Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial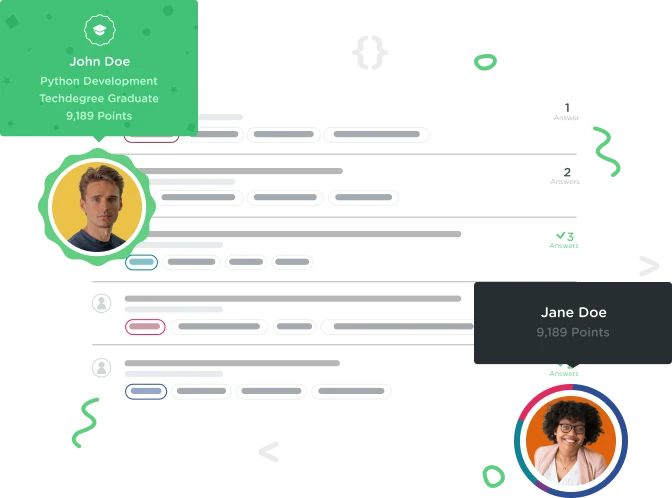

natem2
74 PointsSee the following error on 'Post Form and View' code: __init__() missing 1 required positional argument: 'model'
Any idea how I can fix this error?
Traceback (most recent call last): File "app.py", line 7, in <module> import forms
File "/flask-social/forms.py", line 6, in <module> from models import User
File "/flask-social/models.py", line 62, in <module> class Post(Model):
File "/flask-social/models.py", line 66, in Post related_name='posts'
TypeError: init() missing 1 required positional argument: 'model'
6 Answers

Wes R
4,527 PointsPeewee 3.x no longer supports rel_model
or related_name
- instead, use model
and backref
. See their change log here.
Try using the following:
class Post(Model):
timestamp = DateTimeField(default=datetime.datetime.now)
user = ForeignKeyField(
model=User,
backref='posts')
content = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)

Wes R
4,527 PointsHmm, you do indeed need to fix the tuple but it sounds like you're missing your shebang line from your app.py. While your virtual environment is active, try which python3
. Most likely, the path you see should be for the python you have in your environment. For example, mine is something like:
/Users/myname/my/project/path/.../social-app/env/bin/python3
Copy all of it and add it to the top of your app.py like this, replacing it with your path:
#!/Users/myname/my/project/path/.../social-app/env/bin/python3

natem2
74 PointsThat worked, thank you Wes.
The code is now throwing another set of errors, seems related to Peewee update again.
Can I assume the crux of the issue is the need to add join_date? Because it can't truly be that the user already exists since the info I am using is all different. Not sure how to deal with this one.
Traceback (most recent call last):
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2875, in execute_sql
cursor.execute(sql, params or ())
sqlite3.IntegrityError: NOT NULL constraint failed: user.join_date
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/flask-social/models.py", line 57, in create_user
is_admin=admin)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 5816, in create
inst.save(force_insert=True)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 5967, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 1737, in inner
return method(self, database, *args, **kwargs)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 1808, in execute
return self._execute(database)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2512, in _execute
return super(Insert, self)._execute(database)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2275, in _execute
cursor = database.execute(self)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2888, in execute
return self.execute_sql(sql, params, commit=commit)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2882, in execute_sql
self.commit()
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2666, in __exit__
reraise(new_type, new_type(*exc_args), traceback)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 179, in reraise
raise value.with_traceback(tb)
File "/flask-social/myenv/lib/python3.7/site-packages/peewee.py", line 2875, in execute_sql
cursor.execute(sql, params or ())
peewee.IntegrityError: NOT NULL constraint failed: user.join_date
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 2309, in __call__
return self.wsgi_app(environ, start_response)
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 2295, in wsgi_app
response = self.handle_exception(e)
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 1741, in handle_exception
reraise(exc_type, exc_value, tb)
File "/flask-social/myenv/lib/python3.7/site-packages/flask/_compat.py", line 35, in reraise
raise value
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 2292, in wsgi_app
response = self.full_dispatch_request()
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 1815, in full_dispatch_request
rv = self.handle_user_exception(e)
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 1718, in handle_user_exception
reraise(exc_type, exc_value, tb)
File "/flask-social/myenv/lib/python3.7/site-packages/flask/_compat.py", line 35, in reraise
raise value
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 1813, in full_dispatch_request
rv = self.dispatch_request()
File "/flask-social/myenv/lib/python3.7/site-packages/flask/app.py", line 1799, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "/flask-social/app.py", line 55, in register
password=form.password.data
File "/flask-social/models.py", line 59, in create_user
raise ValueError("User already exists")
ValueError: User already exists

Ben Jakuben
Treehouse TeacherHowdy all, just wanted to give a no-update update and let you know that Ashley Boucher and I are digging into this. This course is on our roadmap for a full refresh, but we'll see if we can fix this specific issue in the mean time.

saeed shaban
133 Pointsi have this problem now what is solution please ?
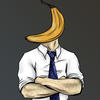
Lee B
1,141 PointsCould you post your code where that class is? Hard to say what it is when we can't look at the code.
From my understanding, these types of errors happen when a variable in the class calls on an argument that doesn't actually exist. In your case, 'model' may not be defined.
Make sure you 'model' is being called in the arguments of the __init__
class NateMan:
def __init__(self, model):
self.model = model
def showModel(self):
print(self.model)

natem2
74 PointsHi Pierson, yes, I actually compared and ran into this error with the supplied class files.
So it seems to be an issue not just in my code but also in the provided files. So I don't think its just what I coded.
Showing code snippets below for
1) the lines inclusive of 62 and 66 the traceback error references from models.py
2) the main initialization lines from app.py (though traceback does not show an error explicitly here)
3) then the full models.py code just as reference
Thank you for responding and looking at this issue!
class Post(Model):
timestamp = DateTimeField(default=datetime.datetime.now)
user = ForeignKeyField(
rel_model=User,
related_name='posts'
)
content = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)
if __name__ == '__main__':
models.initialize()
try:
models.User.create_user(
username='kennethlove',
email='kenneth@teamtreehouse.com',
password='password',
admin=True
)
except ValueError:
pass
app.run(debug=DEBUG, host=HOST, port=PORT)
import datetime
from flask_bcrypt import generate_password_hash
from flask_login import UserMixin
from peewee import *
DATABASE = SqliteDatabase('social.db')
class User(UserMixin, Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField(max_length=100)
joined_at = DateTimeField(default=datetime.datetime.now)
is_admin = BooleanField(default=False)
class Meta:
database = DATABASE
order_by = ('-joined_at',)
def get_posts(self):
return Post.select().where(Post.user == self)
def get_stream(self):
return Post.select().where(
(Post.user << self.following()) |
(Post.user == self)
)
def following(self):
"""The users that we are following."""
return (
User.select().join(
Relationship, on=Relationship.to_user
).where(
Relationship.from_user == self
)
)
def followers(self):
"""Get users following the current user"""
return (
User.select().join(
Relationship, on=Relationship.from_user
).where(
Relationship.to_user == self
)
)
@classmethod
def create_user(cls, username, email, password, admin=False):
try:
with DATABASE.transaction():
cls.create(
username=username,
email=email,
password=generate_password_hash(password),
is_admin=admin)
except IntegrityError:
raise ValueError("User already exists")
class Post(Model):
timestamp = DateTimeField(default=datetime.datetime.now)
user = ForeignKeyField(
rel_model=User,
related_name='posts'
)
content = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
to_user = ForeignKeyField(User, related_name='related_to')
class Meta:
database = DATABASE
indexes = (
(('from_user', 'to_user'), True)
)
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Post, Relationship], safe=True)
DATABASE.close()

natem2
74 PointsHi Ben, thanks for posting and letting me know. As it’s been 1 month, is there any further update on a possible specific fix for this issue and timing on course refresh? I care specifically about this class for being able to apply it in my own projects. It is a high priority for me, so hoping for a solution. Thank you!

Ben Jakuben
Treehouse TeacherI'm sorry about the delayed response here. We're revamping Python plans for the rest of the year, and it looks like we're going to focus on Django refreshes over Flask because of its higher demand in the job market. We are also confident that students who learn Django with us will be able to pick up Flask pretty easily after that. This is a little bit of a moving target, but it means we'll retire Flask content as issues like this pop up, and with updated Django content, we'll be able to offer a better learning path in the Techdegree and Django track.
natem2
74 Pointsnatem2
74 PointsThanks Wes, I updated and then it threw some other peewee errors.
Which I saw a fix for stating it was due to tuples in peewee, and would fix key error 'f;
https://stackoverflow.com/questions/49115997/peewee-throws-keyerror-f
I then applied the above suggested additional fix
indexes = ((('from_user', 'to_user'), True),)
But then it throws this error below. So not sure if the above tuple fix was best fix, but if yes, then now how to fix the below?