Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial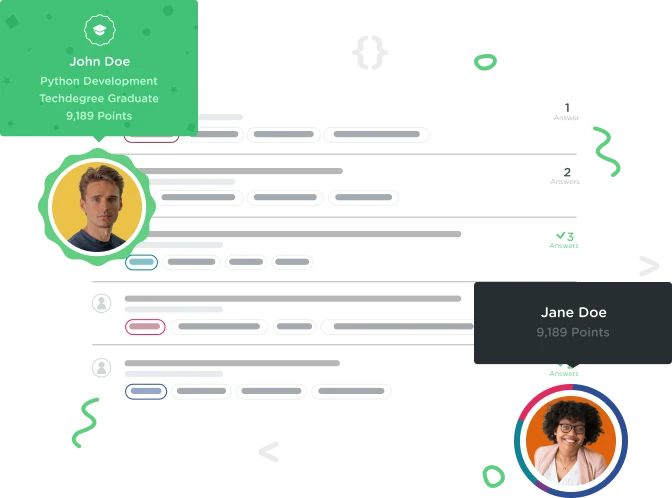
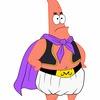
<noob />
17,062 PointsSeeking an explantion about few lines of code on this video
Hi, Overall i understood what craig have done but he introduced a lot of new things, therfore i have few questions:
- what this line of code do:
java return choice.trim().toLowerCase();
2.What the keywords entrySet() and Map.Entry actually doing?, i mean i understand we are looping over the map and name option as our acess point to the map in order to get the key and value of the map
the code:
for(Map.Entry<String, String> option : mMenu.entrySet()
- in this code:
private Map<String,String> mMenu;
we are declaring a Map interface named mMenu and after that we are intialize a new map and assign it to mMenu in the Constructor. code:
mMenu = new HashMap<String, String>();
my question is why we are doing this in this way?, I mean why we first declare a private Interface of map named mMenu and only in the constructor we intialize a new map?
any insights and answer will be appriciated ;D Steve Hunter Eric McKibbin ANTONY MUCHIRI
10 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHi Noob,
Map.Entry is an object type that represents one entry (key-value pair) from the Map.
entrySet() method is from the Map Interface; when called on a Map<Key,Value> object it returns a Set<> Object containing all the keys and values.
Consider this code comparing standard Array Iteration vs Map Iteration:
public static void someMethod (){
//demo String Array Iteration
String [] countries = {"US", "UK", "UAE"};
//looping through countries Array with for-each loop
for (String country: countries){
System.out.println(country);
//String Country is the Object Type returned after each iteration on the countries Array.
}
//demo for Maps
Map<String, String> countryAndCode_Map = new HashMap<>();
countryAndCode_Map.put("US","+1");
countryAndCode_Map.put("UK","+44");
countryAndCode_Map.put("UAE" , "971");
//looping on a Map with for-each loop
for (Map.Entry<String, String> entry: countryAndCode_Map.entrySet()){
System.out.printf("The code for %s is %s%n", entry.getKey(),entry.getValue());
//method countryAndCode_Map.entrySet() returns a Set<> Collection that you can iterate on.
/*Map.Entry<K,V> entry is an object type with Key-Value that is returned with each
iteration through the countryCode_Map*/
}
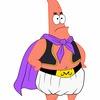
<noob />
17,062 Points
k doshi
128 Points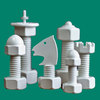
Steven Parker
231,268 Pointsk doshi — Hello. Why'd you tag me on this old question (that already has been answered)?
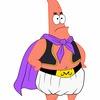
<noob />
17,062 Pointsi didn’t answer ur answer about trim, what exactly trim doing? same for the map.entry and entry.set, why we chose to use this methods?

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello Noob
Trim method returns a copy of the string with the blank spaces on the front and at the back of string eliminated e.g.
String abc = " abc "; String trimmed = abc.trim(); ----->> you will get just "abc" with no spaces before and after the String Characters.

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello Jan Kalasnikov
entrySet() method is from the Map Interface.
When called on a Map<Key,Value> object it returns a Set<> Object containing all the keys and values.
You can then easily iterate on the returned Set<> object using the for-each loop and an object of type Map.Entry<Key, Value> will be returned with each iteration. You can then use the Map.Entry<Key, Value> to get the key and the value for each entry on the Map.
Thanks
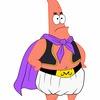
<noob />
17,062 PointsHi, very good explanation thanks antony! however can u explain again what u said here: method countryAndCode_Map.entrySet() returns a Set<> Collection that you can iterate on.
how does the new Set<> is like? can i give an example on how it looks aft we use the entry set command? because as far as i know Sets are like arrays but with benefits such as adding an element remove etc and they contain 1 elements how they know which is the key and which is the value if they are in the same set? i hope u understand my question. thanks in advance!

