Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial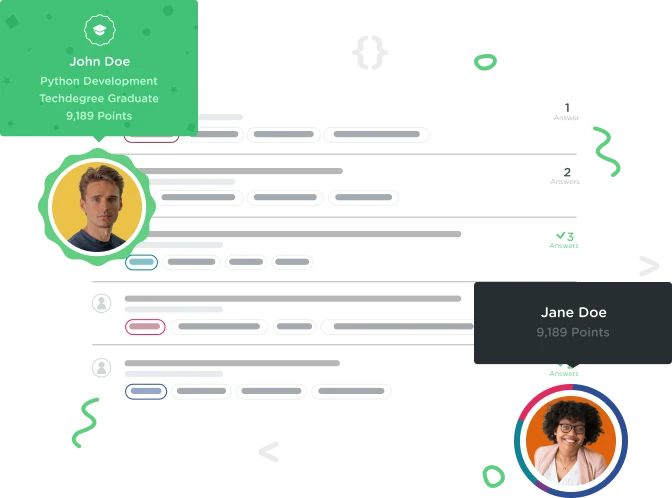

Mohamed THIAM
1,091 PointsSecond Shopping list App
Hello everyone,
So I did the Second Shopping list App challenge and got it to work without using functions, ELIFs or ELSEs. FOR some reason, the moment I do things stop working.
Can someone please walk me through optimizing my code?
Thanks!
shopping_list = []
print('What would you like to add to your list?')
print('Enter DONE when you are finished adding items.')
print('Enter HELP for assistance.')
print('Enter SHOW to see all your current items.')
while True:
new_item = input('> ')
if new_item == 'DONE':
break
shopping_list.append(new_item)
if new_item == 'HELP':
shopping_list.remove('HELP')
print('Add an item to your list and enter DONE when you are finished.')
if new_item == 'SHOW':
print('Here are your current items: ')
shopping_list.remove('SHOW')
for item in shopping_list:
print(item)
print('Here is your list of items:')
for item in shopping_list:
print(item)
Here is a second attempt:
shopping_list = []
print('What would you like to add to your list?')
def show_help():
print('''Enter DONE when you are finished adding items.
Enter HELP for assistance.
Enter SHOW to see all your current items.''')
def show_list():
print('Here are your current items: ')
for item in shopping_list:
print(item)
def main():
while True:
new_item = input('> ')
if new_item == 'DONE':
break
shopping_list.append(new_item)
if new_item == 'HELP':
shopping_list.remove('HELP')
show_help()
if new_item == 'SHOW':
shopping_list.remove('SHOW')
show_list()
print('Here is your list of items:')
for item in shopping_list:
print(item)
show_help()
main()
[MOD: added python to the markdown formatting -cf]
2 Answers

Mohamed THIAM
1,091 PointsThis is awesome Andreas. I'm still figuring out my code hierarchy. Sometimes, it's the reason my script doesn't run. You made it very clear. Thanks for taking the time.
Mo.
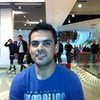
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Mohamed
Elif works for me, also if you append the new item's to the list at the end after all the conditions are accounted for you wont need to remove help and show from the list. Also you dont need the while loop inside a function called main.
shopping_list = []
print('What would you like to add to your list?')
def show_help():
print('''
Enter DONE when you are finished adding items.
Enter HELP for assistance.
Enter SHOW to see all your current items.''')
def show_list():
print('Here are your current items: ')
for item in shopping_list:
print(item)
while True:
new_item = input('> ')
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
shopping_list.append(new_item)
show_list()