown');
} else {
document.write('no crown');
}
So essentially, if you get 5, the first conditional only runs and everything else is skipped. And if the amount you get correct is greater than or equal to 3, that condition only runs. So >= 3
= 4 or 3, and 5, but because there is already a conditional for 5, the second conditional won't run, only the first one will? If you were to get 5, even though it specifically states anything equal to or greater than 3? Etc..
Luqman Shah
3,016 PointsLuqman Shah
3,016 PointsAhh thank you for pointing that out, rookie mistake xD