Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial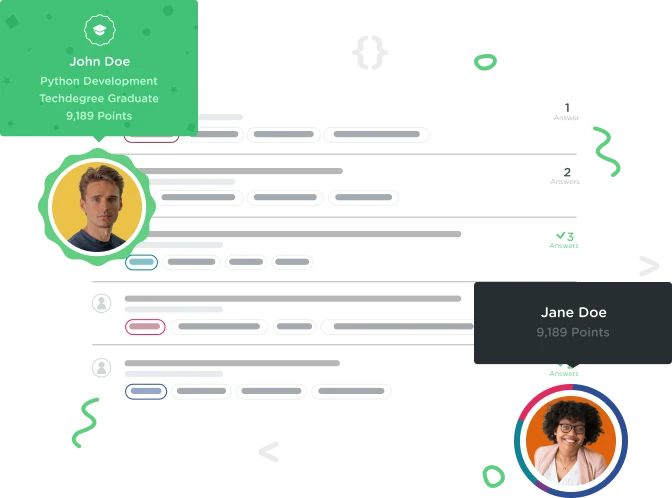
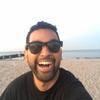
Anthony Albertorio
22,624 PointsSee this question before you start. Get a high level overview of Redux, so that you know why this is worthwhile
First watch this video from the end to see the cool features we can use with Redux. A visual explanation helps a lot. Just notice the cool features. See: https://teamtreehouse.com/library/adding-redux-devtools
Then, skim through these three articles to understand from a very high level what Redux does. No need to worry about understanding it. Just look at the graphs that outline the architecture.
https://www.smashingmagazine.com/2016/06/an-introduction-to-redux/
https://medium.freecodecamp.org/why-redux-makes-sense-to-me-and-how-i-conceptualize-it-c8a3a9db15ca
https://www.sohamkamani.com/blog/2017/03/31/react-redux-connect-explained/
Refer to them as you work through the course. It will help click the logic of why we have all these folders. Just try to understand how files are connected.
Refer to this graph from smashing magazine every time you need to figure out where something is in relation to something else: https://cloud.netlifyusercontent.com/assets/344dbf88-fdf9-42bb-adb4-46f01eedd629/44e53712-498d-400f-8967-8ed0dbdb90a1/new-redux-data-flow-large-opt.png
A quick summary:
React handles your UI. Redux handles your data.
Redux allows you to deal with changes in your data. Data flows one way in a predictable manner. We handle state with two things, actions and reducers.
Actions are the things that change you state. Actions are created by action creators, which are basically are functions that return plain old javascript objects. These objects have two items, the actiontype and the meta data (just key-values) that will be used to update the action.
An action type is basically a string saved as a constant. This is saved in a separate folder. Why? Its just a design decision to help everyone on a team be on the same page with what features exist for each component. Saving the string as a constant allows for errors to pop up and debugging easier.
Reducers are just your methods to change data in your application re-written and placed into a new file. Instead of mutating the data with .setState, we return a new object. Creating a new object for every change allows us to track changes easier. Think of them as git commits for every action. Reducer never mutate data. This is important.
Actions go into reducers and reducers create a new state. That new state is passed to component and the state is updated. Thats the gist of it.
We organize our components into container and presentational components. Container components are the only ones that deal with Redux. Presentational components don't and act just like regular old React.
Each component that has application state changes will have three files. A action file, reducer, and actiontype. Each type has a folder. So player has action/player.js, actiontype/player.js, reducers/player.js. They all have the same name (but located in different files) for organizational purposes.
The Redux store is where you hold your data. Its all your reducers. This is important to know early on. The store is located in your entry file, the index.js of your app.
so action -> reducer (located inside store) -> new state.
There is more, but that is the gist. Don't worry about understanding all of it immediately. It will sink in.
I am leaving lots of stuff out. But just try to get the gist of it. If you have any questions, just ask.
Hope this helps.
1 Answer

mk37
10,271 PointsThank you Anthony, your post helped me a lot
Will Powers
6,612 PointsWill Powers
6,612 PointsThank you for this post, Anthony. As someone who got a bit over their skis using Redux for the first time, this helped me take a step back to get a better understanding of how all the pieces fit together. Cheers! Now on to the course...