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello noob developer 👽
A set is an unordered collection of objects in which duplicate values cannot be stored. You are right that Sets are like Arrays but just remember that unlike List and Arrays, Sets do not support indexing or positions of its elements so you cannot access elements by their index . Yes you are also right that you can add and remove stuff from the Set.
Very clever of you to note that Set carries only one item or object type unlike Maps which have 2 objects which may of the same type or different type.
Now the Set obtained from Map.entrySet() method takes one Object of type Map.Entry<Key, Value>
So it is the job of the Map.Entry<Key,Value> object to know which is the key and which is the value. The job of the Set is to just store or carry the Map.Entry<Key,Value> Objects collections inside it.
In-fact I went ahead and printed out the Set obtained from the Map.entrySetMethod() and I got this.
[UK=+44, UAE=971, US=+1]
It is obvious that this printout of raw set is not formatted and a normal person may struggle to understand it. That's why you need to loop through the raw set to do some formatting and represent the data from the set correctly so that it is easy for a normal person to read.
See the code below.
public static void someMethod (){
Map<String, String> countryAndCode_Map = new HashMap<>();
countryAndCode_Map.put("US","+1");
countryAndCode_Map.put("UK","+44");
countryAndCode_Map.put("UAE" , "971");
//looping on a Map with for-each loop
Set<Map.Entry<String, String >> set = countryAndCode_Map.entrySet();
System.out.print(set);
//on printing out you will get this without looping
//>>>>>[UK=+44, UAE=971, US=+1]
}
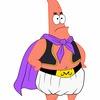
<noob />
17,062 PointsSo, basically i should use Map.entry and entry.Set when i want to iterate thorugh the key and the value and print them toghter in a situations like i want to index something as craig did? i just want to get a clarification : the Set<> the is returned by the Map.entrySet is actually contains a <Key, Value> in each of his indexes? and that is why we can use the getKey() and the getValue() methods in order to print them?
if u can confrim that i understand it correctly it will be good.
Tonnie i really appreiciate ur time for answering my questions. Tonnie Fanadez

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsThanks for your good comments, you're welcome.
Yeah it is the best practice to use Map.Entry<K,V> object and Map.entrySet() so that you can get a chance to format the output properly.
Indices don't apply on a Set because Sets don't have the get() method. To get something out of a set you have to loop through the Set and print every element on the Set. To get an Index from a Set you will have to convert the Set to a List, and then use get()method of list.
List<String> list = new ArrayList<String>(set);
The Set obtained from Map.entrySet() method contain objects of Map.Entry<Key, Value> class. You can then use the Map.Entry<Key, Value> to get the key and the value using getKey() and the getValue() methods in order to print them?
Craig uses the Set to Store HashTags and Mentions so that there is no repetition or duplication for a HashTag or a Mention.
Hope this clarifies.
Thanks,
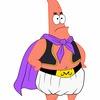
<noob />
17,062 Pointshow excatly the objects looks in the set that is the last thing im trying to figure out. the Set<> containing for example this objects like u did? :
[UK=+44, UAE=971, US=+1]

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsIf you really want to see how a Set looks likes for different Generic Objects I suggest you open Jshell and try out this code for example with a Set<String>. jshell> Set<String> set = new HashSet<>(); set ==> []
jshell> set.add("me"); $2 ==> true
jshell> set.add("you"); $3 ==> true
jshell> set.add("them"); $4 ==> true
jshell> set.add("her"); $5 ==> true
jshell> set.add("him"); $6 ==> true
jshell> set.add("it"); $7 ==> true
jshell> set set ==> [her, me, them, it, him, you]
It prints out String Objects without any order separated by a comma and enclosed within Square Brackets []. So it is basically the same code as this - [UK=+44, UAE=971, US=+1]
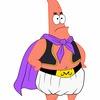
<noob />
17,062 Pointsso when i use the getKey and getValue methods java knows to get the key and the value even if they separated?

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointsnoob developer Yes but you need to use a combination of Set and Map.Entry Object and then do a loop, Set alone won't help as it will print everything in a raw format.
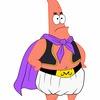
<noob />
17,062 PointsThanks tonnie i finally understand it!

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointsnoob developer I'm glad you did, will see you around.
Ikuyasu Usui
36 PointsIkuyasu Usui
36 PointsGiven mMenu is a Map, mMenu.entrySet gives you things in the Map for the data type(interface) for each entry as Map.Entry<key, value> where key and value obviously are corresponding key and value in the Map. Now, Map.Entry<key, value> is some sort of Map like interface, and option is an object of this data type. It has and used in the video two methods, getKey() and .getValue(): This is an link.
Map.Entry looks a bit unfamiliar. We know Map is an interface, and interface has a method? It turns out that Entry is a nested class or nested interface in Map. So, in the code for Map, we should be able to find something like: